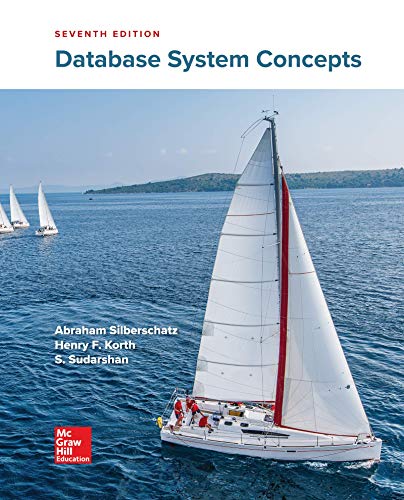
Concept explainers
I am making a program with a CSV file with three columns: names, cities, and heights. In each city, I found the average height. I need to find the two people closest to the average. Most of the time, it works, but there is an error. A person second closest to the average doesn't even appear on the list. Also, the value for their height is way too high. What changes do I need to make?
import csv
data = []
with open("C:\\Users\\lucas\\Downloads\\sample-data.csv") as f:
reader = csv.reader(f)
for row in csv.reader(f):
data.append(list(row))
f.close()
data.pop(0)
city_list = []
for i in range(len(data)):
city_list.append(data[i][1])
city_list.sort()
unique_cities = []
people_count = []
for city in city_list:
# this if statement analyzes which cities are in unique_cities. If they are not in the list,
# then they will be appended into the list
if city not in unique_cities:
unique_cities.append(city)
for city in unique_cities:
count = city_list.count(city)
people_count.append(count)
for k in range(len(unique_cities)):
print(f'City: {unique_cities[k]}, Population: {people_count[k]}')
# because unique cities lists one of each city,
# and because we want to count the frequency of the cities, we use city_list
for city in unique_cities:
name_list = []
height_list = []
for d in data:
if city == d[1]:
height_list.append(float(d[2]))
name_list.append(d[0])
avg = sum(height_list) / len(height_list)
difference_list = []
for height in height_list:
diff = abs(height - avg)
difference_list.append(diff)
closest_to_average_height = 1000000000
closest_to_average_name = " "
second_closest_height = 1000000000
second_closest_name = " "
difference_list_two = difference_list.copy()
closest_to_average_diff = difference_list_two[0]
# second_closest_to_average_diff = closest_to_average_diff
for index in range(len(height_list)):
current_diff = difference_list_two[index]
if current_diff <= closest_to_average_diff:
second_closest_to_average_diff = closest_to_average_diff
second_closest_to_average_height = closest_to_average_height
second_closest_to_average_name = closest_to_average_name
closest_to_average_diff = current_diff
closest_to_average_height = height_list[index]
closest_to_average_name = name_list[index]
print(f"City: {city} Most height: {closest_to_average_name}, Height: {closest_to_average_height}, "
f"Second most height: {second_closest_to_average_name}, Height: {second_closest_to_average_height} ")

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

How could this program work...
with lists instead of dictionaries
without the functions lambda or key=
example:
sorted_order=dict(sorted(person_diff.items(), key=lambda item: item[1]))
How could this program work...
with lists instead of dictionaries
without the functions lambda or key=
example:
sorted_order=dict(sorted(person_diff.items(), key=lambda item: item[1]))
- Write a program that reads movie data from a CSV (comma separated values) file and output the data in a formatted table. The program first reads the name of the CSV file from the user. The program then reads the CSV file and outputs the contents according to the following requirements: Each row contains the title, rating, and all showtimes of a unique movie. A space is placed before and after each vertical separator ('|') in each row. Column 1 displays the movie titles and is left justified with a minimum of 44 characters. If the movie title has more than 44 characters, output the first 44 characters only. Column 2 displays the movie ratings and is right justified with a minimum of 5 characters. Column 3 displays all the showtimes of the same movie, separated by a space. Each row of the CSV file contains the showtime, title, and rating of a movie. Assume data of the same movie are grouped in consecutive rows. Hints: Use the find() function to find the index of a comma in each row of…arrow_forwardTry to create a dictionary that has a listing of people and includes one interesting fact about each of them. Display each person and their interesting fact on the screen. From there, alter a fact about one of the people on the list. Also, add an extra person and corresponding fact to the list. Display the newly created list of people and facts. Try running the program multiple times and take note of whether the order changes. Sample output: Jeff: Was born in France. David: Was a mascot in college. Anna: Has arachnophobia. Dylan: Has a pet hedgehog. Jeff: Was born in France. David: Can juggle. Anna: Has arachnophobia. Code Listing 209 106arrow_forwardIn Python IDLE: How would I write a function for the problem in the attached image?arrow_forward
- Write a loop that sets each array element to the sum of itself and the next element, except for the last element which stays the same. Be careful not to index beyond the last element. Ex: Initial scores: 10, 20, 30, 40 Scores after the loop: 30, 50, 70, 40 The first element is 30 or 10 + 20, the second element is 50 or 20 + 30, and the third element is 70 or 30 + 40. The last element remains the same. 324758.2040686.qx3zqy7 1 #include 2 3 int main(void) { const int SCORES SIZE = 4; int bonusScores[SCORES_SIZE]; int i; 4 6. for (i = 0; i < SCORES_SIZE; ++i) { scanf("%d", &(bonusscores[i])); } 8 9. 10 11 12 * Your solution goes here */ 13 for (i = 0; i < SCORES_SIZE; ++i) { printf("%d ", bonusscores[i]); 14 15 16 17 printf("\n"); 18arrow_forwardWrite a loop snippet (just a piece of code, not the whole program) that will sum every third element in a 1D array. The size of the array is held in the variable MAX. You will start with the first element in the array. The name of the array is testarray.arrow_forwardIn Java Write a program that prints the 128-character ASCII table. It should print the tablein eight tab-separated columns. The first column contains a "Dec" heading (fordecimal number) with the numbers 0 through 31 below it. The second columncontains the ASCII characters associated with the numbers 0 through 31. The nextcolumns contain the subsequent numbers and associated ASCII characters. See thebelow sample output for details. Be aware that that output was produced by aprogram running in a console window in a Windows environment. If you runyour program in a different environment, the first 32 characters and the lastcharacter will probably be different from what’s shown below.Note that some characters display in a non-standard manner. For example, thenumber 7 corresponds to a bell sound. You can’t see a sound, but you can see thevacant spot for 7’s character in the below table. The number 8 corresponds to thebackspace character. You can’t see a backspace, but you can see how the…arrow_forward
- Use Java pl DataFileWords.txt: lovegentlensslovepeacelovekindnesslovepeaceunderstandingkindnessjoypeaceunderstandingfriendshipgentlenssgoodnesslovepeacegoodnesslovegentlensscontrolunderstandingunderstandinggentlensslovepeacepatiencecontrolkindnessgentlensslovepeacejoygoodnesspeacefriendshipgoodnesskindnesslovepeacegoodnesscontrolpatiencejoygentlensspatiencegentlensspatiencekindnessgoodnessjoygentlenssgentlenssarrow_forwardIn this project you will generate a poker hand containing five cards randomly selected from a deck of cards. The names of the cards are stored in a text string will be converted into an array. The array will be randomly sorted to "shuffle" the deck. Each time the user clicks a Deal button, the last five cards of the array will be removed, reducing the size of the deck size. When the size of the deck drops to zero, a new randomly sorted deck will be generated. A preview of the completed project with a randomly generated hand is shown in Figure 7-50.arrow_forwardThis is the file :arrow_forward
- PYTHON Complete the function below, which takes two arguments: data: a list of tweets search_words: a list of search phrases The function should, for each tweet in data, check whether that tweet uses any of the words in the list search_words. If it does, we keep the tweet. If it does not, we ignore the tweet. data = ['ZOOM earnings for Q1 are up 5%', 'Subscriptions at ZOOM have risen to all-time highs, boosting sales', "Got a new Mazda, ZOOM ZOOM Y'ALL!", 'I hate getting up at 8am FOR A STUPID ZOOM MEETING', 'ZOOM execs hint at a decline in earnings following a capital expansion program'] Hint: Consider the example_function below. It takes a list of numbers in numbers and keeps only those that appear in search_numbers. def example_function(numbers, search_numbers): keep = [] for number in numbers: if number in search_numbers(): keep.append(number) return keep def search_words(data, search_words):arrow_forwardWrite a program that queries information from three files(given to you). The first file contains the names and telephone numbers of a group of people. The second file contains the names and Social Security numbers of a group of people. The third file contains the names and annual income of a group of people. The groups of people should overlap. Your program should ask the user for a telephone number and then print the name, Social Security number, and annual income, if it can determine that information. Sample run1: Enter the phone number (7 digits, with a dash): 555-1234 555-1234 is associated with Bob Bob's SSN is 000300021 Bob's salary is 55000 Sample run2: Enter the phone number (7 digits, with a dash): 675-4566 Couldn't find a name associated with that number. Sample run3: Enter the phone number (7 digits, with a dash): 000-2345 000-2345 is associated with John John's SSN is 000000004 John's salary is 65000 python languagearrow_forwardCreate an ArrayList of strings to store the names of celebrities or athletes. Add five names to the list. Process the list with a for loop and the get() method to display the names, one name per line. Pass the list to a void method. Inside the method, Insert another name at index 2 and remove the name at index 4. Use a foreach loop to display the arraylist again, all names on one line separated by asterisks. After the method call in main, create an iterator for the arraylist and use it to display the list one more time.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
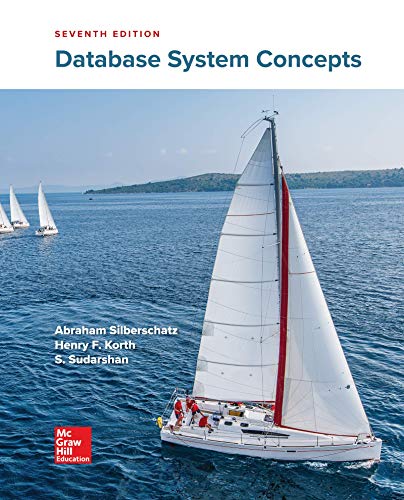
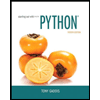
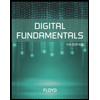
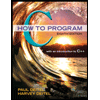
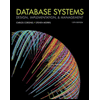
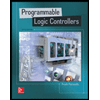