Write a program in c++ to generate a random number between 1 - 100, and then display which quartile the number falls in. First quartile is 1 - 25 Second quartile is 26 - 50 Third quartile is 51 - 75 Fourth quartile is 76 - 100 The following program shows how to get a random integer between 1 - 100. Use the code as an example for your own program. SAMPLE PROGRAM// This program displays a random number between 1 - 100#include <iostream> // for input and output#include <cstdlib> // for rand() and srand()#include <ctime> // for time()using namespace std;int main(){ //constantsconst int MIN_VALUE = 1;const int MAX_VALUE = 100;//get system time and seed random number generatorunsigned seed = time(0);srand(seed);//get random number between 1 - 100int num = (rand() % (MAX_VALUE - MIN_VALUE + 1)) + MIN_VALUE;cout << num << endl;return 0;} Your program should: contain header comments as shown in class display a "hello" message generate a random number between 1 - 100 display the number back to the user determine which quartile the number falls in (using any combination of if, else, and else if) display the quartile back to the user display a "goodbye" message before exiting the program
Write a
- First quartile is 1 - 25
- Second quartile is 26 - 50
- Third quartile is 51 - 75
- Fourth quartile is 76 - 100
The following program shows how to get a random integer between 1 - 100. Use the code as an example for your own program.
SAMPLE PROGRAM
// This program displays a random number between 1 - 100
#include <iostream> // for input and output
#include <cstdlib> // for rand() and srand()
#include <ctime> // for time()
using namespace std;
int main()
{
//constants
const int MIN_VALUE = 1;
const int MAX_VALUE = 100;
//get system time and seed random number generator
unsigned seed = time(0);
srand(seed);
//get random number between 1 - 100
int num = (rand() % (MAX_VALUE - MIN_VALUE + 1)) + MIN_VALUE;
cout << num << endl;
return 0;
}
Your program should:
- contain header comments as shown in class
- display a "hello" message
- generate a random number between 1 - 100
- display the number back to the user
- determine which quartile the number falls in (using any combination of if, else, and else if)
- display the quartile back to the user
- display a "goodbye" message before exiting the program

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

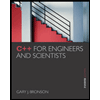
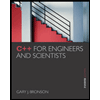