Write a program that reads a file consisting of students’ test scores in the range 0–200. It should then determine the number of students having scores in each of the following ranges: 0–24, 25–49, 50–74, 75–99, 100–124, 125–149, 150–174, and 175–200. Output the score ranges and the number of students. (Run your program with the following input data: 76, 89, 150, 135, 200, 76, 12, 100, 150, 28, 178, 189, 167, 200, 175, 150, 87, 99, 129, 149, 176, 200, 87, 35, 157, 189
Write a program that reads a file consisting of students’ test scores in the range 0–200. It should then determine the number of students having scores in each of the following ranges: 0–24, 25–49, 50–74, 75–99, 100–124, 125–149, 150–174, and 175–200. Output the score ranges and the number of students. (Run your program with the following input data: 76, 89, 150, 135, 200, 76, 12, 100, 150, 28, 178, 189, 167, 200, 175, 150, 87, 99, 129, 149, 176, 200, 87, 35, 157, 189
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Write a program that reads a file consisting of students’ test scores in the range 0–200. It should then determine the number of students having scores in each of the following ranges:
0–24, 25–49, 50–74, 75–99, 100–124, 125–149, 150–174, and 175–200.
Output the score ranges and the number of students. (Run your program with the following input data:
76, 89, 150, 135, 200, 76, 12, 100, 150, 28, 178, 189, 167, 200, 175, 150, 87, 99, 129, 149, 176, 200, 87, 35, 157, 189
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
int main()
{
int scores;
int i [8] = { 0 };
ifstream infile("Ch8_Ex4Data.txt");
if ("Ch8_Ex4Data.txt")
{
while (infile >> scores)
{
if (scores >= 0 && scores <= 24)
i[0]++;
if (scores >= 25 && scores <= 49)
i[1]++;
if (scores >= 50 && scores <= 74)
i[2]++;
if (scores >= 75 && scores <= 99)
i[3]++;
if (scores >= 100 && scores <= 124)
i[4]++;
if (scores >= 125 && scores <= 149)
i[5]++;
if (scores >= 150 && scores <= 174)
i[6]++;
if (scores >= 175 && scores <= 200)
i[7]++;
}
}
cout << "0-24: " << i[0] << endl;
cout << "25-49: " << i[1] << endl;
cout << "50-74: " <<i[2] << endl;
cout << "75-99: " << i[3] << endl;
cout << "100-124: " << i[4] << endl;
cout << "125-149: " << i[5] << endl;
cout << "150-174: " <<i[6] << endl;
cout << "175-200: " << i[7] << endl;
return 0;
}
The output is correct but its still saying 0% when I run it. Anything wrong with it?

Transcribed Image Text:The image contains a list of numerical ranges and their corresponding values, which could represent a frequency distribution or histogram data. Here is the transcription:
```
0-24: 1
25-49: 2
50-74: 0
75-99: 6
100-124: 1
125-149: 3
150-174: 5
175-200: 8
```
At the bottom, there is a terminal prompt displaying "sandbox $", indicating that this data might be part of a command-line output or simulation environment.
Explanation:
- The numbers on the left represent different ranges or "bins."
- The numbers on the right represent the frequency, or how many data points fall within each of those ranges.
- For example, the range "175-200" has the highest count of 8, indicating more data points fall within this bin compared to others.
- The range "50-74" has a frequency of 0, indicating no data points fall within this range.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Recommended textbooks for you
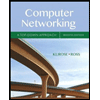
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
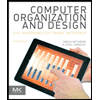
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
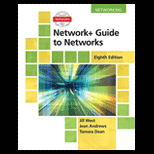
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
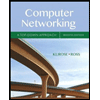
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
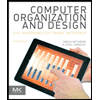
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
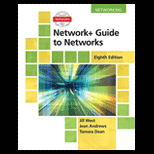
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
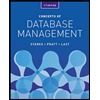
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
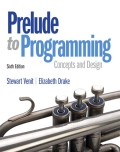
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
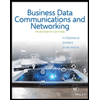
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY