Write a Java program that includes in its class the following methods: - A method named unitMatrix which takes one parameter which is an integer (n) and - prints a unit matrix of dimension (nxn). A unit matrix is a square matrix in which the - diagonal elements are all 1s and the remaining elements are 0s. - For example for n = 3 the printed unit matrix should be: - 1 0 0 - 0 1 0 - 0 0 1 - - A method named triangularMatrix which takes two parameters. The first parameter is - an integer (n) which specifies the dimensions of the triangular matrix to be printed - (nxn). The second parameter is a boolean which specifies the type of the triangular - matrix: if its value is true, the printed matrix must be an upper triangular matrix - (square elements with all elements below the diagonal are 0s), if its value is false the - printed matrix must be a lower triangular matrix (square elements with all elements - above the diagonal are 0s). - The values of non-zero elements must start from 1 counting upwards throughout the - rows. - For example if n = 3 and the Boolean is true, the printed matrix is an upper triangular - as follows: - 1 2 3 - 0 4 5 - 0 0 6 - For example if n = 3 and the Boolean is false, the printed matrix is a lower triangular - as follows: - 1 0 0 - 2 3 0 - 4 5 6 - - In your main method, test the above two methods by calling them for different - dimensionality values. Make sure that you test printing unit matrices, upper triangular - matrices, and lower triangular matrices.
Write a Java program that includes in its class the following methods:
- A method named unitMatrix which takes one parameter which is an integer (n) and
- prints a unit matrix of dimension (nxn). A unit matrix is a square matrix in which the
- diagonal elements are all 1s and the remaining elements are 0s.
- For example for n = 3 the printed unit matrix should be:
- 1 0 0
- 0 1 0
- 0 0 1
- - A method named triangularMatrix which takes two parameters. The first parameter is
- an integer (n) which specifies the dimensions of the triangular matrix to be printed
- (nxn). The second parameter is a boolean which specifies the type of the triangular
- matrix: if its value is true, the printed matrix must be an upper triangular matrix
- (square elements with all elements below the diagonal are 0s), if its value is false the
- printed matrix must be a lower triangular matrix (square elements with all elements
- above the diagonal are 0s).
- The values of non-zero elements must start from 1 counting upwards throughout the
- rows.
- For example if n = 3 and the Boolean is true, the printed matrix is an upper triangular
- as follows:
- 1 2 3
- 0 4 5
- 0 0 6
- For example if n = 3 and the Boolean is false, the printed matrix is a lower triangular
- as follows:
- 1 0 0
- 2 3 0
- 4 5 6
- - In your main method, test the above two methods by calling them for different
- dimensionality values. Make sure that you test printing unit matrices, upper triangular
- matrices, and lower triangular matrices.
- 2. Write a Java program that includes in its class the following methods:
- - A method named duplicateSentence that takes a String and an integer, and returns a
- String that consists of duplicating the passed String number of times that equals the
- passed integer on separate lines.
- For example, if the passed String is Welcome to Java!, and the passed integer is 4, the
- returned String should be:
- Welcome to Java!
- Welcome to Java!
- Welcome to Java!
- Welcome to Java!
- - A method named calculate that takes two double numbers and a character as
- parameters. The character must be one of the four main operations +, -, *, or /. The
- method must return a String that consists of the full equation that includes the two
- numbers, the operation and the result.
- For example, if the passed double numbers are 3.5 and 2.1, and the passed operation is
- +, the returned String must be as follows:
- 3.5 + 2.1 = 5.6
- If the passed character does not represent any of the four main operations, the method
- must return the following String: “Wrong operation”.
- - In your main method, test the above two methods:
- o Print the String (Coding is fun!) 10 times by invoking the method
- duplicateSentence.
- o Declare two double variables, and invoke the method calculate and print the result
- of their addition, subtraction, multiplication, and division.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

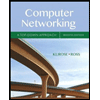
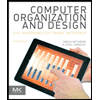
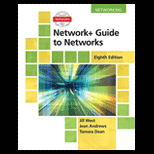
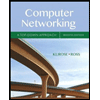
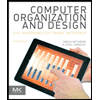
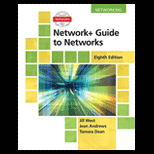
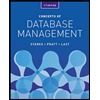
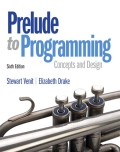
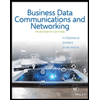