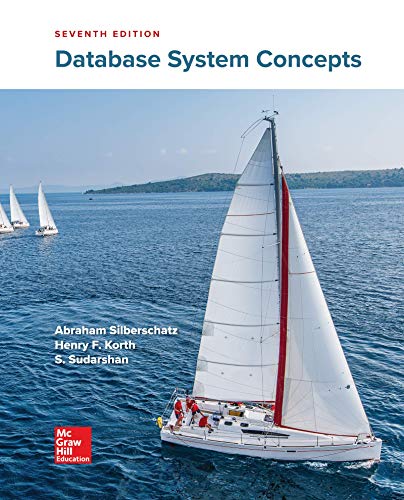
Concept explainers
The program first reads integer cityCount from input, representing the number of pairs of inputs to be read. Each pair has a string and a character. One City object is created for each pair and added to
Ex: If the input is:
4 Sundance N Adomstown N Hum Y Hanna Y
then the output is:
City: Sundance, Tour: N City: Adomstown, Tour: N
Note: The vector has at least one element.
#include <iostream>
#include <vector>
using namespace std;
class City {
public:
void SetNameAndTour(string newName, char newTour);
char GetTour() const;
void Print() const;
private:
string name;
char tour;
};
void City::SetNameAndTour(string newName, char newTour) {
name = newName;
tour = newTour;
}
char City::GetTour() const {
return tour;
}
void City::Print() const {
cout << "City: " << name << ", Tour: " << tour << endl;
}
class SmallTowns {
public:
void InputCities();
void PrintSelectedCities();
private:
vector<City> cityList;
};
void SmallTowns::InputCities() {
int cityCount;
unsigned int i;
City currCity;
string currName;
char currTour;
cin >> cityCount;
for (i = 0; i < cityCount; ++i) {
cin >> currName;
cin >> currTour;
currCity.SetNameAndTour(currName, currTour);
cityList.push_back(currCity);
}
}
/* Your code goes here */
int main() {
SmallTowns smallTowns;
smallTowns.InputCities();
smallTowns.PrintSelectedCities();
return 0;
}
c++ and please please make it right

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

- convert this code to JAVA location = [] size = [] rover = 0 def displayInitialList(location, size): global rover print("FSB# location Size") for i in range(len(location)): print(i," ",location[i]," ",size[i]) if rover<len(size)-1: print("Rover is at ",location[rover+1]) else: print("Rover is at ",location[rover]) def allocateMemory(location,size,blockSize): global rover if rover<len(size): while size[rover]<blockSize: rover+=1 if i==len(size): return False location[rover] += blockSize size[rover] -= blockSize rover+=1 return True else: return False def deallocateMemory(location,size,delLocation,delSize): i=0 while delLocation>location[i]: i+=1 location[i]-=delSize size[i]+=delSize while True: print("1. Define Initital memory\n2. Display initial FSB list\n3. Allocate memory\n4. Deallocate memory\n5. Exit") print("Enter choice: ",end="") choice = int(input()) if…arrow_forwardFor any element in keysList with a value greater than 50, print the corresponding value in itemsList, followed by a comma (no spaces). Ex: If the input is: 32 105 101 35 10 20 30 40 the output is: 20,30, 1 #include 2 3 int main(void) { const int SIZE_LIST = 4; int keysList[SIZE_LIST]; int itemsList[SIZE_LIST]; int i; 4 6 7 8 scanf("%d", &keysList[0]); scanf ("%d", &keysList[1]); scanf("%d", &keysList[2]); scanf("%d", &keysList[3]); 10 11 12 13 scanf ("%d", &itemsList[0]); scanf ("%d", &itemsList[1]); scanf("%d", &itemsList[2]); scanf ("%d", &itemsList[3]); 14 15 16 17 18 19 /* Your code goes here */ 20 21 printf("\n"); 22 23 return 0; 24 }arrow_forwardReadInstructor. The class will: – Create an array of instructor objects – Read the instructors.txt file – Create an Instructor object for each line of input and store the object in the array. – After closing the file, it will print out a line for each instructor in the array. Input file:Mary Smith 1991 50000.00 John Jones 2000 90000.00 Terry Seidel 1992 51000.00 Jessica Terrell 2000 91000.00arrow_forward
- In Java.arrow_forwardIf possible, mention software development metrics. Everything needs extreme care.arrow_forwardA method named getUserStringsAndPopulate Array(). This method should keep prompting the user to enter as many strings as the user wants. The user should be able to stop entering strings by entering EXIT. This method should take the strings entered by the user and populate a one-dimensional array of strings with the user input. The string EXIT should NOT be added to the array because it is a special flag that will tell the method to stop prompting the user to enter more strings. This method should return the one-dimensional array of strings populated with user input and should not accept any arguments. 2.b) A method named searchArray() that would accept a one-dimensional array of strings and search the array for the string Winter. The search logic should NOT be case sensitive. If a match is found, this method should print the string in the array followed by a message like " --- Found a match!". Otherwise, the method should not print anything. This method should NOT return anything.…arrow_forward
- The Lo Shu Magic Square is a grid with 3 rows and 3 columns shown below. The Lo Shu Magic Square has the following properties: The grid contains the numbers 1 – 9 exactly The sum of each row, each column and each diagonal all add up to the same number. This is shown below: Write a program that simulates a magic square using 3 one dimensional parallel arrays of integer type. Do not use two-dimensional array. Each one the arrays corresponds to a row of the magic square. The program asks the user to enter the values of the magic square row by row and informs the user if the grid is a magic square or not. Processing Requirements - c++ Use the following template to start your project: #include<iostream> using namespace std; // Global constants const int ROWS = 3; // The number of rows in the array const int COLS = 3; // The number of columns in the array const int MIN = 1; // The value of the smallest number const int MAX = 9; // The value of the largest number //…arrow_forwardvoid getVectorSize(int& size); void readData(vector<Highscore>& scores); void sortData(vector<Highscore>& scores); vector<Highscore>::iterator findLocationOfLargest( const vector<Highscore>::iterator startingLocation, const vector<Highscore>::iterator endingLocation); void displayData(const vector<Highscore>& scores); The size parameter from the given code won't be needed now, since a vector knows its own size. Notice that the findLocationOfLargest() function does not need the vector itself as a parameter, since you can access the vector using the provided iterator parameters. The name field in the struct must still be a c-string The focus of this assignment is to use iterators. You must use iterators wherever possible to access the vector. As a result, you must not use square brackets, the push_back() function, the at() function, etc. Also, the word "index" shouldn't appear in your code anywhere. You won't get full credit if…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
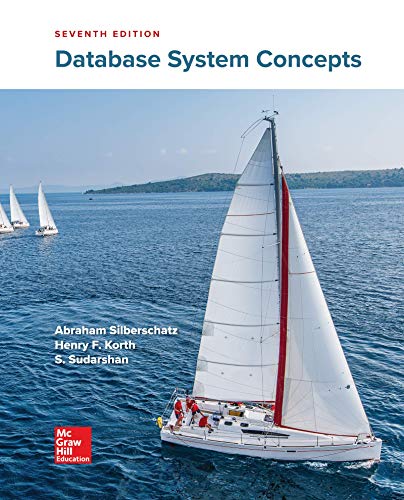
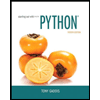
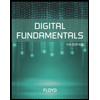
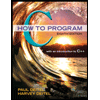
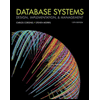
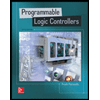