Write a C++ program to do the following: Read the attached file ("TextFile.txt") and the count the number of times each alphabetic letter is used. Do not count punctuation, whitespace, or numbers. Only count letters a-z. When counting, treat lower and upper case characters the same. Use the attached CPP program as a guide to manage the counting. Use the structure and array provided to maintain counts. See examples in the program. When the all processing is completed, display the total for all letter counts. Example Output Below.: This numbers below are not intended to be accurate. No special formatting required but should be readable.
Write a C++ program to do the following:
Read the attached file ("TextFile.txt") and the count the number of times each alphabetic letter is used. Do not count punctuation, whitespace, or numbers. Only count letters a-z. When counting, treat lower and upper case characters the same.
Use the attached CPP program as a guide to manage the counting. Use the structure and array provided to maintain counts. See examples in the program.
When the all processing is completed, display the total for all letter counts.
Example Output Below.: This numbers below are not intended to be accurate. No special formatting required but should be readable.
A: 103
B: 15
C: 22
... // remaining letters
Z: 4
cpp code:
#include "stdafx.h"
#include <iostream>
using namespace std;
struct LetterCount
{
char letter;
int count;
};
// declare an array of structure
const int MAXLETTERS = 26;
LetterCount myLetterCount[MAXLETTERS];
int main()
{
// initialize - MOVE THIS TO A METHOD
for (int idx = 0; idx<MAXLETTERS; idx++) {
myLetterCount[idx].count = 0;
myLetterCount[idx].letter = 'a' + idx; // this will load a-z
}
// search and inc EXAMPLE
char letterToFind = 'a';
for (int idx = 0; idx<MAXLETTERS; idx++) {
if (myLetterCount[idx].letter == letterToFind) {
// inc the count here
}
}
// showLetterCounts - MOVE THIS TO A METHOD
for (int idx = 0; idx<MAXLETTERS; idx++) {
cout << myLetterCount[idx].letter << " " << myLetterCount[idx].count << endl;
}
}
TextFile:
"Count On Me"
I'll sail the world to find you
If you ever find yourself lost in the dark and you can't see
I'll be the light to guide you
We find out what we're made of
When we are called to help our friends in need
You can count on me
Like 1, 2, 3
I'll be there
And I know when I need it
I can count on you
Like 4, 3, 2
You'll be there
'Cause that's what friends are supposed to do
If you're tossin' and you're turnin'
And you just can't fall asleep
I'll sing a song beside you
And if you ever forget how much you really mean to me
Every day I will remind you
We find out what we're made of
When we are called to help our friends in need
You can count on me
Like 1, 2, 3
I'll be there
And I know when I need it
I can count on you
Like 4, 3, 2
You'll be there
'Cause that's what friends are supposed to do
You'll always have my shoulder when you cry
I'll never let go, never say goodbye
You know
You can count on me
Like 1, 2, 3
I'll be there
And I know when I need it
I can count on you
Like 4, 3, 2
And you'll be there
'Cause that's what friends are supposed to do
You can count on me 'cause I can count on you

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

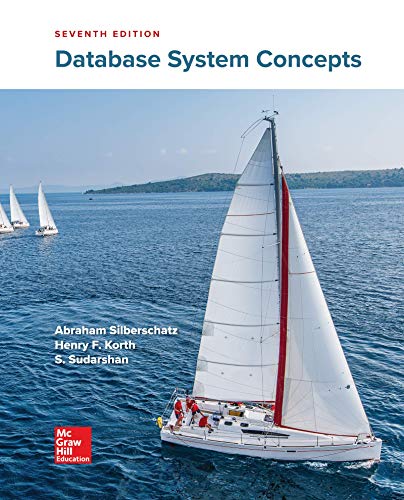
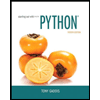
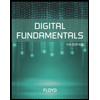
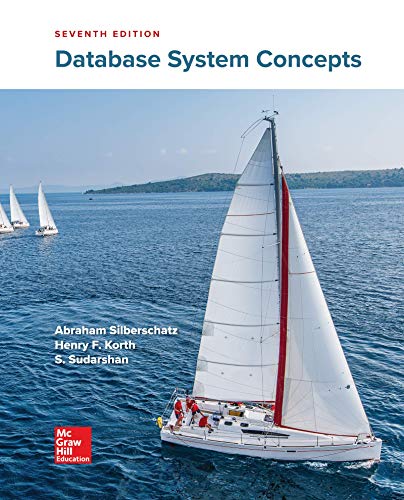
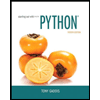
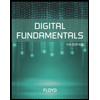
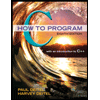
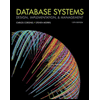
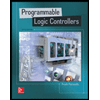