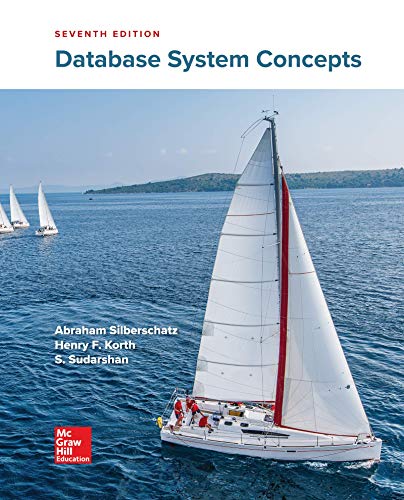
2. Test Scores
File: test_scores.py
Write pseudocode for the main() part of a
When you have written the pseudocode for main, implement your solution in Python code and test it with a range of meaningful data.
Remember that we've done the JCU grades question before, so copy your function from that practical code file.
Sample Output
Enhancements
When you have that working...
-
We asked for 4 scores. Have a look at your code... did you use 4 as a numeric literal or a constant?
Change 4 to 3... Did you have to change the program in more than one place?
If so, then you've missed one of the things we've taught...
As a strong guideline: if you need to use the same literal more than once, you should turn it into a constant .
Do this now if you haven't already. -
Add error-checking to the test score inputs to ensure they are between 0 and 100.
You should be getting good at this by now :) but do notice that it's easy to do this kind of "iterative development" where you don't try and get it working all at once. Error-checking is a common feature to add after you have the core functionality working. -
Add a feature that shows the user what the trend is.
If the last score in the list is higher than the average, then the trend is "positive", otherwise it's "not positive". -
Upgrade your print outputs so the output is formatted nicely like:

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 4 images

- Exercise 5: Please perform in C++ Write a program that lets the user perform arithmetic operations on two numbers. Your program must be menu driven, allowing the user to select the operation (+,-, *, or /) and input the numbers. ● ● ● Furthermore, your program must consist of the following functions: Function showChoice(): This function shows the options to the user and explains how to enter data. Function add(): This function accepts two numbers as arguments and returns the O O O O Function subtract(): This function accepts two numbers as arguments and returns their difference. O Function multiply(): This function accepts two numbers as arguments and returns the product. Function divide(): This function accepts two numbers as arguments and returns the quotient. sum.arrow_forwardIntro to Python Programming:arrow_forwardIn Python Text-based adventure game: Pretend you are creating a text-based adventure game. At different points in the game, you want the user to select to fight, run, or hide from certain enemies. The selection variable must be sent as an argument into the choice() function. The user should enter 1 to fight, 2 to run, or 3 to hide in the main(). The choice() function should print one of the three options. Add an if statement in the choice() function to make the correct selection.arrow_forward
- Include pseudocode that describes all steps required to solve the problem. Employ variable names that describe the values they store and adhere to Python naming conventions. Include additional comments as needed to annotate your code. Use correct spelling and grammar. Use f_strings to output variable values. Use the "dunders test" for __name__ equals __main__ 1. Write a program that contains a main function and a custom, void function named show_larger that takes two random integers as parameters. This function should display which integer is larger and by how much. The difference must be expressed as a positive number if the random integers differ. If the random integers are the same, show_larger should handle that, too. See example outputs. In the main function, generate two random integers both in the range from 1 to 5 inclusive, and call show_larger with the integers as arguments.EXAMPLE OUTPUT 13 is larger than 1 by 2EXAMPLE OUTPUT 2The integers are equal, both are 3 2. Write a…arrow_forwardUsing C++ Program: A palindrome is a word that is spelled the same way both forwards and backwards. For example, the word, 'racecar' is a palindrome. Using character arrays, write a program that prompts the user to enter a word from the keyboard and informs the user whether the word is a palindrome or not.arrow_forwardWrite VBA CODE FOR THE FOLLOWING PROBLEM Problem: The Worksheet named Grades contains a place for students to record the grades they have received in their classes. Students put the number of each letter grade received in the B column. (For example, the worksheet currently shows that the student has taken 9 classes and made five As, one B, one C, one D, and one F as grades. You need to write a program that reads the grades from the worksheet and uses a function to calculate the student’s GPA. For this problem you can assume that all classes are worth the same number of credit hours. The function should send back the GPA to the calling procedure. The procedure will then put up a message box that gives the student their GPA and a message about their GPA, all in one message box. The message should be “You qualify for an internship” if the student has a GPA of at least 3.0. It should be “You’re in the danger zone” if the GPA is between 2.0 and 3.0. And it should be “You are on…arrow_forward
- def truth_value(integer): """Convert an integer into a truth value.""" # Convert 0 into False and all other integers, # including 1, into True if integer == 0: truth_value = False else: truth_value = True return truth_value Now, write a function that goes in the opposite direction of the truth_value() function. This new function should convert a truth value into an integer. In particular, True should be converted into 1 and False into 0. Name the new function integer_0_or_1(). All you need to include below is your definition of the function integer_0_or_1(). It will be tested automatically against relevant inputs. So include your function and nothing more.arrow_forwardInstructions The python "try" keyword is very powerful in that it can, among other things, prevent a program from ending abnormally because of invalid numeric input. Write a python program with two functions/modules that does the following: .main() accepts input and calls a function to test if the input is a number and displays a message regarding the result of that numeric test • numTest() is passed an input string, tests to see if the string is numeric and returns the necessary information to main() . a NULL input (just pressing the enter key) ends the program . DO NOT USE THE BUILTIN PYTHON FUNCTION FOR NUMERIC TESTING Be sure to use clear prompts/labeling for input and output.arrow_forwardIn C language please: Write a function called printprime that takes the parameter N that prints all the prime numbers from 1 to N.arrow_forward
- Writing Functions that Return a Value Summary In this lab, you complete a partially written Python program that includes a function that returns a value. The program is a simple calculator that prompts the user for two numbers and an operator ( +, -, *, or / ). The two numbers and the operator are passed to the function where the appropriate arithmetic operation is performed. The result is then returned to where the arithmetic operation and result are displayed. For example, if the user enters 3, 4, and *, the following is displayed: 3 * 4 = 12 The source code file provided for this lab includes the necessary input and output statements. Comments are included in the file to help you write the remainder of the program. Instructions Write the Python statements as indicated by the comments. Execute the program.arrow_forwardin pythonarrow_forwardScenario: I am trying to create a recursion function that takes a number from the user. That number then goes through to equations where it squares the number and takes the square root of it. Then, that value is supposed to be sent back through the function, through the same equations. Then, I am trying to output the value of each iteration. The amount of iterations is a random number. This code is in C++ and I am using Visual Studio 2023. I have attached the code I, myself, typed and picture of the output. Also, this is a header file that is being called from my source.cpp file (case 5 in switch case). That is why I am entering 5 as the first input when I run the program. Problem: The output only shows one iteration of calculation, then goes straight back into taking an input from the user. What am I doing wrong?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
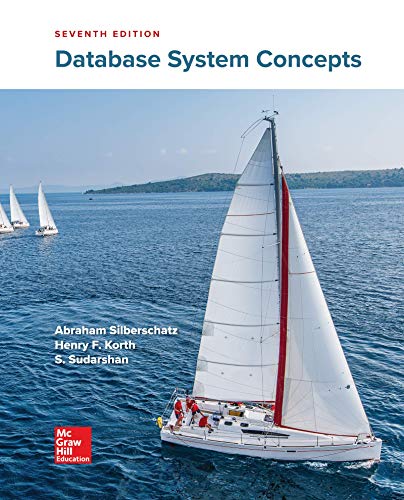
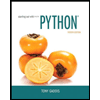
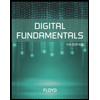
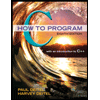
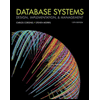
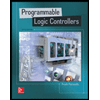