Write a program display Climate.py that performs the following functionalities a. reads the JSON file climate.json b. displays the cities as a list c. prompts the user to enter a city or cities d. searches for the relevant data and display ( (i) the average rainfall for each month as a list (ii) minimum and maximum rainfall per year. (iii) the average rainfall per year.
Please let me know the code for this as I am not able to upload the JSON file
The JSON file
provided, climate.json contains monthly climate data of over one hundred destinations and includes average high and low temperature, dry days, snow days, and rainfall, for every month.
Program Tasks
Write a program display Climate.py that performs the following functionalities
a. reads the JSON file climate.json
b. displays the cities as a list
c. prompts the user to enter a city or cities
d. searches for the relevant data and display (
(i) the average rainfall for each month as a list
(ii) minimum and maximum rainfall per year.
(iii) the average rainfall per year.
A brief introduction to the JSON file
Structure of the climate JSON file:
• JSON data works just like python dictionary and you can access the value using
dictionaryName[key]
• The JSON file contains a list of 104 dictionary items and each dictionary item has 4 keys.
• The monthlyAvg key has a list as its value, which in return contains 12 dictionary items representing each month in a year.
• You may use the website https://codebeautify.org/jsonviewer and load the file into its editor. The website will display the structure and details of this JSON file. Alternatively, you can view this file using any text editor.
• A snapshot of the structure of this file is shown on the following page.
Loading a JSON file:
with open('climate.json', 'r') as f:
# convert a json object into a readable python object
data = json.load(f)
[
{
"id": 1,
"city": "Amsterdam",
"country": "Netherlands",
"monthlyAvg": [
{
"high": 7,
"low": 3,
"dryDays": 19,
"snowDays": 4,
"rainfall": 68
},
{
"high": 6,
"low": 3,
"dryDays": 13,
"snowDays": 2,
"rainfall": 47
},
{
"high": 10,
"low": 6,
"dryDays": 16,
"snowDays": 1,
"rainfall": 65
},
{
"high": 11,
"low": 7,
"dryDays": 12,
"snowDays": 0,
"rainfall": 52
},
{
"high": 16,
"low": 11,
"dryDays": 15,
"snowDays": 0,
"rainfall": 59
},
{
"high": 17,
"low": 11,
"dryDays": 14,
"snowDays": 0,
"rainfall": 70
},
{
"high": 20,
"low": 12,
"dryDays": 14,
"snowDays": 0,
"rainfall": 74
},
{
"high": 20,
"low": 12,
"dryDays": 15,
"snowDays": 0,
"rainfall": 69
},
{
"high": 17,
"low": 10,
"dryDays": 14,
"snowDays": 0,
"rainfall": 64
},
{
"high": 14,
"low": 9,
"dryDays": 16,
"snowDays": 0,
"rainfall": 70
},
{
"high": 9,
"low": 6,
"dryDays": 20,
"snowDays": 1,
"rainfall": 82
},
{
"high": 7,
"low": 1,
"dryDays": 19,
"snowDays": 1,
"rainfall": 85
}
]
},
{
"id": 2,
"city": "Athens",
"country": "Greece",
"monthlyAvg": [
{
"high": 12,
"low": 7,
"dryDays": 21,
"snowDays": 1,
"rainfall": 53
},
{
"high": 12, ..............
Do NOT use __main__ anywhere in your program code.
Output : After user input
Enter 0 to terminate input.
Enter City to check rain fall data: Vancouver
Enter City to check rainfall data: Calgary
Enter city to check rain fall data: 0
Average monthly rain fall in mm for Vancouver:[232,122.8,154,116.4,91.7,79,41.2,54.2,67.8,158.9,250.8,208.5]
Min : 41.2 mm
Max: 250.8 mm
Average 131.44 mm
Average monthly rain fall in mm for Calgary: [11.4,10.5,17.1,28.9,60.6,113.3,59.3,58.6,35.9,17,15.8,11.2]
Min: 10.5 mm
Max: 113.3 mm
Average: 36.3 mm
Would you like to continue? press Y or N:

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

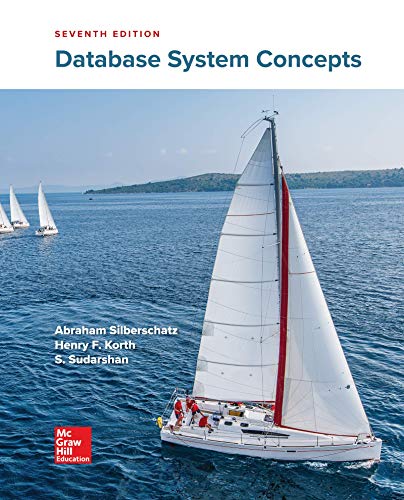
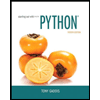
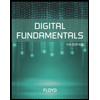
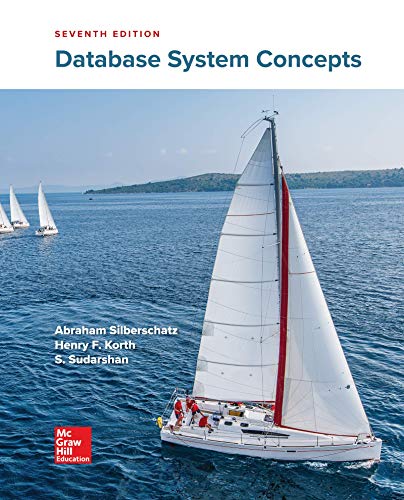
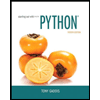
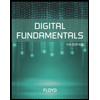
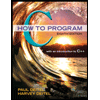
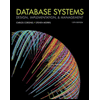
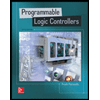