Write a function validate_date(date_string) which takes in a datestring and checks if the datestring is valid. If the string is valid, we want to return a tuple containing True and the date_string itself. If the string is invalid for a specific reason, we want to return a tuple containing False and the error code explaining what was wrong with the date string passed in. Below is the error codes for reasons why a string can be invalid (based on criteria on validity, read above): -1 --> date_string does not separate the day, month, and year with slashes. -2 --> day, month, or year are not numeric. -3 --> month out of range (not between 1 and 12) -4 --> day is out of range (not between 1 and last day of the month) For instance, if validate_date(date_string) is called with "01/12/2022" as the argument, the return value is (True, "01/12/2022") because "01/12/2022" is a valid string. If validate_date(date_string) is called with "01/44/2022" as the argument, the return value is (False, -4) because "01/44/2022" is an invalid string because the day (44) was out of range. So the error code is -4. If validate_date(date_string) is called with "January/12/2022" as the argument, the return value is (False, -2) because "January/12/2022" is an invalid string because the month is not numeric. So the error code is -2. Instructions Finish the validate_date() function Use the dictionary num_days that maps months to the number of days in that month (useful for checking if the day is in the range) You can check if the day, month, and year are separated by slashes by calling .split( "/" ) on the datestring. If the list that is returned by the .split() call is of size 3, that means there are two slashes somewhere in the string that separate the day, month, and year. If the list is not of size 3, you can be sure that datetime is invalid (Error code: -1) def validate_date(date_string): num_days = { 1: 31, 2: 28, 3: 31, 4: 30, 5: 31, 6: 30, 7: 31, 8: 31, 9: 30, 10: 31, 11: 30, 12: 31 } month = len(date_string[0:2]) days = len(date_string[3:5]) if not date_string[0].isdigit() or not date_string[1].isdigit(): return (False, -2) if not date_string[3].isdigit() or not date_string[4].isdigit(): return (False, -2) if not date_string[6].isdigit() or not date_string[7].isdigit() or not date_string[8].isdigit() or not date_string[9].isdigit(): return (False, -2) if month not in num_days: return (False, -3) if (days < 1) or days > num_days[month]: return (False, -4) else: return (True, date_string) # finish the function if __name__ == "__main__": assert validate_date('01/12/2022')[0] == True assert validate_date('01/12/2022')[1] == '01/12/2022' assert validate_date('01/44/2022')[0] == False assert validate_date('01/44/2022')[1] == -4 assert validate_date('January/12/2022')[0] == False assert validate_date('January/12/2022')[1] == -2
- Write a function validate_date(date_string) which takes in a datestring and checks if the datestring is valid. If the string is valid, we want to return a tuple containing True and the date_string itself. If the string is invalid for a specific reason, we want to return a tuple containing False and the error code explaining what was wrong with the date string passed in.
Below is the error codes for reasons why a string can be invalid (based on criteria on validity, read above):
-1 --> date_string does not separate the day, month, and year with slashes. -2 --> day, month, or year are not numeric. -3 --> month out of range (not between 1 and 12) -4 --> day is out of range (not between 1 and last day of the month)
For instance, if validate_date(date_string) is called with "01/12/2022" as the argument, the return value is
(True, "01/12/2022")
because "01/12/2022" is a valid string.
If validate_date(date_string) is called with "01/44/2022" as the argument, the return value is
(False, -4)
because "01/44/2022" is an invalid string because the day (44) was out of range. So the error code is -4.
If validate_date(date_string) is called with "January/12/2022" as the argument, the return value is
(False, -2)
because "January/12/2022" is an invalid string because the month is not numeric. So the error code is -2.
Instructions
- Finish the validate_date() function
- Use the dictionary num_days that maps months to the number of days in that month (useful for checking if the day is in the range)
- You can check if the day, month, and year are separated by slashes by calling .split( "/" ) on the datestring. If the list that is returned by the .split() call is of size 3, that means there are two slashes somewhere in the string that separate the day, month, and year. If the list is not of size 3, you can be sure that datetime is invalid (Error code: -1)
def validate_date(date_string):
num_days = {
1: 31,
2: 28,
3: 31,
4: 30,
5: 31,
6: 30,
7: 31,
8: 31,
9: 30,
10: 31,
11: 30,
12: 31
}
month = len(date_string[0:2])
days = len(date_string[3:5])
if not date_string[0].isdigit() or not date_string[1].isdigit():
return (False, -2)
if not date_string[3].isdigit() or not date_string[4].isdigit():
return (False, -2)
if not date_string[6].isdigit() or not date_string[7].isdigit() or not date_string[8].isdigit() or not date_string[9].isdigit():
return (False, -2)
if month not in num_days:
return (False, -3)
if (days < 1) or days > num_days[month]:
return (False, -4)
else:
return (True, date_string)
# finish the function
if __name__ == "__main__":
assert validate_date('01/12/2022')[0] == True
assert validate_date('01/12/2022')[1] == '01/12/2022'
assert validate_date('01/44/2022')[0] == False
assert validate_date('01/44/2022')[1] == -4
assert validate_date('January/12/2022')[0] == False
assert validate_date('January/12/2022')[1] == -2

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

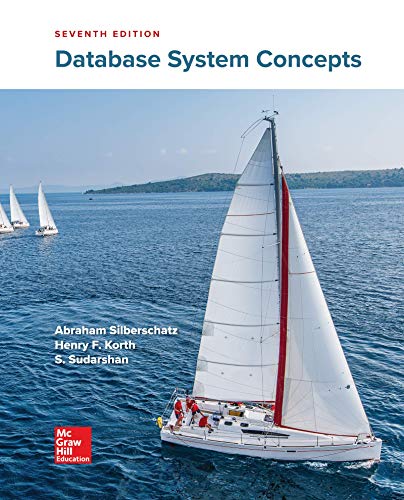
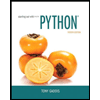
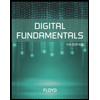
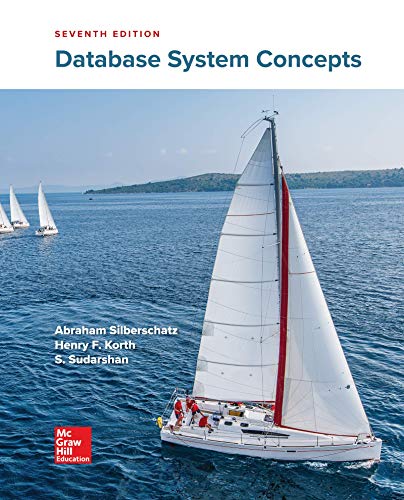
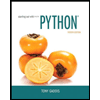
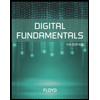
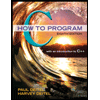
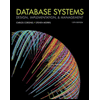
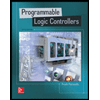