Write a program that reads a list of integers, and outputs whether the list contains all even numbers, odd numbers, or neither. The input begins with an integer indicating the number of integers that follow.
Write a

Programming language is missing in the question. So we will answer this program in Python. If you want this program to be executed in some other programming language, kindly repost the question with a specific programming language.
#Function to check whether the list contains all even numbers
def is_list_even(my_list):
#Iterate through list elements
for x in my_list:
#Check if the element is odd
if x % 2 != 0:
return False
return True
#Function to check whether the list contains all odd numbers
def is_list_odd(my_list):
#Iterate through list elements
for x in my_list:
#Check if the element is even
if x % 2 == 0:
return False
return True
#Create an empty list
my_list = []
#Take number of elements as input
n = int(input())
#Iterate till the range
for i in range(0,n):
#Fill the list
my_list.append(int(input()))
#Check whether the list contains all even numbers
if is_list_even(my_list) == True and is_list_odd(my_list) == False:
#Print statement
print("all even")
#Check whether the list contains all odd numbers
elif is_list_even(my_list) == False and is_list_odd(my_list) == True:
#Print statement
print("all odd")
#Otherwise the list contains neither all even nor all odd numbers
else:
#Print statement
print("not even or odd")
Step by step
Solved in 4 steps with 5 images

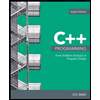
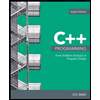