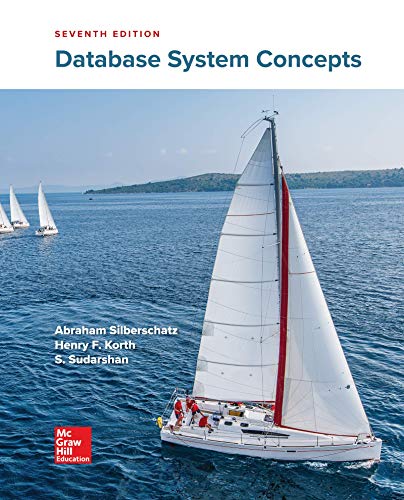
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
Write a program in Java to manipulate a Double Linked List:
-
Count the number of nodes
-
Insert a new node before the value 7 of Double Linked List
-
Search an existing element in a Double linked list (the element of search
is given by the user)
Suppose List contained the following Test Data: Input the number of nodes : 4
Input data for node 1 : 5
Input data for node 2 : 6Input data for node 3 : 7 Input data for node 4: 9
Expert Solution

arrow_forward
Step 1
Algorithm:
- Start
- Implement a class Node with attributes data,next which is pointing to next node and prev which is pointing to previous node
- Create a class LinkedList with head as its attribute.
- Implement constructor to Initialize the data
- Implement a method addNode() which adds node to the linked list
- Implement a method searchNode() which searches for a node with particular data in the list. If the node is present in the list, print node is present else print node is not present
- Implement a function count() which prints the count of number of elements in the list
- Implement a function printList() which prints the list data
- Inside the main method, create object of LinkedList
- Read no.of elements in the list n
- Read n numbers add the data to list by calling addNode()
- Call count() which prints number of elements in the list
- Read the search data
- Call searchNode() by passing search data
- Stop
Step by stepSolved in 4 steps with 2 images

Knowledge Booster
Similar questions
- def reverse_list (1st: List [Any], start: int, end: int) -> None: """Reverse the order of the items in list , between the indexes and (including the item at the index). If start or end are out of the list bounds, then raise an IndexError. The function must be implemented **recursively**. >>> lst ['2', '2', 'v', 'e'] >>>reverse_list (lst, 0, 3) >>> lst ['e', 'v', 'i', '2'] >>> lst [] >>>reverse_list (lst, 0, 0) >>> lst [0] >>> Ist = [] >>> reverse_list (lst, 0, 1) Traceback (most recent call last): IndexError #1 #1arrow_forwardJavaScript Given a singly linked list of integers, determine whether or not it's a palindrome. // Singly-linked lists are already defined with this interface: // function ListNode(x) { // this.value = x; // this.next = null; // } // function isListPalindrome(head) { } Note: in examples below and tests preview linked lists are presented as arrays just for simplicity of visualization: in real data you will be given a head node l of the linked list Example For l = [0, 1, 0], the output should beisListPalindrome(l) = true; For l = [1, 2, 2, 3], the output should beisListPalindrome(l) = false.arrow_forwardAssume a linked list contains following integers: 7, 2, 9, 5, 8, 3, 15 and the pointer head is pointing to the first node of the list. What will be the value of variable a after the following statements are executed: Node<int> *curNode=head; Node<int> *aNode; int s; while(curNode!=NULL){ { aNode=curNode; curNode=curNode->getNext(); } s=aNode->getItem(); A. 15 B. 3 C. 7 D. 2arrow_forward
- A(n) array can be used in an array implementation of a queue to avoid an overflow error at the rear of the queue when the queue is not full.arrow_forwardPython data structures: write a function that takes in a list (L) as input, and returns the number of inversions. An inversion in L is a pair of elements x and y such that x appears before y in L, but x > y. Your function must run in -place and implement the best possible algoritm of time complexity. def inversions(L: List) -> int:'''finds and returns number of inversions'''arrow_forwardAssume a linked list contains following integers: 5, 2, 4, 6, 8, 3, 15 and the pointer head is pointing to the first node of the list. What will be the value of variable a after the following statements are executed: Node<int> *curNode=head; int a=0; while(curNode!=NULL){ a+=curNode->getItem(); curNode=curNode->getNext(); if(curNode!=NULL) curNode=curNode->getNext(); } A.32 B.43 C.19 D.18arrow_forward
- Assume a linked list contains following integers: 2, 2, 4, 5, 8, 3, 15 and the pointer head is pointing to the first node of the list. What will be the value of variable a after the following statements are executed: Node<int> *curNode=head; int a=0; Node<int> *aNode; while(curNode->getItem()<7){ aNode=curNode; curNode=curNode->getNext(); } a=aNode->getItem(); A. 5 B.8 C.0 D.7arrow_forwardThis program will use an array implementation of a linked list to keep an ascending sorted list of numbers. The input data consists of two fields: a single character and an integer value. The single character gets interpreted as one of the following: A - represents a value that is to be added to the linked listD - represents a value to be deleted from the linked listP - indicates that all values are to be printed in ascending order The character is only used to determine which process is to be executed; it should not be printed, entered into the linked list, or processed in any way. The main program should be mainly function calls. Process input data until end of file. All output should be sent to an output file and be appropriately formatted and labeled. Keep a running log of everything that is going on in the processing. Input file: A 54A -17A 32 A 81 A -30 A 18 P A 41 A 93 A 65 A 80 A 104 A -20 D 81 D 54 D -30 A 79 A 63 A 77 A –33 D 104 D -20 D 80 D 32 Parrow_forwardCode in Python:arrow_forward
arrow_back_ios
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
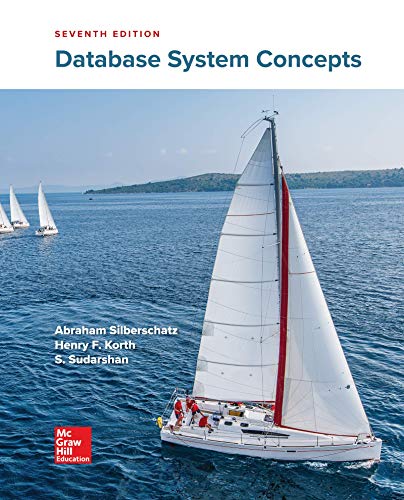
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
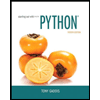
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
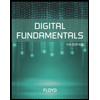
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
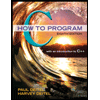
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
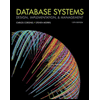
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
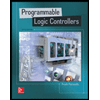
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education