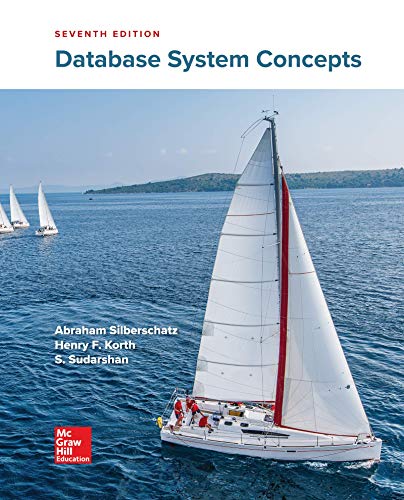
(python) Write a program that first gets a list of integers from input. The input begins with an integer indicating the number of integers that follows. Then, get the last value from the input, and output all integers less than or equal to that value.
Ex: If the input is:
5the output is:
50The 5 indicates that there are five integers in the list, namely 50, 60, 140, 200, and 75. The 100 indicates that the program should output all integers less than or equal to 100, so the program outputs 50, 60, and 75.
Ex. if the input is:
7
25
32
27
28
27
25
31
28
the output is:
25
27
28
27
25
Such functionality is common on sites like Amazon, where a user can filter results. Utilizing functions will help to make your main very clean and intuitive.
Your code must define and call the following two functions:
def get_user_values()
def ints_less_than_or_equal_to_threshold(user_values, upper_threshold)
Note: ints_less_than_or_equal_to_threshold() returns the new array.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps

- Code following instructions using pyhton: 1. Write a function that accepts a list as an argument (assume the list contains integers) and returns the total of the values in the list 2.Draw a flowchart showing the general logic for totaling the values in a list. 3.Assume the names variable references a list of strings. Write code that determines whether 'Ruby' is in the names list. If it is, display the message 'Hello Ruby'. Otherwise, display the message 'No Ruby'.arrow_forward1 - Write a Python function that will do the following: 1. Ask the user to input an integer that will be appended to a list that was originally empty. This will be done 5 times, meaning that when the input is complete, the list will have five elements (all integers). a. Determine whether each element is an even number or an odd number b. Return a list of five string elements "odd" and "even" that map the indexes of the elements of the input list, according to whether these elements are even or odd numbers.For example: if the input sequence is 12, 13, 21, 51, and 30, the function will return the list ["even", "odd", "odd", "odd", "even"].arrow_forwardPlease send me answer of this question immediately and i will give you like sure sirarrow_forward
- python: def character_gryffindor(character_list):"""Question 1You are given a list of characters in Harry Potter.Imagine you are Minerva McGonagall, and you need to pick the students from yourown house, which is Gryffindor, from the list.To do so ...- THIS MUST BE DONE IN ONE LINE- First, remove the duplicate names in the list provided- Then, remove the students that are not in Gryffindor- Finally, sort the list of students by their first name- Don't forget to return the resulting list of names!Args:character_list (list)Returns:list>>> character_gryffindor(["Scorpius Malfoy, Slytherin", "Harry Potter,Gryffindor", "Cedric Diggory, Hufflepuff", "Ronald Weasley, Gryffindor", "LunaLovegood, Ravenclaw"])['Harry Potter, Gryffindor', 'Ronald Weasley, Gryffindor']>>> character_gryffindor(["Hermione Granger, Gryffindor", "Hermione Granger,Gryffindor", "Cedric Diggory, Hufflepuff", "Sirius Black, Gryffindor", "JamesPotter, Gryffindor"])['Hermione Granger, Gryffindor', 'James…arrow_forwardplease solve this question and the result output should EXACTLY match as givenarrow_forwardIn python, Problem Description:You are hosting a party and do not have room to invite all of your friends. You use the following unemotional mathematical method to determine which friends to invite. Number your friends 1, 2, . . . , K and place them in a list in this order. Then perform m rounds. In each round, use a number to determine which friends to remove from the ordered list. The rounds will use numbers r1, r2, . . . , rm. In round i remove all the remaining people in positions that are multiples of ri (that is, ri, 2ri, 3ri, . . .) The beginning of the list is position 1. Output the numbers of the friends that remain after this removal process. Input Specification:The first line of input contains the integer K (1 ≤ K ≤ 100). The second line of input contains the integer m (1 ≤ m ≤ 10), which is the number of rounds of removal. The next m lines each contain one integer. The ith of these lines (1 ≤ i ≤ m) contains ri ( 2 ≤ ri ≤ 100) indicating that every person at a position…arrow_forward
- python LAB: Subtracting list elements from max When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. Write a program that adjusts a list of values by subtracting each value from the maximum value in the list. The input begins with an integer indicating the number of integers that follow.arrow_forwardIN PYTHON! finish the incomplete program by writing the code that can be placed below the #write the code. use loops to calculate the sums and avg of each row of scores in the list. the following output should be displayed. dont change any part of the code. OUTPUT: Average scores for students: Student # 1: 12.0 Student # 2: 22.0 CODE: students =[ [11, 12, 13], [21, 22, 23] ] avgs = [] # Write your code here: print('Average scores for students: ") for i, avg in enumerate (avgs): print("Student #', i + 1, ':', avg)arrow_forwardInteger num_reading is read from input, representing the number of integers to be read next. Read the remaining integers from input and insert each integer at the front of reading_list at position 0.arrow_forward
- For each of the following write a list comprehension: a. Create a list of the first 10 multiples of 13 (from 13 to 130) b. Create a list of the squares of the first 100 integers, but only with those that are odd.arrow_forwardPlease help me with this in python. I have attached question and output below.arrow_forwardI really need help with this problem; (1) Prompt the user to enter four numbers, each corresponding to a person's weight in pounds. Store all weights in a list. Output the list. (2 pts) Ex: Enter weight 1: 236.0 Enter weight 2: 89.5 Enter weight 3: 176.0 Enter weight 4: 166.3 Weights: [236.0, 89.5, 176.0, 166.3] (2) Output the average of the list's elements with two digits after the decimal point. Hint: Use a conversion specifier to output with a certain number of digits after the decimal point. (1 pt) (3) Output the max list element with two digits after the decimal point. (1 pt) Ex: Enter weight 1: 236.0 Enter weight 2: 89.5 Enter weight 3: 176.0 Enter weight 4: 166.3 Weights: [236.0, 89.5, 176.0, 166.3] Average weight: 166.95 Max weight: 236.00 (4) Prompt the user for a number between 1 and 4. Output the weight at the user specified location and the corresponding value in kilograms. 1 kilogram is equal to 2.2 pounds. (3 pts) Ex: Enter a list location (1 - 4): 3 Weight in pounds:…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
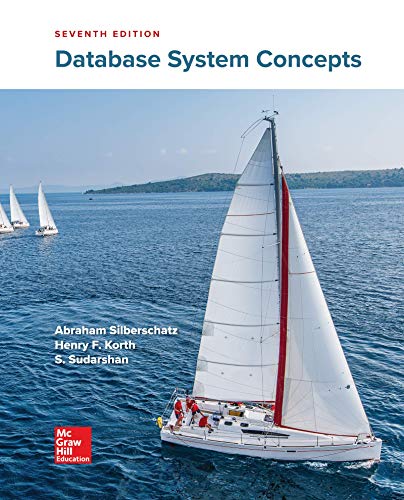
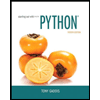
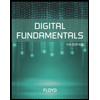
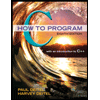
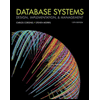
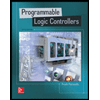