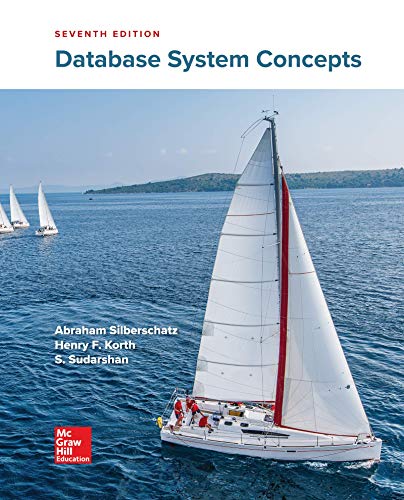
Concept explainers
In this lab, you add a loop and the statements that make up the loop body to a C++ program that is provided. When completed, the program should calculate two totals: the number of left-handed people and the number of right-handed people in your class. Your loop should execute until the user enters the character X instead of L for left-handed or R for right-handed.
The inputs for this program are as follows: R, R, R, L, L, L, R, L, R, R, L, X
Variables have been declared for you, and the input and output statements have been written.
Instructions
-
Ensure the source code file named LeftOrRight.cpp is open in the code editor.
-
Write a loop and a loop body that allows you to calculate a total of left-handed and right-handed people in your class.
-
Execute the program by clicking the Run button and using the data listed above and verify that the output is correct.
**CODE GIVEN**

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- C++ pls (use only while-loop. using for-loop will not get any credit.) Ask user how many grades you have. then ask them to enter those grades (this is a outer while-loop), then each time validate a grade(this is inner while-loop), then when you got all valid grades, find the average.arrow_forwardFor each problem write a C++ program that asks the user for input, then calculates and displays the correct output. 1) Bug Collector A bug collector collects bugs every day for seven days. Write a program that keeps a running total of the number of bugs collected during the seven days. The loop should ask for the number of bugs collected for each day, and when the loop is finished, the program should display the total number of bugs collected. 2) Calories Burned Running on a particular treadmill you burn 3.9 calories per minute. Write a program that uses a loop to display the number of calories burned after 10, 15, 20, 25, and 30 minutes. 3) Budget Analysis Write a program that asks the user to enter the amount that he or she has budgeted for a month. A loop should then prompt the user to enter each of his or her expenses for the month, and keep a running total. When the loop finishes, the program should display the amount that the user is over or under budget. 4) Sum of Numbers…arrow_forward1084 QSearch Write a C++ program, which calculates the area of 6 circles and their total sum. The program should use a loop, which asks the user to input a value for the radius, 6 times (one radius at a time), then calculates the area of each circle, calculates the total sum of these six areas, and finally displays the individual areas and their sum. Note: area = pi"r, where pi-3.14; and r is the radius of a circle. Screenshot Screenshot 2021-08...10.23.40 2021-08...10.11.34 pparrow_forward
- In this lab, you use a counter-controlled while loop in a C++ program provided for you. When completed, the program should print the numbers 0 through 10, along with their values multiplied by 2 and by 10. The data file contains the necessary variable declarations and some output statements. Instructions Ensure the source code file named Multiply.cpp is open in the code editor. Write a counter-controlled while loop that uses the loop control variable to take on the values 0 through 10. Remember to initialize the loop control variable before the program enters the loop. In the body of the loop, multiply the value of the loop control variable by 2 and by 10. Remember to change the value of the loop control variable in the body of the loop. Execute the program by clicking the Run button. Record the output of this program. Strictly use the given code // Multiply.cpp - This program prints the numbers 0 through 10 along // with these values multiplied by 2 and by 10. // Input:…arrow_forwardusing loops in C++ Write a program that will predict the size of a population of organisms. The program should ask the user for the starting number of organisms, their average daily population increase (as a percentage, expressed as a fraction in decimal form: for example 0.052 would mean a 5.2% increase each day), and the number of days they will multiply. A loop should display the size of the population for each day.Prompts, Output Labels and Messages .The three input data should be prompted for with the following prompts: "Enter the starting number of organisms: ", "Enter the average daily population increase (as a percentage): , and "Enter the number of days they will multiply: " respectively. The population sizes displayed should appear on separate lines, each of the form "On day D the population size was P." where D is the day number (starting with 1) and P is the population you calculated for that day.Input Validation.Do not accept a number less than 2 for the starting size of…arrow_forwardIn this lab, you use a counter-controlled while loop in a C++ program provided for you. When completed, the program should print the numbers 0 through 10, along with their values multiplied by 2 and by 10. The data file contains the necessary variable declarations and some output statements. Instructions Ensure the source code file named Multiply.cpp is open in the code editor. Write a counter-controlled while loop that uses the loop control variable to take on the values 0 through 10. Remember to initialize the loop control variable before the program enters the loop. In the body of the loop, multiply the value of the loop control variable by 2 and by 10. Remember to change the value of the loop control variable in the body of the loop. Execute the program by clicking the Run button. Record the output of this program. **CODE GIVEN** // Multiply.cpp - This program prints the numbers 0 through 10 along // with these values multiplied by 2 and by 10. // Input: None // Output:…arrow_forward
- In Java, If a semicolon is placed at the end of a for statement, the action of the for loop is empty. True or Falsearrow_forwardCode should be in Python. Prompt the user to enter a string of their choosing. Output the string.Ex: Enter a sentence or phrase: The only thing we have to fear is fear itself. You entered: The only thing we have to fear is fear itself. Complete the get_num_of_characters() function, which returns the number of characters in the user's string. We encourage you to use a for loop in this function. Extend the program by calling the get_num_of_characters() function and then output the returned result. Extend the program further by implementing the output_without_whitespace() function. output_without_whitespace() outputs the string's characters except for whitespace (spaces, tabs). Note: A tab is '\t'. Call the output_without_whitespace() function in main().Ex: Enter a sentence or phrase: The only thing we have to fear is fear itself. You entered: The only thing we have to fear is fear itself. Number of characters: 46 String with no whitespace: Theonlythingwehavetofearisfearitself.arrow_forwardThis program in C++ obtains a series of letter grades as input from a user. The program should calculate and output the total grade points and the grade point average (note: you may have to track the total number of inputs and the grade points to calculate the grade point average). The algorithm should execute 3 times for testing purposes.Use the appropriate loop for the main structure of the program that will stop accepting letter grades when the user inputs X. Also, choose the appropriate selection statement to convert the letter grade to the correct grade points.Additional Requirements:• Use appropriate data types and variable names throughout the code.• Use constants appropriately. Output needs to look like the image below.arrow_forward
- In this lab, you use a counter-controlled while loop in a C++ program provided for you. When completed, the program should print the numbers 0 through 10, along with their values multiplied by 2 and by 10. The data file contains the necessary variable declarations and some output statements. Instructions Ensure the source code file named Multiply.cpp is open in the code editor. Write a counter-controlled while loop that uses the loop control variable to take on the values 0 through 10. Remember to initialize the loop control variable before the program enters the loop. In the body of the loop, multiply the value of the loop control variable by 2 and by 10. Remember to change the value of the loop control variable in the body of the loop. Execute the program by clicking the Run button. Record the output of this program. **CODE GIVEN** // Multiply.cpp - This program prints the numbers 0 through 10 along // with these values multiplied by 2 and by 10. // Input: None // Output:…arrow_forwardUsing a Sentinel Value to Control a while Loop in C++ In this lab, you write a while loop that uses a sentinel value to control a loop in a C++ program that has been provided. You also write the statements that make up the body of the loop. The source code file already contains the necessary variable declarations and output statements. Each theater patron enters a value from 0 to 4 indicating the number of stars the patron awards to the Guide’s featured movie of the week. The program executes continuously until the theater manager enters a negative number to quit. At the end of the program, you should display the average star rating for the movie. Instructions Ensure the source code file named MovieGuide.cpp is open in your code editor. Write the while loop using a sentinel value to control the loop, and write the statements that make up the body of the loop. The output statements within the loop have already been written for you. Ensure you include the calculations to compute the…arrow_forwardC++, use WHILE loop with ODD numbersarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
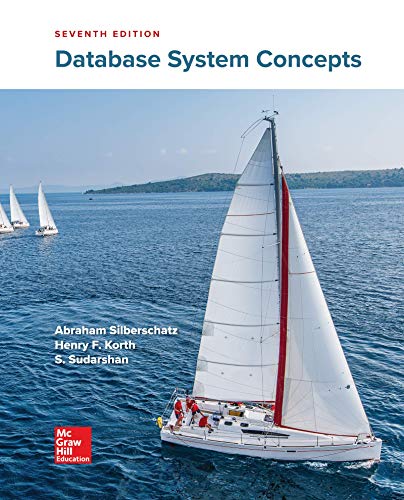
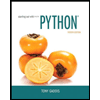
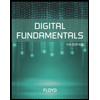
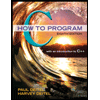
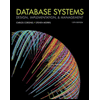
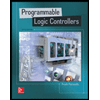