Write a function that takes two arguments: stock and ingr (short for ingredients) and returns the missing ingredients in stock. stock is a dictionary containing (key,value) pairs in the form of ("potato", 5). The key is the name of an ingredient, and the vlaue is its existing amount. ingr is a list of tuples, where each tuple represents the required amount of an ingredient. For example, ("cheese", 3) means we need 3 units of cheese for this recipe. Your function checks whether we have enough of each item in the stock. If an item is missing or not enough then your function returns the missing amount within a list of all such items. If all items are in stock, then you return an empty list. For example: stock = {"salt":100, "sugar":100, "flour":50, "apple":3, "cream":10} ingr = [("sugar",50), ("flour", 120), ("apple",5)] checkStock(stock, ingr) should return [("flour",70), ("apple",2)] which means we need 70 more units of flour and 2 more apples for this recipe. Note that it is possible for an item to not exist in the stock. """ def checkStock(stock, ingr): return # Remove this line to answer this question.
Write a function that takes two arguments: stock and ingr (short for
ingredients) and returns the missing ingredients in stock. stock is
a dictionary containing (key,value) pairs in the form of ("potato", 5).
The key is the name of an ingredient, and the vlaue is its existing
amount. ingr is a list of tuples, where each tuple represents the required
amount of an ingredient. For example, ("cheese", 3) means we need 3 units of
cheese for this recipe. Your function checks whether we have enough of each item
in the stock. If an item is missing or not enough then your function returns
the missing amount within a list of all such items. If all items are in stock, then you return an empty list. For example:
stock = {"salt":100, "sugar":100, "flour":50, "apple":3, "cream":10}
ingr = [("sugar",50), ("flour", 120), ("apple",5)]
checkStock(stock, ingr) should return
[("flour",70), ("apple",2)]
which means we need 70 more units of flour and 2 more apples for this recipe.
Note that it is possible for an item to not exist in the stock.
"""
def checkStock(stock, ingr):
return # Remove this line to answer this question.
![Question 3: (20 Points)
Write a function that takes two arguments: stock and ingr (short for
ingredients) and returns the missing ingredients in stock. stock is
a dictionary containing (key, value) pairs in the form of ("potato", 5).
The key is the name of an ingredient, and the vlaue is its existing
amount. ingr is a list of tuples, where each tuple represents the required
amount of an ingredient. For example, ("cheese", 3) means we need 3 units of
cheese for this recipe. Your function checks whether we have enough of each item
in the stock. If an item is missing or not enough then your function returns
the missing amount within a list of all such items. If all items are in stock, then you return an empty list. For example:
stock
{"salt":100, "sugar":100, "flour":50, "apple":3, "cream":10}
%3D
ingr = [("sugar",50), ("flour", 120), ("apple",5)]
%3D
checkStock(stock, ingr) should return
[("flour", 70), ("apple",2)]
which means we need 70 more units of flour and 2 more apples for this recipe.
Note that it is possible for an item to not exist in the stock.
def checkStock(stock, ingr):
return # Remove this line to answer this question.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Faf8778e6-8e46-4309-9286-75301316b41d%2Fb9171539-931a-4cf5-8c13-c54cd5fa286f%2F366ej9r_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 1 images

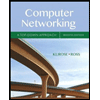
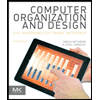
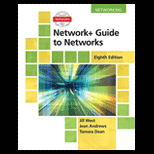
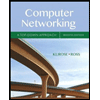
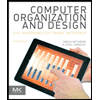
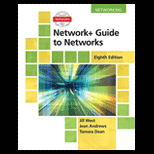
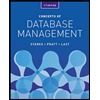
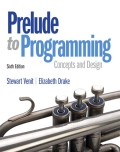
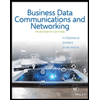