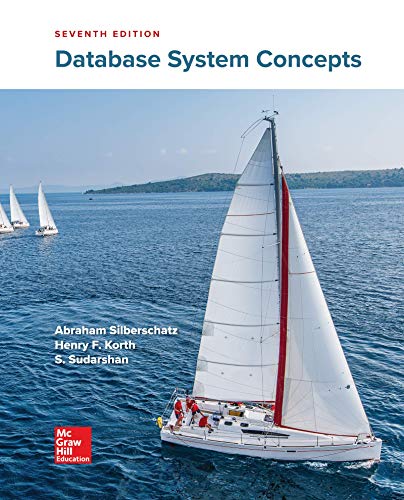
Database System Concepts
7th Edition
ISBN: 9780078022159
Author: Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher: McGraw-Hill Education
expand_more
expand_more
format_list_bulleted
Question
in java
Integer valueIn is read from input. Write a while loop that iterates until valueIn is negative. In each iteration:
- Update integer result as follows:
- If valueIn is divisible by 5, output "win" and increment result.
- Otherwise, output "lose" and do not update result.
- Then, read an integer from input into variable valueIn.
End each output with a newline.
Click here for exampleEx: If the input is 5 15 11 -5, then the output is:
win win lose Result is 2
Note: x % 5 == 0 returns true if x is divisible by 5.
import java.util.Scanner;
public class ResultCalculator {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
int valueIn;
int result;
result = 0;
valueIn = scnr.nextInt();
/your code here/
System.out.println("Result is " + result);
}
}
SAVE
AI-Generated Solution
info
AI-generated content may present inaccurate or offensive content that does not represent bartleby’s views.
Unlock instant AI solutions
Tap the button
to generate a solution
to generate a solution
Click the button to generate
a solution
a solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Similar questions
- Write a do-while loop that continues to prompt a user to enter a number less than 100, until the entered number is actually less than 100. End each prompt with newline Ex: For the user input 123, 395, 25, the expected output is: Enter a number (<100): Enter a number (<100): Enter a number (<100): Your number < 100 is: 25arrow_forwardin java Jump to level 1 The first and second integers in the input are read into variables previousNum and currentNum, respectively. Write a loop that iterates until currentNum is not equal to 6 plus previousNum. In each iteration: Output currentNum, followed by " is 6 plus ", previousNum, and ". Sequence is increasing arithmetically." End with a newline. Assign previousNum with currentNum. Read the next integer from input and assign currentNum with the integer read. After the loop, output the last integer read followed by " breaks the sequence." and a newline. Click here for example Note: Assume that input has at least two integers. 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 import java.util.Scanner; public class Sequencing { publicstaticvoidmain(String[] args) { Scannerscnr=newScanner(System.in); intcurrentNum; intpreviousNum; previousNum=scnr.nextInt(); currentNum=scnr.nextInt(); System.out.println("Sequence starts at "+previousNum+"."); /* Your code…arrow_forwardCHALLENGE ACTIVITY 5.2.3: Basic while loop expression. Write a while loop that repeats while user_num ≥ 1. In each loop iteration, divide user_num by 2, then print user_num.Sample output with input: 2010.0 5.0 2.5 1.25 0.625 Note: If the submitted code has an infinite loop, the system will stop running the code after a few seconds and report "Program end never reached." The system doesn't print the test case that caused the reported message.arrow_forward
- In Java Integer in is read from input. Write a while loop that iterates until in is less than or equal to 0. In each iteration: Update in with the quotient of in and 7. Output the updated in, followed by a newline. Click here for exampleEx: If the input is 2401, then the output is: 343 49 7 1 0 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 import java.util.Scanner; public class QuotientCalculator { publicstaticvoidmain(String[] args) { Scannerscnr=newScanner(System.in); intin; in=scnr.nextInt(); /your code here/ } }arrow_forwardsolve in java Integer numInput is read from input representing the number of integer values to be read next. Use a loop to read the remaining integer values from input. For each integer value read, output "Value read: " followed by the value. Then, output "Highest: " followed by the highest of the integer values read. End each output with a newline. Ex: If the input is: 3 220 -490 5 then the output is: Value read: 220 Value read: -490 Value read: 5 Highest: 220 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 import java.util.Scanner; public class HighestValues { publicstaticvoidmain(String[] args) { Scannerscnr=newScanner(System.in); intnumInput; intinputValue; inthighestVal=0; inti; numInput=scnr.nextInt(); /* Your code goes here */ } }arrow_forwardin java Integer numInts is read from input representing the number of integers to be read next. Use a loop to read the remaining integers from input into variable value. For each integer from 0 to numInts minus 1, inclusive, output the integer followed by " grapes". End each output with a newline. Click here for exampleEx: If the input is: 2 14 12 then the output is: 14 grapes 12 grapes 1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 import java.util.Scanner; public class NumberOfGrapes { publicstaticvoidmain(String[] args) { Scannerscnr=newScanner(System.in); intnumInts; intvalue; inti; numInts=scnr.nextInt(); /* Your code goes here */ } }arrow_forward
- PrimeAA.java Write a program that will tell a user if their number is prime or not. Your code will need to run in a loop (possibly many loops) so that the user can continue to check numbers. A prime is a number that is only divisible by itself and the number 1. This means your code should loop through each value between 1 and the number entered to see if it’s a divisor. If you only check for a small handful of numbers (such as 2, 3, and 5), you will lose most of the credit for this project. Include a try/catch to catch input mismatches and include a custom exception to catch negative values. If the user enters 0, the program should end. Not only will you tell the user if their number is prime or not, you must also print the divisors to the screen (if they exist) on the same line as shown below AND give a count of how many divisors there are. See examples below. Your program should run the test case exactly as it appears below, and should work on any other case in general. Output…arrow_forwardUsing Java, This program should print out first N powers of 2 starting from 2^1, until 2^N. The program first asks the user to enter the max power of 2, reads it using nextInt() and then uses the while loop to print out first n powers of 2. e.g. Enter the number of powers of two: 10 2^1 = 2 2^2 = 4 2^3 = 8 2^4 = 16 2^5 = 32 2^6 = 64 2^7 = 128 2^8 = 256 2^9 = 512 2^10 = 1024arrow_forwardIn Java Write a program to score the paper-rock-scissor game. Each of two users types ineither P, R, or S. The program then announces the winner as well as the basis fordetermining the winner: Paper covers rock, Rock breaks scissors, Scissors cut paper,or Nobody wins. Be sure to allow the users to use lowercase as well as uppercaseletters. Your program should include a loop that lets the user play again until theuser says she or he is done. The program should perform input validation for theplayer’s input (only ‘R’, ‘P’ or ‘S’ are acceptable).Sample Run:Player 1: Please enter either R)ock, P)aper, or S)cissors.RPlayer 2: Please enter either R)ock, P)aper, or S)cissors.SPlayer 1 wins.Totals to this move:Player 1: 1Player 2: 0Play Again? Y/y continues, other quits. YPlayer 1: Please enter either R)ock, P)aper, or S)cissors.SPlayer 2: Please enter either R)ock, P)aper, or S)cissors.PPlayer 1 wins.Totals to this move:Player 1: 2Player 2: 0Play Again? Y/y continues, other quits.…arrow_forward
- JAVA A perfect number is a positive integer that is equal to the sum of its positive divisors, excluding the number itself. A divisor of an integer x is an integer that can divide x evenly. Take an integer n as input from the keyboard, print true if n is a perfect number, otherwise, print false. You do not need to print any explanation, just print true or false. Use a for loop to generate the divisors. Example 1: Input: num = 28 Output: true Explanation: 28 = 1 + 2 + 4 + 7 + 14 1, 2, 4, 7, and 14 are all divisors of 28. Example 2: Input: num = 6 Output: true Example 3: Input: num = 496 Output: true Example 4: Input: num = 8128 Output: true Example 5: Input: num = 2 Output: falsearrow_forwardCan you please make this in Java?arrow_forwardWrite a complete program to generate a username based on a user's first and last name. Part One: Get a Valid First and Last Name ask the user for and read in their first and last name use a loop to validate the first name: it must be at least one character long and it must start with a letter use a loop to validate the last name: it must not contain any spaces Hint: use a nested loop. The outer loop will iterate until there is valid input. The inner loop will check each character in the String to see if any of them are whitespace. Part Two: Generate a Username generate a two-digit random number (i.e., a random number between 10 and 99, inclusive) create a username that is the following data concatenated: the first letter of their first name in lower case the first five characters of their last name in lower case; if the name is shorter than five characters, use the whole last name the two-digit number output the username to the user Additional Coding Requirements code…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
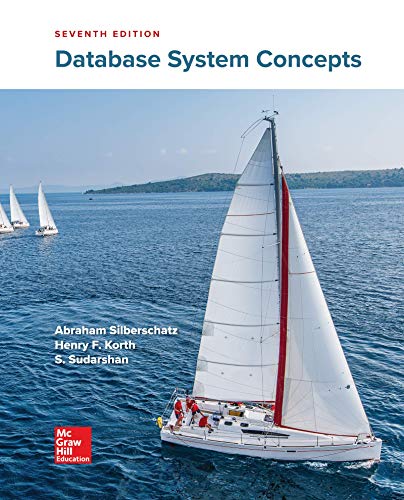
Database System Concepts
Computer Science
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:McGraw-Hill Education
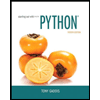
Starting Out with Python (4th Edition)
Computer Science
ISBN:9780134444321
Author:Tony Gaddis
Publisher:PEARSON
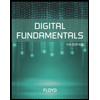
Digital Fundamentals (11th Edition)
Computer Science
ISBN:9780132737968
Author:Thomas L. Floyd
Publisher:PEARSON
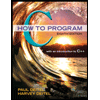
C How to Program (8th Edition)
Computer Science
ISBN:9780133976892
Author:Paul J. Deitel, Harvey Deitel
Publisher:PEARSON
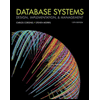
Database Systems: Design, Implementation, & Manag...
Computer Science
ISBN:9781337627900
Author:Carlos Coronel, Steven Morris
Publisher:Cengage Learning
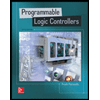
Programmable Logic Controllers
Computer Science
ISBN:9780073373843
Author:Frank D. Petruzella
Publisher:McGraw-Hill Education