Write a Java application program (name the file as DigitReverse.java) that will get multiple non-negative integers from the keyboard, one at a time (-1 to exit). For each integer, it should print the digits in reverse order, one per line. Your program should include the main method and a method called digitExtract. You main method should allow user to enter multiple integers (non-negative integers, except for -1). The digitExtract( ) method has an integer as a parameter. The method should break up the integer into individual digits (using some simple math) and print them to the screen in reverse order, with each digit appearing on a separate line. For example: Please enter a non-negative integer (-1 to exit): 57321 Your number printed in reverse order is: 1 2 3 7 5 Please enter a non-negative integer (-1 to exit): 0 Your number printed in reverse order is: 0 Please enter a non-negative integer (-1 to exit): -1
Write a Java application program (name the file as DigitReverse.java) that will get
multiple non-negative integers from the keyboard, one at a time (-1 to exit). For each
integer, it should print the digits in reverse order, one per line. Your program should
include the main method and a method called digitExtract. You main method should
allow user to enter multiple integers (non-negative integers, except for -1). The
digitExtract( ) method has an integer as a parameter. The method should break up the
integer into individual digits (using some simple math) and print them to the screen in
reverse order, with each digit appearing on a separate line.
For example:
Please enter a non-negative integer (-1 to exit): 57321
Your number printed in reverse order is:
1
2
3
7
5
Please enter a non-negative integer (-1 to exit): 0
Your number printed in reverse order is:
0
Please enter a non-negative integer (-1 to exit): -1

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

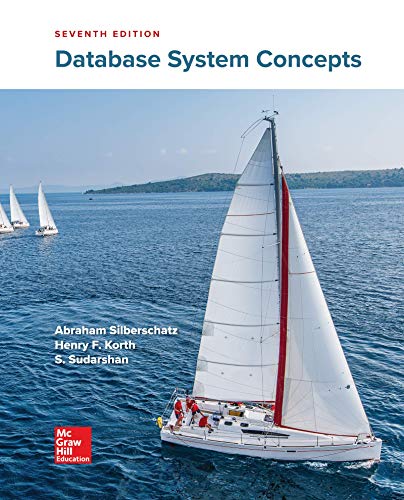
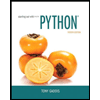
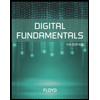
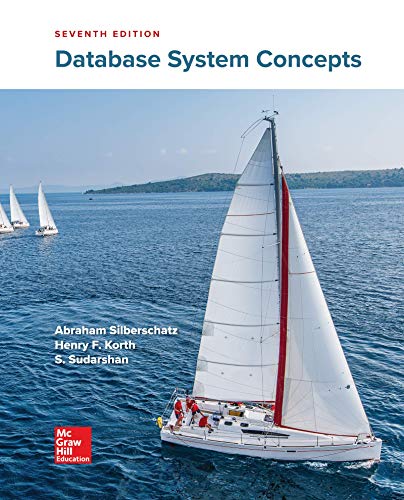
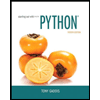
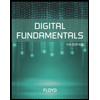
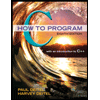
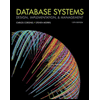
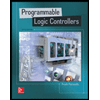