Java only Design, implement and test a Java class that processes a series of triangles. For this assignment, triangle data will be read from an input file and the program’s output will be written to another file. The output file will also include a summary of the data processed. You must use at least one dialog box in this program. The data for each triangle will be on a separate line of the input file, with the input data echoed and the results referred to by the line number (see example). On initialization, the program will prompt the user for both an input and an output file. If a non-existent input file is specified, the appropriate exception must be handled, resulting in an error message. For the exception case, re-prompt the user for the correct input file. Once the I/O file is specified, the program will read in and process all the entries in the file. The output of the program will be written to the specified output file and echoed to the console. The program will evaluate each line and determine the validity of the triangle. If valid, the program will output a summary line for the triangle calculation. If invalid, the program will output a description of the error found in the entry. Your program must be robust and handle situations where all the triangle inputs are not proper and/or present. Pathname of the input file Number of lines processed Number of valid triangles Number of invalid triangles Multiple methods Exception handing File I/O Dialog box file input: # 3 4 5 # 5 5 5 # 5 5 7 XXX # -3 10 10 15 # 0 0 0 # 3 4.1 5 # 3 x 4 # 5 4 3 6 YYY ZZZ # 1 1 10 # 15 16 22 # 3 4 output: 1: 3, 4, 5: The triangle is a right triangle, The area of this triangle is 6.00 2: 5, 5, 5: The triangle is equilateral, The area of this triangle is 10.83 3: 5, 5, 7: The triangle is isosceles, The area of this triangle is 12.50 Item discarded: XXX Error - At least one of your sides is an invalid integer value Item discarded: 15 Error - At least one of your sides is an invalid integer value Error - At least one of your sides is not an integer Error - At least one of your sides is not an integer Error - Sides not in ascending order Item discarded: 6 Item discarded: YYY Item discarded: ZZZ Error - Sides do not form a valid triangle 4: 15, 16, 22: This triangle is not distinctive, The area of this triangle is 120.00 EOF found File Path: E:\Java\Assign-05\pa5-test-01.txt --------------- Summary Report --------------- Total entries processed=10 Number of valid entries=4 Number of invalid entries=6 Percentage of valid entries=40.00% This is what I have------- import java.util.InputMismatchException; import java.util.*; import java.io.*; public class practice { public static void main(){ boolean valid = true; int side1 = 0; int side2 = 0; int side3 = 0; String side4 = ""; int count = 1; try { File myfile = new File("triangles.txt"); Scanner fileReader = new Scanner(myfile); PrintWriter writer = new PrintWriter("output.txt"); while(fileReader.hasNextInt()) { side1 = fileReader.nextInt(); side2 = fileReader.nextInt(); side3 = fileReader.nextInt(); side4 = fileReader.nextLine(); if (side1 <= 0 || side2 <= 0 || side3 <=0){ writer.println("Error - At least one of your sides is an invalid integer value"); } if (side1 > side2 || side2 > side3){ writer.println("Error - Sides not in ascending order"); } if(side1==side2 && side2==side3){ writer.println(count + ": "+ side1 + " " + side2 + " "+ side3 +" The triangle is equalateral"); writer.printf("The area of this triangle is %.2f\n", calculateArea(side1, side2, side3)); } else if((side1==side2 && side2 != side3 && side1!=side3) || (side2==side3 && side2!=side1 && side3!=side1) || (side3==side1 && side3!=side2 && side1!=side2 )) { writer.println(count + ": "+ side1 + " " + side2 + " "+ side3 +" The triangle is isoceles"); writer.printf("The area of this triangle is %.2f\n", calculateArea(side1, side2, side3)); } else if ((Math.pow(side1, 2) + Math.pow(side2, 2)) == (Math.pow(side3, 2)) || (Math.pow(side3, 2) + Math.pow(side2, 2)) == (Math.pow(side1, 2))){ writer.println(count + ": " + side1 + " " + side2 + " "+ side3 +" This triangle is a right triangle"); writer.printf("The area of this triangle is %.2f\n", calculateArea(side1, side2, side3)); } else if ((side1 != side2) && (side1 != side3) && (side2!=side3) && (side1!=side3)){ writer.println(count + ": " + side1 + " " + side2 + " "+ side3 +" This triangle is scalene"); writer.printf("The area of this triangle is %.2f\n", calculateArea(side1, side2, side3)); } else if(side4 == null){ continue; } else if(side4 != null){ writer.println("Item discarded: " + side4); } count++; } writer.close(); } catch(Exception e) { e.printStackTrace(); System.exit(-1); } } private static double calculateArea(int a,int b,int c) { double area = ((a+b+c)/ 2.0); double area1 = Math.sqrt(area*((area-a)*(area-b)*(area-c))); double area2 = Math.round(area1*100.0)/100.0; return area2; } } What am I missing?
Java only
Design, implement and test a Java class that processes a series of triangles. For this assignment, triangle data will be read from an input file and the program’s output will be written to another file. The output file will also include a summary of the data processed. You must use at least one dialog box in this program.
The data for each triangle will be on a separate line of the input file, with the input data echoed and the results referred to by the line number (see example).
On initialization, the program will prompt the user for both an input and an output file. If a non-existent input file is specified, the appropriate exception must be handled, resulting in an error message. For the exception case, re-prompt the user for the correct input file. Once the I/O file is specified, the program will read in and process all the entries in the file. The output of the program will be written to the specified output file and echoed to the console.
The program will evaluate each line and determine the validity of the triangle. If valid, the program will output a summary line for the triangle calculation. If invalid, the program will output a description of the error found in the entry. Your program must be robust and handle situations where all the triangle inputs are not proper and/or present.
- Pathname of the input file
- Number of lines processed
- Number of valid triangles
- Number of invalid triangles
- Multiple methods
- Exception handing
- File I/O
- Dialog box
file input:
# 3 4 5
# 5 5 5
# 5 5 7 XXX
# -3 10 10 15
# 0 0 0
# 3 4.1 5
# 3 x 4
# 5 4 3 6 YYY ZZZ
# 1 1 10
# 15 16 22
# 3 4
output:
1: 3, 4, 5: The triangle is a right triangle,
The area of this triangle is 6.00
2: 5, 5, 5: The triangle is equilateral,
The area of this triangle is 10.83
3: 5, 5, 7: The triangle is isosceles,
The area of this triangle is 12.50
Item discarded: XXX
Error - At least one of your sides is an invalid integer value
Item discarded: 15
Error - At least one of your sides is an invalid integer value
Error - At least one of your sides is not an integer
Error - At least one of your sides is not an integer
Error - Sides not in ascending order
Item discarded: 6
Item discarded: YYY
Item discarded: ZZZ
Error - Sides do not form a valid triangle
4: 15, 16, 22: This triangle is not distinctive,
The area of this triangle is 120.00
EOF found
File Path: E:\Java\Assign-05\pa5-test-01.txt
--------------- Summary Report ---------------
Total entries processed=10
Number of valid entries=4
Number of invalid entries=6
Percentage of valid entries=40.00%
This is what I have-------
import java.util.InputMismatchException;
import java.util.*;
import java.io.*;
public class practice
{
public static void main(){
boolean valid = true;
int side1 = 0;
int side2 = 0;
int side3 = 0;
String side4 = "";
int count = 1;
try
{
File myfile = new File("triangles.txt");
Scanner fileReader = new Scanner(myfile);
PrintWriter writer = new PrintWriter("output.txt");
while(fileReader.hasNextInt()) {
side1 = fileReader.nextInt();
side2 = fileReader.nextInt();
side3 = fileReader.nextInt();
side4 = fileReader.nextLine();
if (side1 <= 0 || side2 <= 0 || side3 <=0){
writer.println("Error - At least one of your sides is an invalid integer value");
}
if (side1 > side2 || side2 > side3){
writer.println("Error - Sides not in ascending order");
}
if(side1==side2 && side2==side3){
writer.println(count + ": "+ side1 + " " + side2 + " "+ side3 +" The triangle is equalateral");
writer.printf("The area of this triangle is %.2f\n", calculateArea(side1, side2, side3));
}
else if((side1==side2 && side2 != side3 && side1!=side3) || (side2==side3 && side2!=side1 && side3!=side1) || (side3==side1 && side3!=side2 && side1!=side2 )) {
writer.println(count + ": "+ side1 + " " + side2 + " "+ side3 +" The triangle is isoceles");
writer.printf("The area of this triangle is %.2f\n", calculateArea(side1, side2, side3));
}
else if ((Math.pow(side1, 2) + Math.pow(side2, 2)) == (Math.pow(side3, 2)) || (Math.pow(side3, 2) + Math.pow(side2, 2)) == (Math.pow(side1, 2))){
writer.println(count + ": " + side1 + " " + side2 + " "+ side3 +" This triangle is a right triangle");
writer.printf("The area of this triangle is %.2f\n", calculateArea(side1, side2, side3));
}
else if ((side1 != side2) && (side1 != side3) && (side2!=side3) && (side1!=side3)){
writer.println(count + ": " + side1 + " " + side2 + " "+ side3 +" This triangle is scalene");
writer.printf("The area of this triangle is %.2f\n", calculateArea(side1, side2, side3));
}
else if(side4 == null){
continue;
}
else if(side4 != null){
writer.println("Item discarded: " + side4);
}
count++;
}
writer.close();
}
catch(Exception e)
{
e.printStackTrace();
System.exit(-1);
}
}
private static double calculateArea(int a,int b,int c)
{
double area = ((a+b+c)/ 2.0);
double area1 = Math.sqrt(area*((area-a)*(area-b)*(area-c)));
double area2 = Math.round(area1*100.0)/100.0;
return area2;
}
}
What am I missing?

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

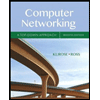
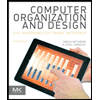
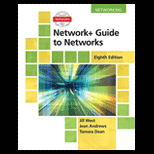
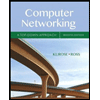
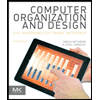
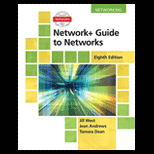
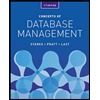
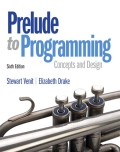
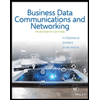