write a java program that reads a single integer from standard input. Upon reading this single integer, your program should check to see if the value entered is actually an integer, if not, then print an error and exit. If it is an integer, your program should make sure that the number is actually positive single digit, if not then print another error and exit the program. After you read the number, then you should read another positive integer representing the number of lines to print. Again you should error‐check for invalid input. Once the correct single positive digit and the number of lines are entered, then the program should display the digit on the screen to start printing a pyramid of digit combinations that counts the number of each digit in the previous line. The format of each line starting with line 2 of the pyramid would be the number of times a digit showed up in the previous line, followed by a space, followed by the digit. These counts are also separated by a space. The pyramid should be formatted like a pyramid which is centered in the screen, not left justified or right justified. You should continue producing lines until one or more of the following conditions happens: 1. You have used all 9 digits in the output. So you need to keep track of which digits you used. This may not happen by the way, but check for it any way. 2. You have produced the required number of lines. 3. You produced the exact line as the one above it (see the example below). Once one or more of the above conditions stops printing the lines, then you should print a message showing which digits were used in the output. Look at the following example: This is a scenario of your program running. Please enter a positive digit: 5 Please enter the number of lines in the pyramid: 20 The following is the produced pyramid: 5 1 5 1 1 1 5 3 1 1 5 2 1 1 3 1 5 3 1 1 2 1 3 1 5 4 1 1 2 2 3 1 5 1 4 3 1 2 2 1 3 1 5 4 1 2 2 1 4 2 3 1 5 3 1 3 2 1 3 2 4 1 5 3 1 2 2 3 3 1 4 1 5 3 1 2 2 3 3 1 4 1 5 The digits 1, 2, 3, 4, and 5 were used. Your implementation must contain a main class as well as another class called Pyramid. The main program should declare and instantiate a Pyramid class and simply call 3 methods from the Pyramid class. a. A method to read the input b. A method to print the pyramid c. A method to print the digits used. So, the main program should only be 4 statements inside of the main method. All the other code that performs the needed work should be included in the class Pyramid.
write a java program that reads a single integer from standard input. Upon reading this single integer, your program should check to see if the value entered is actually an integer, if not, then print an error and exit. If it is an integer, your program should make sure that the number is actually positive single digit, if not then print another error and exit the program. After you read the number, then you should read another positive integer representing the number of lines to print. Again you should error‐check for invalid input. Once the correct single positive digit and the number of lines are entered, then the program should display the digit on the screen to start printing a pyramid of digit combinations that counts the number of each digit in the previous line. The format of each line starting with line 2 of the pyramid would be the number of times a digit showed up in the previous line, followed by a space, followed by the digit. These counts are also separated by a space. The pyramid should be formatted like a pyramid which is centered in the screen, not left justified or right justified. You should continue producing lines until one or more of the following conditions happens: 1. You have used all 9 digits in the output. So you need to keep track of which digits you used. This may not happen by the way, but check for it any way. 2. You have produced the required number of lines. 3. You produced the exact line as the one above it (see the example below). Once one or more of the above conditions stops printing the lines, then you should print a message showing which digits were used in the output. Look at the following example: This is a scenario of your program running. Please enter a positive digit: 5 Please enter the number of lines in the pyramid: 20 The following is the produced pyramid: 5 1 5 1 1 1 5 3 1 1 5 2 1 1 3 1 5 3 1 1 2 1 3 1 5 4 1 1 2 2 3 1 5 1 4 3 1 2 2 1 3 1 5 4 1 2 2 1 4 2 3 1 5 3 1 3 2 1 3 2 4 1 5 3 1 2 2 3 3 1 4 1 5 3 1 2 2 3 3 1 4 1 5 The digits 1, 2, 3, 4, and 5 were used. Your implementation must contain a main class as well as another class called Pyramid. The main program should declare and instantiate a Pyramid class and simply call 3 methods from the Pyramid class. a. A method to read the input b. A method to print the pyramid c. A method to print the digits used. So, the main program should only be 4 statements inside of the main method. All the other code that performs the needed work should be included in the class Pyramid.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

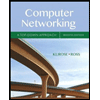
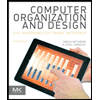
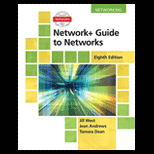
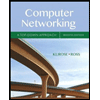
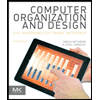
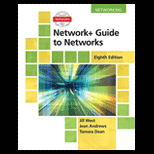
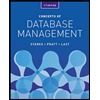
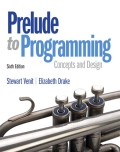
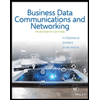