Integer numBenefits is read from input, representing the number of floating-point numbers to be read next. Then, the remaining numbers are read and stored into array benefitsArray. Write a loop that assigns array secondArray with benefitsArray's elements shifted to the left by one index. The element at index 0 of benefitsArray should be copied to the end of secondArray. Ex: If the input is: 3 390.5 240.5 320.5 then the output is: Original benefits: 390.5 240.5 320.5 Updated benefits: 240.5 320.5 390.5 1 import java.util.Scanner; 2 3 public class ArrayModification { 5 8 9 10 11 12 13 14 15 16 17 18 public static void main(String[] args) { Scanner scnr = new Scanner (System.in); double[] benefitsArray; double[] secondArray; int numBenefits; int i; numBenefits = scnr.nextInt (); benefitsArray = new double [numBenefits]; secondArray = new double [numBenefits]; for (i = 0; i < benefitsArray.length; ++i) { benefitsArray[i] = scnr.nextDouble(); }
Integer numBenefits is read from input, representing the number of floating-point numbers to be read next. Then, the remaining numbers are read and stored into array benefitsArray. Write a loop that assigns array secondArray with benefitsArray's elements shifted to the left by one index. The element at index 0 of benefitsArray should be copied to the end of secondArray. Ex: If the input is: 3 390.5 240.5 320.5 then the output is: Original benefits: 390.5 240.5 320.5 Updated benefits: 240.5 320.5 390.5 1 import java.util.Scanner; 2 3 public class ArrayModification { 5 8 9 10 11 12 13 14 15 16 17 18 public static void main(String[] args) { Scanner scnr = new Scanner (System.in); double[] benefitsArray; double[] secondArray; int numBenefits; int i; numBenefits = scnr.nextInt (); benefitsArray = new double [numBenefits]; secondArray = new double [numBenefits]; for (i = 0; i < benefitsArray.length; ++i) { benefitsArray[i] = scnr.nextDouble(); }
Chapter8: Arrays
Section: Chapter Questions
Problem 9PE
Related questions
Question
Grey lines of code (start-18 & 22-end of code) can NOT be edited.
New JAVA code must go inbetween.
![Integer numBenefits is read from input, representing the number of floating-point numbers to be read next. Then, the remaining
numbers are read and stored into array benefitsArray. Write a loop that assigns array secondArray with benefitsArray's elements
shifted to the left by one index. The element at index 0 of benefitsArray should be copied to the end of secondArray.
Ex: If the input is:
3
390.5 240.5 320.5
then the output is:
Original benefits: 390.5 240.5 320.5
Updated benefits: 240.5 320.5 390.5
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33 }
34 }
benefitsArray[i] = scnr.nextDouble();
*Your code goes here */
System.out.print ("Original benefits: ");
for (i = 0; i < benefitsArray.length; ++i) {
System.out.printf("%.1f ", benefitsArray[i]);
}
System.out.println();
System.out.print("Updated benefits: ");
for (i = 0; i < second Array.length; ++i) {
System.out.print (secondArray[i] + " ");
}
System.out.println();](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0cc153ae-b205-4fb2-9991-7cf6a21d5016%2F31472412-61ff-474d-81e2-a852060df8b8%2F8g68lfb_processed.png&w=3840&q=75)
Transcribed Image Text:Integer numBenefits is read from input, representing the number of floating-point numbers to be read next. Then, the remaining
numbers are read and stored into array benefitsArray. Write a loop that assigns array secondArray with benefitsArray's elements
shifted to the left by one index. The element at index 0 of benefitsArray should be copied to the end of secondArray.
Ex: If the input is:
3
390.5 240.5 320.5
then the output is:
Original benefits: 390.5 240.5 320.5
Updated benefits: 240.5 320.5 390.5
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33 }
34 }
benefitsArray[i] = scnr.nextDouble();
*Your code goes here */
System.out.print ("Original benefits: ");
for (i = 0; i < benefitsArray.length; ++i) {
System.out.printf("%.1f ", benefitsArray[i]);
}
System.out.println();
System.out.print("Updated benefits: ");
for (i = 0; i < second Array.length; ++i) {
System.out.print (secondArray[i] + " ");
}
System.out.println();
![Jump to level 1
Integer numBenefits is read from input, representing the number of floating-point numbers to be read next. Then, the remaining
numbers are read and stored into array benefitsArray. Write a loop that assigns array second Array with benefitsArray's elements
shifted to the left by one index. The element at index 0 of benefitsArray should be copied to the end of secondArray.
Ex: If the input is:
3
390.5 240.5 320.5
then the output is:
Original benefits: 390.5 240.5 320.5
Updated benefits: 240.5 320.5 390.5
1 import java.util.Scanner;
2
3 public class ArrayModification {
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
double[] benefitsArray;
double [] secondArray;
int numBenefits;
int i;
numBenefits = scnr.nextInt ();
benefitsArray = new double [numBenefits];
secondArray = new double [numBenefits];
for (i = 0; i < benefitsArray.length; ++i) {
benefitsArray[i] = scnr.nextDouble();](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0cc153ae-b205-4fb2-9991-7cf6a21d5016%2F31472412-61ff-474d-81e2-a852060df8b8%2Fghgkyk5_processed.png&w=3840&q=75)
Transcribed Image Text:Jump to level 1
Integer numBenefits is read from input, representing the number of floating-point numbers to be read next. Then, the remaining
numbers are read and stored into array benefitsArray. Write a loop that assigns array second Array with benefitsArray's elements
shifted to the left by one index. The element at index 0 of benefitsArray should be copied to the end of secondArray.
Ex: If the input is:
3
390.5 240.5 320.5
then the output is:
Original benefits: 390.5 240.5 320.5
Updated benefits: 240.5 320.5 390.5
1 import java.util.Scanner;
2
3 public class ArrayModification {
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
double[] benefitsArray;
double [] secondArray;
int numBenefits;
int i;
numBenefits = scnr.nextInt ();
benefitsArray = new double [numBenefits];
secondArray = new double [numBenefits];
for (i = 0; i < benefitsArray.length; ++i) {
benefitsArray[i] = scnr.nextDouble();
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
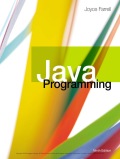
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
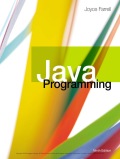
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT