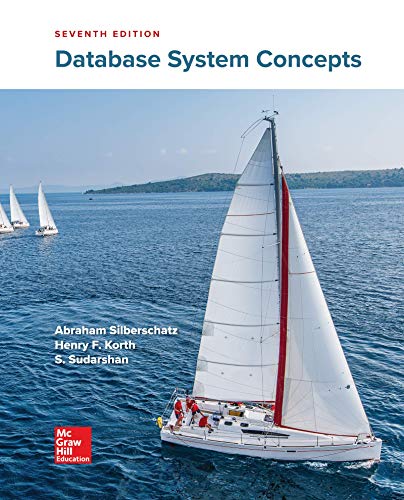
Write a program that reads words passing from a string parameter and create a dictionary mapping last letters to the number of words ending with that letter.
For example, the string is 'apple banana orange grape cherry', the program will out put a dictionary fruitName that
print(fruitName)
will give us output
{'e': 3, 'a': 1, 'y': 1}
def count_last_letter(words):
# YOUR CODE HERE
raise NotImplementedError()

In the given scenario, we need to write a function count_last_letter(words) which takes a string as parameter and returns a dictionary with the last letters of the words present in the dictionary and their count of the number of such last lettered words present in the string.
For this we will make use of a function named split() in python which will separate the words in the string by a white space.
Step by stepSolved in 4 steps with 1 images

- Complete the doctring.def average_daily_temp(high_temps: List[int], low_temps: List[int]) -> List[float]: """high_temps and low_temps are daily high and low temperatures for a series of days. Return a new list of temperatures where each item is the daily average. Precondition: len(high_temps) == len(low_temps) >>> average_daily_temp([26, 27, 27, 28, 27, 26], [20, 20, 20, 20, 21, 21]) [23.0, 23.5, 23.5, 24.0, 24.0, 23.5] """arrow_forwardImplement the following: Given: names = ['ann', 'bill', 'calvin', 'david']ids = [12, 34, 56, 78] 1) Create a dictionary, stuInfo by using the two given lists above.2) Use a loop to print all keys and values in the dictionary stulfno. 3) Print the dictionary, stuInfo. Example Output12 ANN34 BILL 56 CALVIN78 DAVID{'ann': 12, 'bill': 34, 'calvin': 56, 'david': 78} NEED HELP WITH PYTHONarrow_forwardQ1. The last post didnt include the items in the database. Need a different function for the python program display_ticket below: def display_tickets():t = Texttable()tickets=cur.execute('''SELECT * FROM tickets''')for i in tickets:t.add_rows([['tid', 'actual_speed','posted_speed','age','violator_sex'],list(i)])print()print(t.draw())print() Q2. Also need a different function for the python program add_ticket below: def add_tickets():actual_speed=int(input("enter actual speed:"))posted_speed=int(input("enter posted speed:"))age=int(input("enter age:"))sex=input("Male or Female:")cur.execute('''INSERT INTO tickets(actual_speed,posted_speed,age,violator_sex) VALUES(?,?,?,?)''',(actual_speed,posted_speed,age,sex))con.commit() If you'd like to see what thousands of traffic tickets look like, check out this link: https://www.twincities.com/2017/08/11/we-analyzed-224915-minnesota-speeding-tickets-see-what-we-learned/arrow_forward
- How can I read from a file a string version of a nested dictionary and store as a dictionary? For example, I have the following nested dictionary: {1: {'Name': 'Bob', 'Pass': 'pass123', 'Sports': ['Hockey', 'Football'], 'Activities': ['eating', 'sleeping']} ; 2: {'Name': 'Kayla', 'Pass': 'cyansus', 'Sports': [], 'Activities': []}} To write this to a file I needed to convert to a string (and it is fine writing to a file). But I am having trouble reading this from the file and creating a nested dictionary from it. I can read the string in fine but creating the nested dictionary I am lost at. Any help will be appreciated. Thank youarrow_forwardInstructions Write a program to test various operations of the class doublyLinkedList. Your program should accept a list of integers from a user and use the doubleLinkedList class to output the following: The list in ascending order. The list in descending order. The list after deleting a number. A message indicating if a number is contained in the list. Output of the list after using the copy constructor. Output of the list after using the assignment operator. An example of the program is shown below: Enter a list of positive integers ending with -999: 83 121 98 23 57 33 -999 List in ascending order: 23 33 57 83 98 121 List in descending order: 121 98 83 57 33 23 Enter item to be deleted: 57 List after deleting 57 : 23 33 83 98 121 Enter item to be searched: 23 23 found in the list. ********Testing copy constructor*********** intList: 23 33 83 98 121 ********Testing assignment operator*********** temp: 23 33 83 98 121 Your program should use the value -999 to denote the end of the…arrow_forwardWrite a python program that reads the contents of a text file. The program should create a dictionary in which the keys are the individual words found in the file and the values are the number of times each word appears. For example, if the word "the" appears 128 times, the dictionary would contain an element with 'the' as the key and 128 as the value. The program should either display the frequency of each word or create a second file containing a list of each word and it's frequency. This program has really been giving me trouble. Any help is great appreciated. Thanks so much!arrow_forward
- In Python languagearrow_forwardDesign a class that acquires the JSON string from question #1 and converts it to a class data member dictionary. Your class produces data sorted by key or value but not both. Provide searching by key capabilities to your class. Provide string functionality to convert the dictionary back into a JSON string. question #1: import requestsfrom bs4 import BeautifulSoupimport json class WebScraping: def __init__(self,url): self.url = url self.response = requests.get(self.url) self.soup = BeautifulSoup(self.response.text, 'html.parser') def extract_data(self): data = [] lines = self.response.text.splitlines()[57:] # Skip the first 57 lines for line in lines: if line.startswith('#'): # Skip comment lines continue values = line.split() row = { 'year': int(values[0]), 'month': int(values[1]), 'decimal_date': float(values[2]),…arrow_forwardjava Suppose namesis an ABList<String>containing 10elements. The call names.add(0, "George") results in: A. an exception being thrown. B. a 10-element list with "George" as the first element. C. an 11-element list with "George" as the first element. D. a single element list containing "George". E. None of these is correct.arrow_forward
- In python: student_dict is a dictionary with students' name and pedometer reading pairs. A new student is read from input and added into student_dict. For each student in student_dict, output the student's name, followed by "'s pedometer reading: ", and the student's pedometer reading. Then, assign average_value with the average of all the pedometer readings in student_dict..arrow_forwardWrite a python program that stores current grades in a dictionary, with course codes as keys and percent grades as values. Start with an empty dictionary and then use a while loop to enable input of course codes and percent grades from the keyboard. Enter data for at least five courses. After entering the data, use a for loop and the keys to show the current status of all courses. This same loop should include code that enables determination of the worst course and the average of all courses. Both of these findings should be printed when the loop ends. The worst course should be dropped and reported. This being done, the program should use another loop and the items method to display the revised courses and grades and report the revised term average.arrow_forwardComplete the method “readdata”. In this method you are to read in at least 10 sets of student data into a linked list. The data to read in is: student id number, name, major (CIS or Math) and student GPA. You will enter data of your choice. You will need a loop to continue entering data until the user wishes to stop. Complete the method “printdata”. In this method, you are to print all of the data that was entered into the linked list in the method readdata. Complete the method “printstats”. In this method, you are to search the linked list and print the following: List of student’s id and names who are CIS majors List of student’s id and names who are Math majors List of student’s names along with their gpa who are honor students (gpa 3.5 or greater) All information for the CIS student with the highest gpa (you may assume that different gpa values have been entered for all students) CODE (student_list.java) You MUST use this code: package student_list;import…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
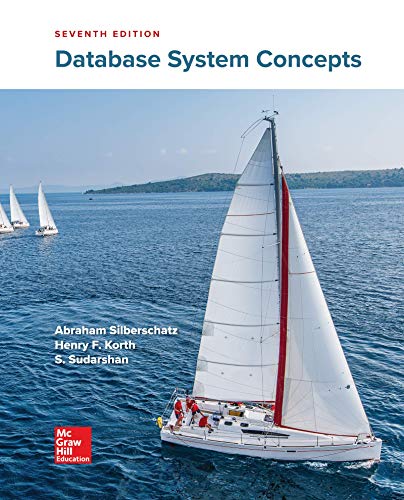
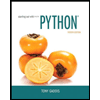
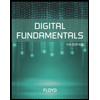
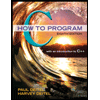
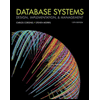
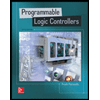