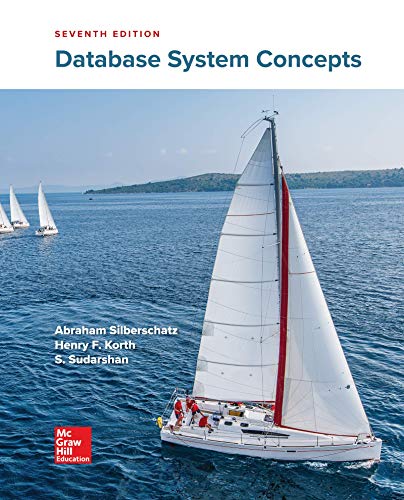
Write a program in Python on Visual Code, that displays the various coffee drinks your vending machine offers along with the option number for each drink. Beside each option include the price (you can set the cost for the small, the costs for medium should be 2 times the cost of the small and the cost of the large should be 3 times the cost of the small). These are some of the options you could choose from.
1.Espresso (Small $1.75 , Medium $3.50, Large $5.25)
2.Americano (Small $1.75 , Medium $3.50, Large $5.25)
3.Café au Lait (Small $1.75 , Medium $3.50, Large $5.25)
4.Latte (Small $1.75 , Medium $3.50, Large $5.25)
5.Cappuccino (Small $1.75 , Medium $3.50, Large $5.25)
6.Macchiato (Small $1.75 , Medium $3.50, Large $5.25)
7.Mocha (Small $1.75 , Medium $3.50, Large $5.25)
For example: Then prompt the user for their drink selection and enter their selection. Record the cost of the small drink into a variable that will be used to compute the amount due. Display a message such as “You selected Mocha ”. If the user does not enter a valid option, print an error message and exit the program. You should use a conditional statement for this problem. To check that you have recorded the amount due properly, after the statement, display the amount due for the small size of the drink selected. You must offer at least 4 drink options.

Trending nowThis is a popular solution!
Step by stepSolved in 4 steps with 4 images

- In this lab, you use the flowchart and pseudocode found in the figure below to add code to a partially created Java program. When completed, college admissions officers should be able to use the Java program to determine whether to accept or reject a student, based on his or her test score and class rank. start input testScore, classRank if testScore >= 90 then if classRank >= 25 then output "Accept" else output "Reject" end if else if testScore >= 80 then if classRank >= 50 then output "Accept" else output "Reject " endif else if testScore >= 70 then if classRank >= 75 then output "Accept" else output "Reject" end if else out put "Reject" end if end if end if stop Instructions Study the pseudocode in picture above. Declare the variables testScoreString and classRankString. Write the interactive input statements to retrieve: A student’s test score (testScoreString) A student's class rank (classRankString) Write the statements to convert the string representation…arrow_forwardHello, I need to solve this problem with C++ programming language using Visual Studio. Thank you.arrow_forwardYou are about to embark on a trip. You know your start and finish locations. However, you have not settled on a formal route for the trip. You will use Google Maps for your trip. To help make the decision, we will create a trip planning report. The user will enter three alternate routes for the trip which will have different mileages but will use the same starting and finishing locations. This program can be used to create a trip report regardless of the mileage of the trip.The input menus will not specify the route, but will:• Show a descriptive route name• A mileage value• An estimated time (to the nearest decimal - remember, 6 minutes, is 1/10 of an hour- The user may adjust the time if they wish ) from Google Maps.• If the route is expected to have any direct route expenses (tolls, ferries, etc.)• A default valueMenu example:• Menu 1 - Tampa, Florida via I-75 | 1067.00 |15.33 | N• Menu 2 - Tampa, Florida via I-77 | 1028.00 | 15.33 | N• Menu 3 - Tampa, Florida via I-65 | 1124.00 |…arrow_forward
- Program in C++ & Visual Studio not Studio Code Everyone has played Yahtzee... Right? There are so many better dice games, but my family likes this one the best. A YouTube video that describes Yahtzee can be found here. The basic rules are as follows: At the start of your turn you roll five normal everyday six sided dice. In the course of your turn you would choose to reroll any of the dice up to two more times. We are more concerned in this problem with the dice at the completion of your turn. This website gives a great rundown of the probabilities of Yahtzee that you will need. NOTE: You may ONLY use a set and map data structure to complete the solution to this problem Let’s see what results the dice give us using the following data structures and process: Prompt the user to enter five valid numbers (Range: One to six inclusive on both ends) Each time the user enters a valid number, “place” it into a map of integersCreate and use a set of integers with your data to…arrow_forwardCreate a Raptor Flow Chart for this problem (hand written or using a software) ; screen capture it or save it as a Picture. and attach it here A certain electrical company charges its customers for electricity as follows. Kilowatt-Hours (kWh) O to 500 501 to 1000 over 1000 Cost per kWh (in pesos) 10 10 + 0.05 for each kwh over 500 35 + 0.03 for each kwh above 1000 Enter the previous meter reading and the present meter reading, calculate the cost and print out how much is charged. Sample Input: Input the previous meter reading (in kWh): 1056 Input the present meter reading (in kWh): 1232 Expected Output: Electric bill (in pesos): 1,760.00 Sample Input: Input the previous meter reading (in kWh): 1056 Input the present meter reading (in kWh): 1560 Expected Output: Electric bill (in pesos): 5,040.20 Sample Input: Input the previous meter reading (in kWh): 1056 Input the present meter reading (in kWh): 2172 Expected Output: Electric bill (in pesos): 39,063.48arrow_forwardWrite a turtle graphics program that draws a city skyline like the one shown in Figure 2. The program’s overall task is to draw an outline of some city buildings against a night sky. Modularize the program by writing functions that perform the following tasks: Draw the outline of buildings. Draw some windows on the buildings. Use randomly placed dots as the stars (make sure the stars appear on the sky, not on the buildings).arrow_forward
- The text presented the Sierpinski triangle fractal. Inthis exercise, you will write a program to display another fractal, called the Kochsnowflake, named after a famous Swedish mathematician. A Koch snowflake iscreated as follows:1. Begin with an equilateral triangle, which is considered to be the Koch fractalof order (or level) 0, as shown in Figure a.2. Divide each line in the shape into three equal line segments and draw an outwardequilateral triangle with the middle line segment as the base to create aKoch fractal of order 1, as shown in Figure b.3. Repeat Step 2 to create a Koch fractal of order 2, 3, . . . , and so on, as shownin Figures c and d.arrow_forwardDevelop a program that allows the user to enter a start value of 1 to 4, a stop value of 5 to 12 and a multiplier of 2 to 8. The program must display a multiplication table with results using these values. For example, if the user enters a start value of 3, a stop value of 7 and a multiplier value of 3, the table should be displayed as follows: Multiplication Table 3 x 3 = 9 3 x 4 = 12 3 x 5 = 15 3 x 6 = 18 3 x 7 = 21 additionally Each multiplication problem must be displayed with a problem number. The program must prompt the user to enter an answer to each of the multiplication problems. The correct answer should be displayed after the user enters an answer. A message should be displayed informing the user that the answer entered was correct or incorrect followed by an appropriate motivating comment. A running record of the number of correct and incorrect responses must be kept. After all the problems have been presented the user must be informed of his/her performance; number of…arrow_forwardWith clear comments in visual studio.arrow_forward
- writing a program that will ask the user to input four pieces of information: Their first name Their last name Their student ID number. Only the numbers after any leading 0’s. The amount of a scholarship Your program will provide a display where it will show where the user has been asked for the information, followed by a statement that will display the information entered as a confirmation statement on the screen, then a list of classes will be presented with the number of credit hours for each class and the overall enrollment at the bottom. Following the list of classes will be a billing statement. The billing statement will display the cost of tuition, the fees that are applied to a student’s account, any scholarship(s) the student has received and the total of what the student has to pay. Both the list of classes and the billing statement should be displayed in a tabular format. You must use output formatting such as set-width, and alignment to create the tabular display. For…arrow_forwardPython. Use decimal modulearrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
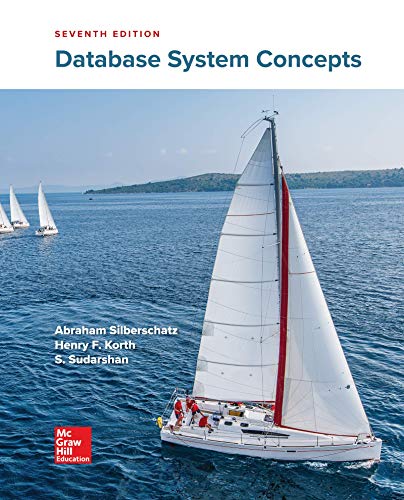
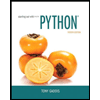
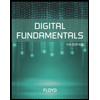
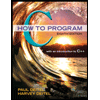
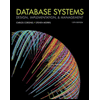
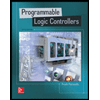