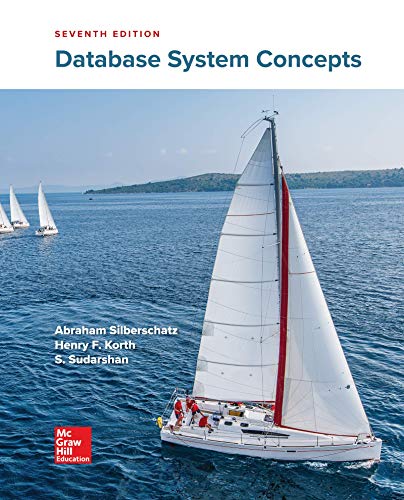
Concept explainers
Write a
You can choose the actual values to use for your data. Make sure to do enough sets of data to well test your program! (Empty data set, normal data set, data with large values, data with short values, etc.)
Also, your program can't know ahead of time how many people are enrolled in the class...
Don't forget to read the file's name from the user and protect your program against any errors that may occur during the opening of the file.
Try to use functions to break up the program into more manageable pieces.
USING C++
As an example, you might have the data file contain:

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 4 images

- In Java: When analyzing data sets, such as data for human heights or for human weights, a common step is to adjust the data. This can be done by normalizing to values between 0 and 1, or throwing away outliers. For this program, adjust the values by subtracting the smallest value from all the values. The input begins with an integer indicating the number of integers that follow. Assume that the list will always contain less than 20 integers. Ex: If the input is: 5 30 50 10 70 65 the output is: 20 40 0 60 55 For coding simplicity, follow every output value by a space, even the last one. Your program must define and call a method:public static int getMinimumInt(int[] listInts, int listSize) import java.util.Scanner; public class LabProgram {/* Define your method here */ public static void main(String[] args) {/* Type your code here. */}}arrow_forwardIn Python Initially, you will prompt the user for the names of two files: one will contain Customer information, and the other will contain Product information, as described below. Sample files are attached (below) to this project so you can download and review them. Some test cases may use other files, which you will not be able to see, so it is important that your program ask the user for the file names, and be able to open and read any file. The Customer Information file will be a csv file, with no header row, in which each line has the following fields, which are separated by commas. Customer_Number Customer_Name Customer_Balance Customer_Password For example, the sample file named customers.csv has several lines, and the first two are: 24155,"Carey, Drew Allison",838.41,Bo7&J 24426,"Butler, Geoffrey Barbara",722.93,Ep5& The Product Information file will be a csv file, with no header row, in which each line has the following fields, which are separated by commas:…arrow_forwardUse C++. This lab will help you practice simple data processing from a file where you don't know the amount of information in the file ahead of time. (It also provides some refresher in basic statistics.) (classes may or may not be of use here...you decide!) Write a program that calculates basic statistics for a set of numbers stored in a file. Make sure to allow the user to tell you the name of their file and to check for file open errors. The data in the file will be a space-separated list of numbers. You won't know the length of the list ahead of time. You'll need to calculate the average and standard deviation of the list as well as determining the maximum and minimum for the data. To refresh your memory, the standard deviation is the square root of the variance. And the variance? Well, it is found as discussed in this brief paper. You can choose the actual values to use for the data. Make sure to do enough different sets of data to test your program well! Remember that your…arrow_forward
- 1. The codewords will be scrambled words. You will read the words used for the codes from a file and store it into an array. There are 60 words in the file that range in size from 3 characters to 7 characters. The file is called wordlist.txt and can be found attached to the assignment in IvyLearn.2. Start with input for an integer seed for the random number generator. There is no need to put a prompt before the cin operation.3. Ask the Player if they are ready to play and only proceed if they type a Y or y. If the user types an N or n then the program should finish. a. INPUT VALIDATION: Make sure the user has typed either y, Y, n, or N4. Create a variable to keep track of the number of guesses the user has made.5. Use the random number generator to pick a word from the list of words.6. Once you have chosen a word as the codeword you will need to scramble the letters to make it into a code. You should use the random number generator to help you mix up the letters.7. Display to the user…arrow_forwardWrite a program in C++ that prints a sorted phone list from a database of names and phone numbers. The data is contained in two files named "phoneNames.txt" and "phoneNums.txt". The files may contain up to 2000 names and phone numbers. The files have one name or one phone number per line. To save paper, only print the first 50 lines of the output. Note: The first phone number in the phone number file corresponds to the first name in the name file. The second phone number in the phone number file corresponds to the second name in the name file. etc. You will find the test files in the Asn Five.ziparrow_forwardFor this assignment, you will write a Python program that uses a file named scores.txt to store sets of bowling scores for different dates. scores.txt should store the data so that each line has a month, day, and the scores the user earned on that date. As an example, scores.txt might look something like this: January 15 200 300 126 200 250April 20 125 100 May 17 300 100 215 The very first time your program starts, scores.txt should not exist (i.e. create it with Python code the first time the program runs). In your main function, continuously give the user the following five options: Quit the program. View all Scores. If the user selects this option, call a function named view_scores. This function should print the scores in a nicely formatted manner. For example: "On January 15, you scored 200, 300, and 126", etc.. Add a Score. If the user selects this option, call a function named add_score. This function should ask the user for a month, day, and as many scores as they want to…arrow_forward
- Try to create a dictionary that has a listing of people and includes one interesting fact about each of them. Display each person and their interesting fact on the screen. From there, alter a fact about one of the people on the list. Also, add an extra person and corresponding fact to the list. Display the newly created list of people and facts. Try running the program multiple times and take note of whether the order changes. Sample output: Jeff: Was born in France. David: Was a mascot in college. Anna: Has arachnophobia. Dylan: Has a pet hedgehog. Jeff: Was born in France. David: Can juggle. Anna: Has arachnophobia. Code Listing 209 106arrow_forwardJava Your program must read a file called personin.txt. Each line of the file will be a person's name, the time they arrived at the professor's office, and the amount of time they want to meet with the professor. These entries will be sorted by the time the person arrived. Your program must then print out a schedule for the day, printing each person's arrival, and printing when each person goes in to meet with the professor. You need to print the events in order of the time they happen. In other words, your output will be sorted by the arrival times and the times the person goes into the professor's office. In your output you need to print out a schedule. In the schedule, new students go to the end of the line. Whenever the professor is free, the professor will either meet with the first person in line, or meet with the first person in line if nobody is waiting. Assume no two people arrive at the same time. You should solve this problem using a stack and a queue. You can only…arrow_forwardI know you are using Al tools to generate answer but I don't want these answers. If I see Al or plagiarism in my response I'll definitely reduce rating and will report for sure. So be careful. I'll take serious action. Scenario involving a list of books stored in a file named "Books.txt." Create a Python program that performs the following tasks: Output all books in your list. Output books published between the years 2015 and 2020. Calculate the average rating of all books. Create a histogram of the number of books in each genre. Each element in the histogram should represent the count of books in a specific genre. Take all the books in "Books.txt" and store them in a Python set. Output all unique authors of the books. Output the total number of entries in your set. Books.txt: "The Silent Observer," 2018, Mystery, Emily Williams "Beyond the Horizon," 2016, Fantasy, David Thompson "Code Breakers," 2019, Thriller, Sarah Miller "Infinite Realms," 2017, Sci-Fi, Mark Anderson "Whispers of…arrow_forward
- You are going to write a program (In Python) called BankApp to simulate a banking application.The information needed for this project are stored in a text file. Those are:usernames, passwords, and balances.Your program should read username, passwords, and balances for each customer, andstore them into three lists.userName (string), passWord(string), balances(float)The txt file with information is provided as UserInformtion.txtExample: This will demonstrate if file only contains information of 3 customers. Youcould add more users into the file.userName passWord Balance========================Mike sorat1237# 350Jane para432@4 400Steve asora8731% 500 When a user runs your program, it should ask for the username and passwordfirst. Check if the user matches a customer in the bank with the informationprovided. Remember username and password should be case sensitive.After asking for the user name, and password display a menu with the…arrow_forwardSplit blood pressure into systolic and diastolic blood pressure with integer as data type readings and remove the original column. Examine the data and look for predictor columns that are indicator variables, 0 or 1. Convert the data type to Boolean. Using code prepare lists of predictor column names for each data type. Make sure all the predictor columns are included in one and only one list. Print the length of the list of each data type and the sum of the lengths and verify that all predictor variables are accounted for in the lists. Use f-string to align the numbers properly.arrow_forwardPlease write a program that checks if the last data item entered appears earlier in the list. The input is a sequence of non-negative numbers, which is terminated by a user entering a -1. The code needs to output ONLY ONE of these 3 messages: Yes the last data item appears earlier, No the last data item appears earlier, No Data Provided The code is also not allowed to compare the last number with itself. Thanks!arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
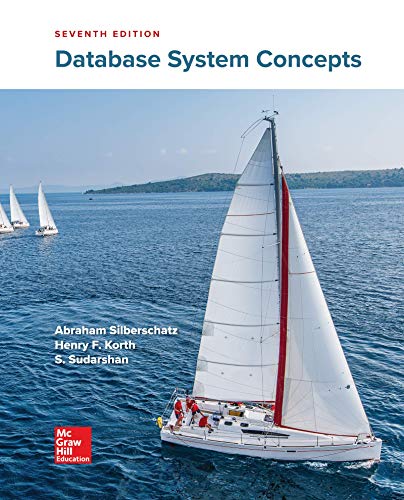
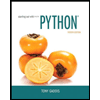
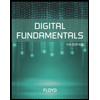
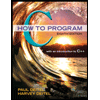
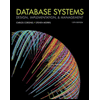
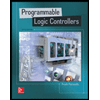