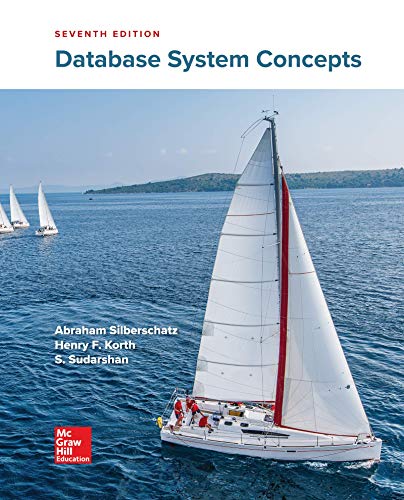
Concept explainers
Write a java program to perform statistical analysis of scores for a class of students. The class may have up to 40 students.There are five quizzes during the term. Each student is identified by a four-digit student ID number.
The program is to print the student scores and calculate and print the statistics for each quiz. The output is in the same order as the input; no sorting is needed. The input is to be read from a text file. The output from the program should be similar to the following:
Here is some sample data (not to be used) for calculations:
Stud Q1 Q2 Q3 Q4 Q5
1234 78 83 87 91 86
2134 67 77 84 82 79
1852 77 89 93 87 71
High Score 78 89 93 91 86
Low Score 67 77 84 82 71
Average 73.4 83.0 88.2 86.6 78.6
The program should print the lowest and highest scores for each quiz.
Plan of Attack
Learning Objectives
You will apply the following topics in this assignment:
File Input operations.
Working and populating an array of objects.
Wrapper Classes.
Object Oriented Design and Programming.
Understanding Requirements
Here is a copy of actual data to be used for input.
Stud Qu1 Qu2 Qu3 Qu4 Qu5
1234 052 007 100 078 034
2134 090 036 090 077 030
3124 100 045 020 090 070
4532 011 017 081 032 077
5678 020 012 045 078 034
6134 034 080 055 078 045
7874 060 100 056 078 078
8026 070 010 066 078 056
9893 034 009 077 078 020
1947 045 040 088 078 055
2877 055 050 099 078 080
3189 022 070 100 078 077
4602 089 050 091 078 060
5405 011 011 000 078 010
6999 000 098 089 078 020
Essentially, you have to do the following:
Read Student data from a text file.
Compute High, Low and Average for each quiz.
Print the Student data and display statistical information like High/Low/Average..
Design
This program can be written in one class. But dividing the code into simple and modular classes based on functionality, is at the heart of Object Oriented Design.
You must learn the concepts covered in the class and find a way to apply.
Please make sure that you put each class in its own .java file.
package lab2;
class Student {
private int SID;
private int scores[] = new int[5];
//write public get and set methods for
//SID and scores
//add methods to print values of instance variables.
}
/************************************************************************************/
package lab2;
class Statistics
{
int [] lowscores = new int [5];
int [] highscores = new int [5];
float [] avgscores = new float [5];
void findlow(Student [] a) {
/* This method will find the lowest score and store it in an array names lowscores. */
}
void findhigh(Student [] a) {
/* This method will find the highest score and store it in an array names highscores. */
}
void findavg(Student [] a) {
/* This method will find avg score for each quiz and store it in an array names avgscores. */
}
//add methods to print values of instance variables.
}
************************************************************************************/
package lab2;
class Util {
Student [] readFile(String filename, Student [] stu) {
//Reads the file and builds student array.
//Open the file using FileReader Object.
//In a loop read a line using readLine method.
//Tokenize each line using StringTokenizer Object
//Each token is converted from String to Integer using parseInt method
//Value is then saved in the right property of Student Object.
}
}
************************************************************************************/
//Putting it together in driver class:
public static void main(String [] args) {
Student lab2 [] = new Student[40];
//Populate the student array
lab2 = Util.readFile("filename.txt", lab2);
Statistics statlab2 = new Statistics();
statlab2.findlow(lab2);
//add calls to findhigh and find average
//Print the data and statistics
}
Topics to Learn
Working with Text Files
//ReadSource.java -- shows how to work with readLine and FileReader
public class ReadSource {
public static void main(String[] arguments) {
try {
FileReader file = new FileReader("ReadSource.java");
BufferedReader buff = new BufferedReader(file);
boolean eof = false;
while (!eof) {
String line = buff.readLine();
if (line == null)
eof = true;
else
System.out.println(line);
}
buff.close();
} catch (IOException e) {
System.out.println("Error -- " + e.toString());
}
}
}
//How do you tokenize a String? You can use other ways of doing this, if you like.
StringTokenizer st = new StringTokenizer("this is a test");
while (st.hasMoreTokens()) {
System.out.println(st.nextToken());
}
//How to convert a String to an Integer
int x = Integer.parseInt(String) ;
Please be sure to include a class diagram

Trending nowThis is a popular solution!
Step by stepSolved in 7 steps with 2 images

- PROBLEM 1: Have you ever wondered how websites validate your credit card number when you shop online? They do not check a large database of numbers. Most credit providers rely on a checksum formula for distinguishing valid numbers from random collections of digits (or typing mistakes). The objective of this lab you will implement a program that read a file that contains a table with two columns: A column of customer names and a column of credit card numbers. For each customer, print the validity of the credit card number and name of the corresponding credit card company (if the number is valid), For our purpose, the algorithm that valid credit cards is the following: Double the value of every second digit beginning from the right. That is, the last digit is unchanged; the second-to-last digit is doubled; the third-to-last digit is unchanged; and so on. For example, [1,3,8,6] becomes [2,3,16,6] Add the digits of the doubled values and the undoubled digits from the original number. For…arrow_forwardThe objective of this problem is to show that you can write a program that reads a text file and uses string methods to manipulate the text. File cart.txt contains shopping cart type data from golfsmith.com representing the gifts you have purchased for your dad for this past Fathers Day. Good for you. You have been very generous! The first item in each row of the file is the part number, the second the quantity, the third the price and the fourth the description. Your program will read the file line by line and compute the total cost of all of the items in the list. You will then display a message that looks like this, assuming the cost of each item adds up to 168.42: Here's what your output should look like: The total price for the awesome Fathers Day gifts you bought is $168.42. The shopping cart can be found here: cart.txt. Right click on the link and save the file to the folder where you have your Python files.arrow_forwardPYTHON: I need to get the avg statement out of the loop so that the output prints correctly. Any suggestions. Write a program that reads the student information from a tab separated values (tsv) file. The program then creates a text file that records the course grades of the students. Each row of the tsv file contains the Last Name, First Name, Midterm1 score, Midterm2 score, and the Final score of a student. A sample of the student information is provided in StudentInfo.tsv. Assume the number of students is at least 1 and at most 20. The program performs the following tasks: Read the file name of the tsv file from the user. Open the tsv file and read the student information. Compute the average exam score of each student. Assign a letter grade to each student based on the average exam score in the following scale: A: 90 =< x B: 80 =< x < 90 C: 70 =< x < 80 D: 60 =< x < 70 F: x < 60 Compute the average of each exam. Output the last names, first names, exam…arrow_forward
- can you do it pythonarrow_forwardDescriptionWrite a program to compute average grades for a course. The course records are in a single file and are organized according to the following format: each line contains a student's first name, then one space, then the student's last name, then one space, then some number of quiz scores that, if they exist, are separated by one space. Each student will have zero to ten scores, and each score is an integer not greater than 100. Your program will read data from this file and write its output to a second file. The date in the output file will be nearly the same as the data in the input file except that you will print the names as last-name, first-name; each quiz score, and there will be one additional number at the end of each line:the average of the student's ten quiz scores.Both files are parameters. You can access the name of the input file with argv[1]. and the name of the output file with argv[2].The output file must be formatted as described below: 1. First and last names…arrow_forwardPlease use Replit to complete in C programming language. PLEASE FOLLOW guidelines CLOSELY AND SHOW OUTPUTSarrow_forward
- JAVA PROGRAM (Create large dataset) Create a data file with 500 lines. Each line in the file consists of a faculty member’s first name, last name, rank, and salary. The faculty member’s first name and last name for the ith line are FirstNamei and LastNamei. The rank is randomly generated as assistant, associate, and full. The salary is randomly generated as a number with two digits after the decimal point. The salary for an assistant professor should be in the range from 50,000 to 80,000, for associate professor from 60,000 to 110,000, and for full professor from 75,000 to 130,000. Save the file in Salary.txt. Here are some sample data: FirstName1 LastName1 assistant 60055.95 FirstName2 LastName2 associate 81112.45 . . . FirstName500 LastName500 full 92255.21arrow_forwardProblem A straight line can be defined by a pair of points p1(x1, yı) and p2(x2, y2). The slope m of a line is defined as follow: У2 — У1 m = X2 - X1 Your program reads the points from a text file called 'points.txt’ which contains the coordinates of unknown number of pairs of points as shown in Figure 1. Each line contains four values x1, y1, x2, y2, where x1, yl are the coordinates of the first point and x2, y2 are the coordinates of the second point. 10 -2 7.5 -3.2 4 15.5 -4.6 21 -2 Зр 12.5 6. 2 5 -3 -6 10 3 10 15 Figure 1. Input file contains unknown number of point pairs Use Spider, to create the following files: (i) The input file 'points.txt' shown in Figure 1. Your Python program that reads from the input file 'points.txt', the coordinates of unknown number of pairs of points, computes the corresponding slopes then prints the results on the screen as shown in Figure 2. (ii) Line # X1 Y1 X2 Y2 slope 1 10.00 -2.00 7.50 -3.20 0.48 2 4.00 15.50 -4.60 21.00 -0.64 3 0.00 0.00 0.00…arrow_forwardDesign a modular program in Python to process payroll for all hourly paid employees, save all the data into a text file and make a backup file as well. The program should allow user enter each employee's ID, name, hours worked, and hourly pay rate. And then calculate gross pay with overtime hours(hours beyond 40) paid 50% more. And then the program should display pay statement for each employee and store them into a same text file. The program should display total number of employees, total number of hours worked by all employees, and total gross pay and save them at the end of the same text file as well. The program should contain the following functions: main(), get_employee_info(), calc_gross_pay(hours, rate), display_pay_statement(emp_id, emp_name, hours_horked, hourly_pay_rate, gross_pay), save_pay_statement_to_file(file_object, emp_id, emp_name, hours_worked, hourly_pay_rate, gross_pay), save_summary_to_file(file_object, number_of_employees, total_hours_worked, total_gross_pay).…arrow_forward
- 9b_act2. Please help me answer this in python programming.arrow_forwardGiven a text file containing the availability of food items, write a program that reads the information from the text file and outputs the available food items. The program first reads the name of the text file from the user. The program then reads the text file, stores the information into four separate lists, and outputs the available food items in the following format: name (category) - description Assume the text file contains the category, name, description, and availability of at least one food item, separated by a tab character ('\t'). Ex: If the input of the program is: food.txt and the contents of food.txt are: Classic ham sandwich Available sandwich Chicken salad sandwich Not available Classic cheeseburger Cheeseburger Not available Salads Water 16oz bottled water Available Caesar salad Chunks of romaine heart lettuce dressed with lemon juice Available Salads Asian salad Mixed greens with ginger dressing, sprinkled with sesame Not available Beverages Beverages Mexican food…arrow_forwardIn a text file, I have two numbers each line. The numbers are the employee ID and the number of hours the employee work. My goal of this program is to read the both employee ID and the number of hours the employee work and print it out in the main program. If the list of employee id is not in order, sort it in order and still print it out in the main program. Additionally, after printing them out, print out the total number of employees and the total number of hours all the employee work. Write this in java For example File.txt EmployeeID Number of Hours Work 3 12 2 20 1 40 5 20 7 9 In the main program print: EmployeeID Number of Hours Work 1 40 2 20 3 12 5 20 7 9arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
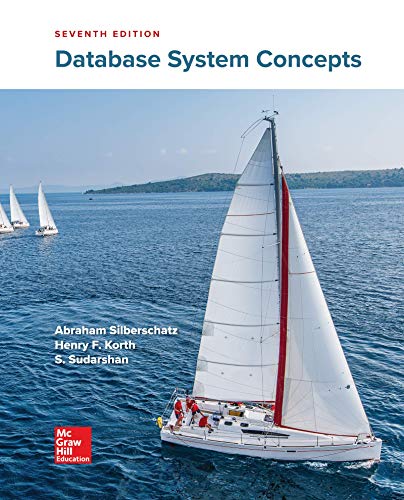
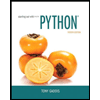
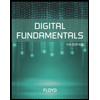
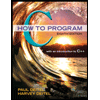
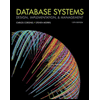
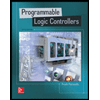