Write a program designed to get ages and heights from the user, then find the average age, average height, and average age/height ratio. */ #include #include #define MAXNUM 50 typedef struct person { int age; double height; } Person; int getData(Person people[], int max) /* Get the data from the user and put it in an array of type Person. You must use a while loop like we did for the exponent lab. Have the user enter the age prior to entering the loop (negative number or 0 to quit) Test to be sure the age is > 0, and also test to be sure the data won't overflow the array. Think about what you need to do inside of the loop. If there is too much data, print a message to the user and return to the main program with the current count and the structs that have already been stored in the array. Returns the number of people read as the value of the function. */ void getAverages(Person people[], double *aveAge, double *aveHeight, double *aveRatio, int numPeople) /* Calculate the averages here. You will loop through the array just like you have done in the past in order to include all numPeople in your calculations To find the average age/height ratio, take each persons age and divide it by their height, then calculate the average of all of those values. */ void printAverages(double aveAge, double aveHeight, double aveRatio) /* 3 lines of code to print your results! Be sure to format it nicely */ void main(void) { //declare your variables here // call function getData // do some debug prints here to be sure you have the correct count and data // call function getAverages // call function printAverages } /* A few notes: 1. You will need some local variables in your functions. 2. Hint: This line might be valuable to you: scanf("%d",&people[count].age) 3. What should you do in your main program if getData returns with no data? What would happen if you called getAverages when numPeople = 0? 4. When you are testing, you might want to make MAXNUM small, maybe 3 or 4. You can increase it when you have finished testing. 5. When you call getData from your main program, you must capitalize MAXNUM in the function call. */
Write a program designed to get ages and heights from the user, then find the average age, average height, and average age/height ratio. */ #include #include #define MAXNUM 50 typedef struct person { int age; double height; } Person; int getData(Person people[], int max) /* Get the data from the user and put it in an array of type Person. You must use a while loop like we did for the exponent lab. Have the user enter the age prior to entering the loop (negative number or 0 to quit) Test to be sure the age is > 0, and also test to be sure the data won't overflow the array. Think about what you need to do inside of the loop. If there is too much data, print a message to the user and return to the main program with the current count and the structs that have already been stored in the array. Returns the number of people read as the value of the function. */ void getAverages(Person people[], double *aveAge, double *aveHeight, double *aveRatio, int numPeople) /* Calculate the averages here. You will loop through the array just like you have done in the past in order to include all numPeople in your calculations To find the average age/height ratio, take each persons age and divide it by their height, then calculate the average of all of those values. */ void printAverages(double aveAge, double aveHeight, double aveRatio) /* 3 lines of code to print your results! Be sure to format it nicely */ void main(void) { //declare your variables here // call function getData // do some debug prints here to be sure you have the correct count and data // call function getAverages // call function printAverages } /* A few notes: 1. You will need some local variables in your functions. 2. Hint: This line might be valuable to you: scanf("%d",&people[count].age) 3. What should you do in your main program if getData returns with no data? What would happen if you called getAverages when numPeople = 0? 4. When you are testing, you might want to make MAXNUM small, maybe 3 or 4. You can increase it when you have finished testing. 5. When you call getData from your main program, you must capitalize MAXNUM in the function call. */
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please do it like someone wouldve done it as a beginer programer. Dont use pointer unless it asking for.
/*
Write a program designed to get ages and heights from the user,
then find the average age, average height, and average age/height ratio.
*/
#include <stdlib.h>
#include <stdio.h>
#define MAXNUM 50
typedef struct person
{
int age;
double height;
} Person;
int getData(Person people[], int max)
/*
Get the data from the user and put it in an array of type Person.
You must use a while loop like we did for the exponent lab. Have the user enter
the age prior to entering the loop (negative number or 0 to quit)
Test to be sure the age is > 0, and also test to be sure the data won't overflow the array.
Think about what you need to do inside of the loop.
If there is too much data, print a message to the user and return to the main program
with the current count and the structs that have already been stored in the array.
Returns the number of people read as the value of the function.
*/
void getAverages(Person people[], double *aveAge, double *aveHeight, double *aveRatio, int numPeople)
/*
Calculate the averages here.
You will loop through the array just like you have done in the past in order to include all
numPeople in your calculations
To find the average age/height ratio, take each persons age and divide it by their height,
then calculate the average of all of those values.
*/
void printAverages(double aveAge, double aveHeight, double aveRatio)
/*
3 lines of code to print your results!
Be sure to format it nicely
*/
void main(void)
{
//declare your variables here
// call function getData
// do some debug prints here to be sure you have the correct count and data
// call function getAverages
// call function printAverages
}
/*
A few notes:
1. You will need some local variables in your functions.
2. Hint: This line might be valuable to you: scanf("%d",&people[count].age)
3. What should you do in your main program if getData returns with no data? What would happen
if you called getAverages when numPeople = 0?
4. When you are testing, you might want to make MAXNUM small, maybe 3 or 4. You can
increase it when you have finished testing.
5. When you call getData from your main program, you must capitalize MAXNUM in the
function call.
*/
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
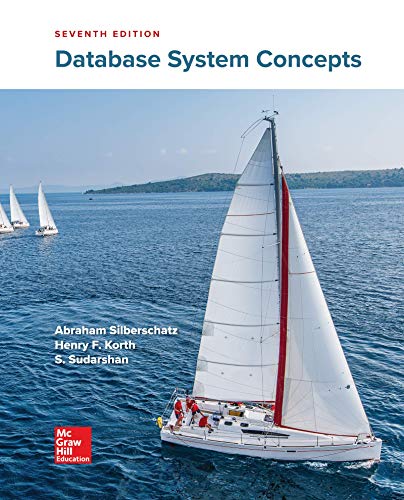
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
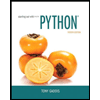
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
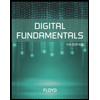
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
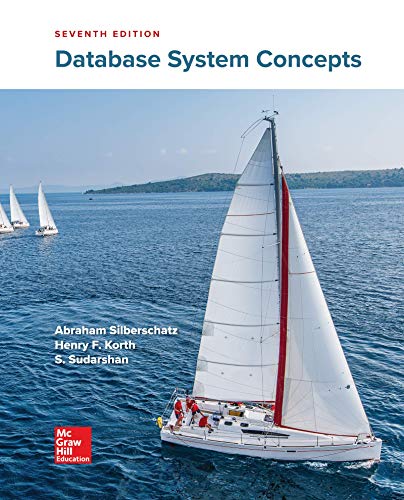
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
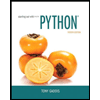
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
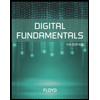
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
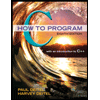
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
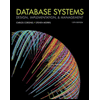
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
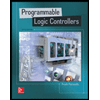
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education