Write a C program that gets user input as a string and determines if the given string is a palindrome (the same when spelt forwards or backwards). Examples of palindromes (ignoring case, punctuation and spaces) are: civic Race car Madam, I’m Adam. A man, a plan, a canal, Panama. We need to check if a word or phrase that may contain uppercase letters, lowercase letters, spaces, and punctuation marks. For example, race car is not a palindrome because 'e' is not the same as ' ' and Racecar is not a palindrome because 'R' is not the same as 'r'. To solve this problem, write a function lettersOnlyLower that, given a string phrase, converts all letters to lowercase and removes all spaces and non-letters. To find out if the given word or phrase is a palindrome, write a function called palindrome, which, given a string word, returns 1 if word is a palindrome and 0 if it is not. Note that if the user hit enter without typing any word or phrase, exit the program. Use the following example code below to write your code. lettersOnlyLower function uses two char pointers as its arguments and palindrome function uses a char pointer. So, pass your values accordingly. #include #include #include void lettersOnlyLower(char *phrase, char *word); int palindrome(char *word); int main(int argc, char* argv[]) { char aPhrase[100], aWord[100]; }
Write a C program that gets user input as a string and determines if the given string is a
palindrome (the same when spelt forwards or backwards). Examples of palindromes
(ignoring case, punctuation and spaces) are:
civic
Race car
Madam, I’m Adam.
A man, a plan, a canal, Panama.
We need to check if a word or phrase that may contain uppercase letters, lowercase
letters, spaces, and punctuation marks. For example, race car is not a palindrome
because 'e' is not the same as ' ' and Racecar is not a palindrome because 'R' is not the
same as 'r'. To solve this problem, write a function lettersOnlyLower that, given a
string phrase, converts all letters to lowercase and removes all spaces and non-letters.
To find out if the given word or phrase is a palindrome, write a function called
palindrome, which, given a string word, returns 1 if word is a palindrome and 0 if it is
not.
Note that if the user hit enter without typing any word or phrase, exit the program.
Use the following example code below to write your code. lettersOnlyLower function uses
two char pointers as its arguments and palindrome function uses a char pointer. So,
pass your values accordingly.
#include <stdio.h>
#include <string.h>
#include <ctype.h>
void lettersOnlyLower(char *phrase, char *word);
int palindrome(char *word);
int main(int argc, char* argv[]) {
char aPhrase[100], aWord[100];
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 4 images

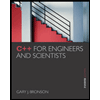
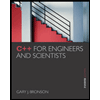