Write a FULL Java procedural program for one human player to play a “Higher or Lower” card game. In this game, each card has a value from 1..10 inclusive. There are 4 of each value in the deck, i.e., 40 cards in total. Cards are not replaced in the deck once drawn, i.e., no more than 4 of each value will be drawn. The program starts by asking the player for a target score. The game proceeds in a series of rounds with the program repeatedly drawing and showing a card from the deck to the player one at a time. Each time, it asks the player to enter "h" (higher) or "l" (lower) to guess whether the next card drawn will be higher or lower in value. The player gains a point if they guess correctly. The game continues until the player guesses incorrectly or the target score is reached. When the game ends, it prints either a "You win!" or a "Nice try, you scored …” message as illustrated below. The image provided shows two examples of the required program behaviour: (bold is keyboard input by the player): Hint: you could start by considering how to design your program while first ignoring the details of the deck (e.g., not worrying about drawing more than 4 of one value). Then extend your basic idea to include those details in some way. One option is to design an abstract data type to track the cards drawn so far during the game. However, you may employ any logical approach you wish. Your program - should use a for-loop, a while-loop, and an array (at least one of each). - must be a procedural program. (Not an object-oriented program.) - must not use global variables. All variables should be defined locally in a method.
Write a FULL Java procedural program for one human player to play a “Higher or Lower” card game. In this game, each card has a value from 1..10 inclusive. There are 4 of each value in the deck, i.e., 40 cards in total. Cards are not replaced in the deck once drawn, i.e., no more than 4 of each value will be drawn. The program starts by asking the player for a target score. The game proceeds in a series of rounds with the program repeatedly drawing and showing a card from the deck to the player one at a time. Each time, it asks the player to enter "h" (higher) or "l" (lower) to guess whether the next card drawn will be higher or lower in value. The player gains a point if they guess correctly. The game continues until the player guesses incorrectly or the target score is reached. When the game ends, it prints either a "You win!" or a "Nice try, you scored …” message as illustrated below. The image provided shows two examples of the required program behaviour: (bold is keyboard input by the player):
Hint: you could start by considering how to design your program while first ignoring the details of the deck (e.g., not worrying about drawing more than 4 of one value). Then extend your basic idea to include those details in some way. One option is to design an abstract data type to track the cards drawn so far during the game. However, you may employ any logical approach you wish. Your program - should use a for-loop, a while-loop, and an array (at least one of each). - must be a procedural program. (Not an object-oriented program.) - must not use global variables. All variables should be defined locally in a method.

![Target score? 10
I drew a 5
Round 1: Higher or lower? (h/1) 1
I drew a 4
Round 2: Higher or lower? (h/1) h
I drew a 2
Nice try, you scored 1.
Target score? 40
I drew a 10
Round 1: Higher or lower? (h/1) 1
I drew a 5
Round 2: Higher or lower? (h/1) h
[lines omitted here for space reasons]
I drew a 2
...
Round 40: Higher or lower? (h/1) h
I drew a 10
You win!](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fa959c3e2-24d7-4bac-89a8-7a621a28f6f4%2Fe81843ec-36d9-4256-998f-0b4d24f822f5%2Fnvqhffs_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 3 images

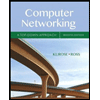
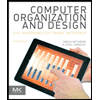
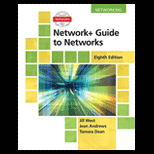
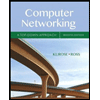
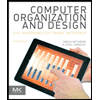
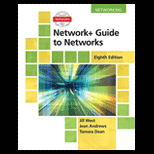
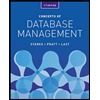
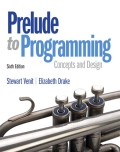
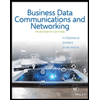