I have the following code but with the input file studentInfo.txt that has the input: ed18, Edward Duran, 077, 3.5 abc1234MikePeterson, 123, 3.7 dw0,Danika Wilson, 083 , 3.9 wd50,WillDavidson, 090,3 hpj4332, Helen Jordan1983.88 xd222XavierDavies1983.65 ah1 Allisson Henson 147 2.9 ne099 NinaEstrada, 095, 2 sh5555 Skylar Huff 112 3.3 def reformat_student_info(filename): try: #Function to split the name into first and last parts def split_name(name): parts = name.split() if len(parts) == 2: return parts else: #If no space assume last name starts after first letter return [parts[0], parts[1:]] #Function to format GPA def format_gpa(gpa): #Ensure exactly one decimal point if '.' not in gpa: gpa = gpa + '.0' return gpa #Open input file with open(filename, 'r') as input_file: lines = input_file.readlines() #Open output file for writing with open('studentInfoReformatted.csv', 'w') as output_file: for line in lines: line = line.strip() #Remove unnecessary spaces and newlines #Split line into netID, name, major, and GPA parts = line.split(',') if len(parts) == 4: netID, name, major, gpa = parts netID = netID.strip() name = name.strip() major = major.strip() gpa = gpa.strip() #Format the name and GPA first_name, last_name = split_name(name) gpa = format_gpa(gpa) #Write reformatted data to output file output_file.write(f"{netID}, {first_name} {last_name}, {major}, {gpa}\n") except: raise NotImplementedError() I am getting the output: ed18 Edward Duran 077 3.5 ed18 Edward Duran 077 3.5 dw0 Danika Wilson 083 3.9 wd50 WillDavidson [] 090 3.0 wd50 WillDavidson [] 090 3.0 wd50 WillDavidson [] 090 3.0 wd50 WillDavidson [] 090 3.0 wd50 WillDavidson [] 090 3.0 wd50 WillDavidson [] 090 3.0 Note: the rest of the names and info is not outputting to the studentInfoReformatted.csv
I have the following code but with the input file studentInfo.txt that has the input:
ed18, Edward Duran, 077, 3.5
abc1234MikePeterson, 123, 3.7
dw0,Danika Wilson, 083 , 3.9
wd50,WillDavidson, 090,3
hpj4332, Helen Jordan1983.88
xd222XavierDavies1983.65
ah1 Allisson Henson 147 2.9
ne099 NinaEstrada, 095, 2
sh5555 Skylar Huff 112 3.3
def reformat_student_info(filename):
try:
#Function to split the name into first and last parts
def split_name(name):
parts = name.split()
if len(parts) == 2:
return parts
else:
#If no space assume last name starts after first letter
return [parts[0], parts[1:]]
#Function to format GPA
def format_gpa(gpa):
#Ensure exactly one decimal point
if '.' not in gpa:
gpa = gpa + '.0'
return gpa
#Open input file
with open(filename, 'r') as input_file:
lines = input_file.readlines()
#Open output file for writing
with open('studentInfoReformatted.csv', 'w') as output_file:
for line in lines:
line = line.strip() #Remove unnecessary spaces and newlines
#Split line into netID, name, major, and GPA
parts = line.split(',')
if len(parts) == 4:
netID, name, major, gpa = parts
netID = netID.strip()
name = name.strip()
major = major.strip()
gpa = gpa.strip()
#Format the name and GPA
first_name, last_name = split_name(name)
gpa = format_gpa(gpa)
#Write reformatted data to output file
output_file.write(f"{netID}, {first_name} {last_name}, {major}, {gpa}\n")
except:
raise NotImplementedError()
I am getting the output:

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

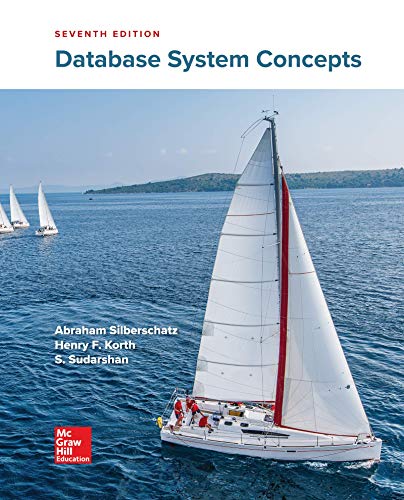
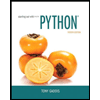
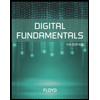
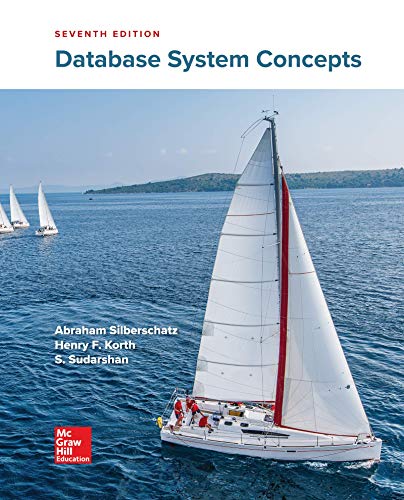
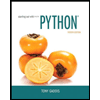
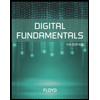
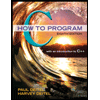
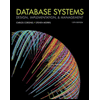
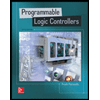