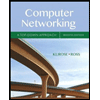
-
Write a program that can be used to calculate the federal tax. The tax is calculated as follows: For single people, the standard exemption is $4,000; for married people, the standard exemption is $7,000. A person can also put up to 6% of his or her gross income in a pension plan. The tax rates are as follows: If the taxable income is:
-
Between $0 and $15,000, the tax rate is 15%.
-
Between $15,001 and $40,000, the tax is $2,250 plus 25% of the taxable income over $15,000.
-
Over $40,000, the tax is $8,460 plus 35% of the taxable income over $40,000.
Prompt the user to enter the following information:
-
Marital status
-
If the marital status is “married,” ask for the number of children under the age of 14
-
Gross salary (If the marital status is “married” and both spouses have income, enter the combined salary.)
-
Percentage of gross income contributed to a pension fund
Your program must consist of at least the following functions:
-
Function getData: This function asks the user to enter the relevant data.
-
Function taxAmount: This function computes and returns the tax owed.
To calculate the taxable income, subtract the sum of the standard exemption, the amount contributed to a pension plan, and the personal exemption, which is $1,500 per person. (Note that if a married couple has two children under the age of 14, then the personal exemption is $1500 * 4 = $6000 )
-

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- Write a C# program that plays a guessing game with the user. Your program should select a random number between 1 and 50 and then let the user try to guess it. The program should continue asking the user for a number until he guesses correctly. (See below for some tips on random numbers). CHALLENGE #1: Modify your program so that it only allows the user 10 guesses, and then declares them to be an inadequate guesser if they haven’t gotten it correct. Your program should output the random number chosen. CHALLENGE #2: Modify your program so that after they guess a number (or get declared inadequate, if you do Challenge #1) that it asks them if they want to play again, and responds accordingly. Some Random Number Generation HintsRandom rndNumber = new Random();Console.WriteLine(rndNumber.Next()); //random integerConsole.WriteLine(rndNumber.Next(101)); //random integer between 0 and 100Console.WriteLine(rndNumber.Next(10, 43)); //random integer between 10 and…arrow_forwardpython3 problemarrow_forwardWrite a program that will calculate the cost of carpeting. We-do-Carpets is a company that specializes in carpeting homes. The company is interested in new software for their sales staff. The software needs to provide quotes to potential customers. Your program should: Prompt the user to enter in lengths and widths for the following rooms Living Room Master Bedroom Family Room Kids Bedroom Calculate the cost of carpeting for each room Calculate the sub total cost for all rooms Calculate the 6.625 NJ Sales Tax Calculate the grand Total use java programming Your program must be formatted according to the output Sample Outputarrow_forward
- You are working for a lumber company, and your employer would like a program that calculates the cost of lumber for an order. The company sells pine, fir, cedar, maple, and oak lumber. Lumber is priced by board feet. One board foot equals one square foot that is one inch thick. Theprice per board foot is given in the following table: Pine 0.89Fir 1.09Cedar 2.26 Maple 4.50Oak 3.10 The lumber is sold in different dimensions (specified in inches of width and height, and feet of length) that need to be converted to board feet. For example, a 2 x 4 x 8 piece is 2 inches wide, 4 inches high, and 8 feet long, and is equivalent to 5.333 board feet (2 * 4 * 8 = 64, which when divided by 12 = 5.333 board feet). An entry from the user will be in the form of a letter and four integer numbers. The integers are the number of pieces, width, height, and length. The letter will be one of P, F, C, M, O (corresponding to the five kinds of wood) or T, meaning total. When the letter is T, there are no…arrow_forwardC program onlyarrow_forwardThe ideal gas law allows the calculation of volume of a gas given the pressure(P), amount of the gas (n), and the temperature (T). The equation is:V = nRT / PSince we only have used integer arithmetic, all numbers will be integer values with no decimal points. The constant R is 8.314 and will be specified as (8314/1000). This gives the same result. Implement the idea gas law program where the user is prompted for and enters values for n, T, and P, and V is calculated and printed out. Be careful to implement an accurate version of this program. Your program should include a proper and useful prompt for input, and print the results in a meaningful manner.arrow_forward
- Imagine you are working for a lumber company, and your employer would like a program that calculates the cost of lumber for a customer order. The company sells pine, fir, cedar, maple, and oak lumber. The lumber is priced by board feet. One board foot equals one square foot that is one inch thick. The price per board foot is given in the following table: Pine 0.89 Fir 1.09 Cedar 2.26 Maple 4.50 Oak 3.10 The lumber is sold in different dimensions (specified in inches of width and height, and feet of length) that need to be converted to board feet. For example, a 2 x 4 x 8 piece is 2 inches wide, 4 inches high, and 8 feet long, and is equivalent to 5.333 board feet (2 * 4 * 8 = 64, which when divided by 12 = 5.333 board feet). Create a blank c++ Give the customer instructions and then ask the user (customer) to identify the items they wish to purchase by inputting the type of wood, the length, width, & heights of the board, and the number of boards they desire. Allow the user to…arrow_forwardAn Internet service provider has three different subscription packages for its customers:Package A: For $9.95 per month 10 hours of access are provided. Additional hours are $2.00per hour.Package B: For $13.95 per month 20 hours of access are provided. Additional hours are $1.00per hour.Package C: For $19.95 per month unlimited access is provided.Write a program that calculates a customer’s monthly bill. It should ask the user to enter theletter of the package the customer has purchased (A, B, or C) and the number of hours thatwere used. It should then display the total charges. Please program in C++ Requirements: Please include a Flowchart and the pseudocode for your programarrow_forwardWrite a program that calculate electricity bill. The program prompts the user to enter the amount of KWh units of electricity consumed, the task is to calculate the electricity bill total with the help of the below charges: • 1 to 50 units of total units - Unit price=10 • 50 to 100 units of total units - Unit price=15 • above 100 units - Unit price=20 Sample Runl: Enter the amount of KWh units U: 250 Total Bill: 4250 Explanation: Charge for the first 50 units - 10 50 500 Charge for the 50 to 100 units - 15 50 - 750 Charge for the 100 to 250 units - 20*150 - 3000 Sample Run2: Enter the amount of KWh units U: 95 Total Bill: 1175 Explanation: Charge for the first 100 units - 10*50 - 500 Charge for the 50 to 100 units - 15 45 675arrow_forward
- Using pythonarrow_forwardWrite a program that plays a dice game called "21" It is a variation on BlackJack where one player plays against the computer trying to get 21 or as close to 21 without going over. Here are the rules of the game: You will play with dice that have numbers from 1 to 11. To win, the player or the computer has to get to 21, or as close as possible without going over. If the player or computer goes over 21, they instantly lose. If there is a tie, the computer wins. Starting the game: The player is asked to give the computer a name. For now, we'll simply call the computer opponent, "computer." The game starts with rolling four dice. The first two dice are for the player. These two dice are added up and the total outputted to the screen. The other two dice are for the computer. Likewise, their total is outputted to the screen. Player: If a total of 21 has been reached by either the player or the computer, the game instantly stops and the winner is declared. Otherwise,…arrow_forwardWrite a program that asks the user to enter a number of seconds and works as follows: There are 60 seconds in a minute. If the number of seconds entered by the user is greater than or equal to 60, the program should convert the number of seconds to minutes and seconds. There are 3,600 seconds in an hour. If the number of seconds entered by the user is greater than or equal to 3,600, the program should convert the number of seconds to hours, minutes, and seconds. There are 86,400 seconds in a day. If the number of seconds entered by the user is greater than or equal to 86,400, the program should convert the number of seconds to days, hours, minutes, and seconds.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
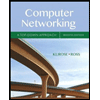
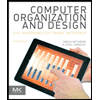
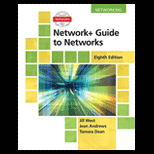
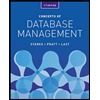
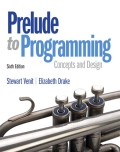
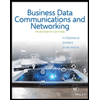