Write a JAVA program that reads the height of a triangle (less than 10) from the user and then calls two void methods named plotTril and plotTri2. Each of the 2 methods should have a parameter for the height of the triangle and use printf inside of nested For loops to produce the output of the respective triangle for the user. Note that the numbers in the triangle are left-aligned.
Write a JAVA program that reads the height of a triangle (less than 10) from the user and then calls two void methods named plotTril and plotTri2. Each of the 2 methods should have a parameter for the height of the triangle and use printf inside of nested For loops to produce the output of the respective triangle for the user. Note that the numbers in the triangle are left-aligned.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:## JAVA Triangle Program Tutorial
### Overview
This tutorial guides you through writing a JAVA program that reads the height of a triangle (less than 10) from the user. It then calls two void methods named `plotTri1` and `plotTri2`. These methods print triangles of numbers, which are left-aligned.
### Instructions
1. **Project Setup:**
- Create a project and class named `HW8FirstnameLastname` in NetBeans, replacing `Firstname` and `Lastname` with your actual name.
2. **Method Description:**
- Both `plotTri1` and `plotTri2` methods should take a parameter for the triangle's height and use `printf` inside nested For loops.
- The output should produce triangles with the specified height.
3. **Example:**
- For height = 8, the methods `plotTri1` and `plotTri2` will print the following:
#### `plotTri1` Output
```
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
1 5 10 10 5 1
1 6 15 20 15 6 1
1 7 21 35 35 21 7 1
```
#### `plotTri2` Output
```
1
2 1 2
4 2 1 2 4
8 4 2 1 2 4 8
16 8 4 2 1 2 4 8 16
32 16 8 4 2 1 2 4 8 16 32
64 32 16 8 4 2 1 2 4 8 16 32 64
128 64 32 16 8 4 2 1 2 4 8 16 32 64 128
```
### Explanation of the Triangles
- **`plotTri1`:** This triangle mirrors Pascal's Triangle, where each number is

Transcribed Image Text:**Creating Triangles Using Java in NetBeans**
In this exercise, you will create a project and class named `HW8FirstnameLastname` in NetBeans, replacing "FirstnameLastname" with your actual first and last names. The task involves programming code to display two distinct triangular patterns using numbers.
### Task Details
For a given height of 8, the functions `plotTri1` and `plotTri2` will generate and print two triangles as follows:
**Triangle Pattern 1 (`plotTri1`):**
This pattern displays Pascal's Triangle aligned to the left. Each subsequent row shows the binomial coefficients for that particular number:
```
1
1 1
1 2 1
1 3 3 1
1 4 6 4 1
1 5 10 10 5 1
1 6 15 20 15 6 1
1 7 21 35 35 21 7 1
```
**Triangle Pattern 2 (`plotTri2`):**
This pattern also shows a numerical series, similar to Pascal's Triangle, but aligned to the right:
```
1
1 1
1 2 1
1 4 6 4 1
1 8 16 32 16 8 1
1 16 32 64 128 64 32 16 1
1 32 64 128 256 256 128 64 32 1
```
### Instructions
1. **Set Up the Project:**
- Open NetBeans and create a new project.
- Name the project and class as described, replacing "FirstnameLastname" with your actual names.
2. **Implement the Plot Functions:**
- Write a method `plotTri1()` to print the first triangle using nested loops.
- Write another method `plotTri2()` to print the second triangle, ensuring proper alignment.
### Learning Outcomes
- Understand how to use loops to generate complex patterns.
- Familiarize yourself with Pascal’s Triangle and the concept of binomial coefficients.
- Gain experience in formatting output for better readability and presentation in code.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 6 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
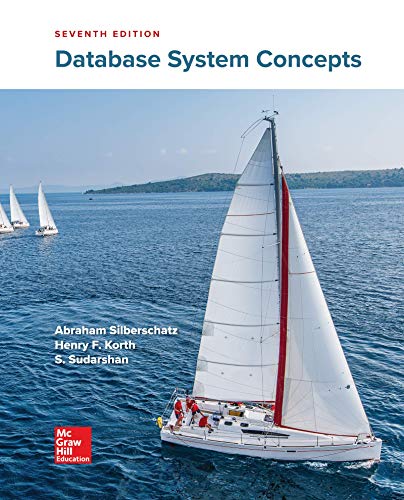
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
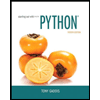
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
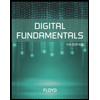
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
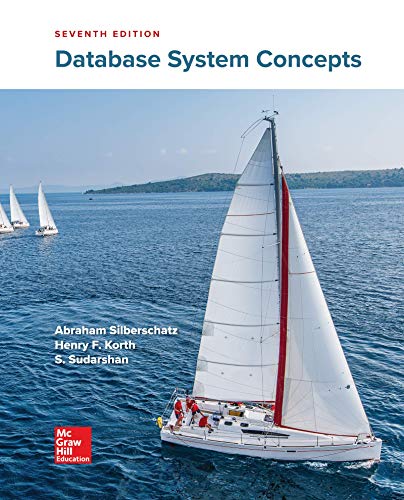
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
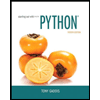
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
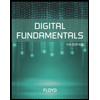
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
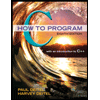
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
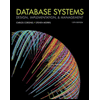
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
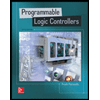
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education