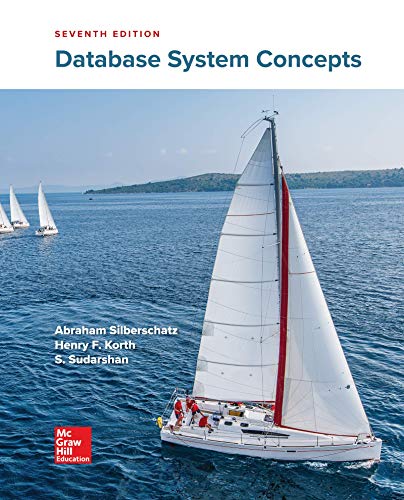
Write a C++
In main, begin by declaring variables and displaying the class header, and cout an explanation of the program. You may include this in your header or make it separate.
You will need constants for the maximum color number and for the maximum picture number as well as a value that indicates the user has finished drawing.
These will look like:
//Constants
const int QUIT{ 6};
const int MAX_COLORS{ 6};
const int MAX_PIX{3};
At this point, create a handle to the standard output device (the console) using:
HANDLE screen = GetStdHandle(STD_OUTPUT_HANDLE);
You will use this handle to access the screen to change colors. You will also need to #include <windows.h>.
Open a do-while loop. This is the “play loop.” Provide a menu of colors and ask the user to select a color for the drawing. There will be 6 menu items, 5 colors, blue, green, cyan, red and purple. These will be selections 1-5. Selection 6 is to quit the drawing loop.
Use a while loop to check to make sure that the user’s answer is in the correct range of value, 1 – 6. If it is not, loop back and ask the user to re-enter their choice.
As long as the selection is not 6, present another menu and ask the user to select the picture to be drawn. There will be 3 pictures, a 1) smiling face, 2) a pyramid and 3) a picture of your choice. Check the input validity of that selection using a do while loop.
Use a switch structure to process the picture selection. Declare any variables you will use in the drawings up above the switch statement.
In each case statement, set the color using:
SetConsoleTextAttribute(screen, colorChoice);
Be sure to adjust the colorChoice value so that you will be drawing with the bright version of the color. It shows up so much better! Each picture will be drawn using the color selected by the user. Each picture can be drawn using symbols of your choice.
When the user selects 6 for the color selection, drop out of the loop, and show a good-bye message.

C++ program:
#include <iostream>
#include <windows.h>
// Constants
const int QUIT{6};
const int MAX_COLORS{6};
const int MAX_PIX{3};
// Function prototypes
void showMenuColors();
void showMenuPix();
void drawSmilingFace(HANDLE screen, int colorChoice);
void drawPyramid(HANDLE screen, int colorChoice);
void drawMyChoice(HANDLE screen, int colorChoice);
int main()
{
// Declare variables
int colorChoice, pictureChoice;
HANDLE screen = GetStdHandle(STD_OUTPUT_HANDLE);
// Display program header and explanation
std::cout << "CIS247A Week 3 Lab B\n"
<< "Programmer: YOUR NAME\n"
<< "This program allows the user to select a color and a shape to draw.\n"
<< "The user can select from 5 colors (blue, green, cyan, red, and purple)\n"
<< "and 3 shapes (smiling face, pyramid, and a picture of your choice).\n\n";
// Play loop
do
{
// Show menu of colors
showMenuColors();
// Get color choice from user
std::cout << "Enter your color choice (1-6): ";
std::cin >> colorChoice;
// Check if color choice is valid
while (colorChoice < 1 || colorChoice > MAX_COLORS)
{
std::cout << "Invalid choice. Enter your color choice (1-6): ";
std::cin >> colorChoice;
}
// Quit if color choice is 6
if (colorChoice == QUIT)
{
break;
}
// Show menu of pictures
showMenuPix();
// Get picture choice from user
std::cout << "Enter your picture choice (1-3): ";
std::cin >> pictureChoice;
// Check if picture choice is valid
while (pictureChoice < 1 || pictureChoice > MAX_PIX)
{
std::cout << "Invalid choice. Enter your picture choice (1-3): ";
std::cin >> pictureChoice;
}
// Process picture choice
switch (pictureChoice )
{
case 1:
drawSmilingFace(screen, colorChoice);
break;
case 2:
drawPyramid(screen, colorChoice);
break;
case 3:
drawMyChoice(screen, colorChoice);
break;
}
} while (colorChoice != QUIT);
// Display goodbye message
std::cout << "Goodbye!
Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 2 images

- in C# Write a program named InputMethodDemo2 that eliminates the repetitive code in the InputMethod() in the InputMethodDemo program in Figure 8-5. Rewrite the program so the InputMethod() contains only two statements: one = DataEntry("first");two = DataEntry("second"); I am getting the error Method DataEntry is defined to eliminate repetitive code 0 out of 1 checks passed. unit Test Incomplete Method DataEntry prompts the user to enter an integer and returns the integer Build Status Build Failed Build Output Compilation failed: 1 error(s), 0 warnings NtTest37b77fb0.cs(21,47): error CS0234: The type or namespace name `DataEntry' does not exist in the namespace `InputMethodDemo2'. Are you missing an assembly reference? Test Contents [TestFixture] public class DataEntryMethodTest { [Test ] public void DataEntryTest() { string consoleInput = "97"; int returnedValue; string expectedString = "Enter third integer"; using (var inputs = new StringReader(consoleInput)) {…arrow_forwardAnalyze the following code: int x = 0;int y = ((x < 100) && (x > 0)) ? 1: -1; The code has syntax error. y becomes 1 after the code is executed. y becomes -1 after the code is executed. The code has run time error.arrow_forwardSummary 2 HouseSign.py - This program calculates prices for cu house signs. In this lab, you complete a prewritten Python program for a carpenter who creates personalized house signs. The program is supposed to compute the price of any sign a customer orders, based 4 on the following facts: 5 # Declare and initialize variables here. 6 # Charge for this sign. • The charge for all signs is a minimum of $35.00. # Number of characters. 8 # Color of characters. • The first five letters or numbers are included in the minimum charge; there is a $4 charge for 9 # Type of wood. 10 each additional character. 11 # Write assignment and if statements here as appropr • If the sign is make of oak, add $20.00. No charge is added for pine. 12 13 # Output Charge for this sign. • Black or white characters are included in the minimum charge; there is an additional $15 14 print("The charge for this sign is $" + str(charge)) charge for gold-leaf lettering. 15 Instructions 1. Make sure the file HouseSign.py…arrow_forward
- Please submit a flowchart of your program for your project below. Need a class which will contain: Student Name Student Id Student Grades (an array of 3 grades) A constructor that clears the student data (use -1 for unset grades) Get functions for items a, b, and c, average, and letter grade Set functions for items a, n, and c Note that the get and set functions for Student grades need an argument for the grade index. Need another class which will contain: An Array of Students (1 above) A count of number of students in use You need to create a menu interface that allows you to: Add new students Enter test grades Display all the students with their names, ids, test grades, average, and letter grade Exit the program Add comments and use proper indentation. Nice Features: I would like that system to accept a student with no grades, then later add one or more grades, and when all grades are entered, calculate the final average or grade. I would like the system to display the…arrow_forwardIn python Add the following four methods to your Crew class: move(self, location): This takes in a location as a string, along with self, and attempts to move the crew member to the specified location. If location is one of the five valid location options ("Bridge", "Medbay", "Engine", "Lasers", or "Sleep Pods"), then this should change self.location to that new value. Otherwise, the function should print out the message: Not a valid location. repair(self, ship): first_aid(self, ship): fire_lasers(self, ship, target_ship, target_location): The above three methods represent tasks that a basic Crew member is not capable of (but one of its derived classes will be able to accomplish). So each of them should simply print out a message of the form: <Name> doesn't know how to do that. Examples: Copy the following if __name__ == "__main__" block into your hw12.py file, and comment out tests for parts of the class you haven’t implemented yet. if __name__ == '__main__': crew1…arrow_forwardIn C++ Programming, write a program that calculates and prints the monthly paycheck for an employee. The net pay is calculated after taking the following deductions: Federal Income Tax: 15% State Tax: 3.5% Social Security Tax: 5.75% Medicare/Medicaid Tax: 2.75% Pension Plan: 5% Health Insurance: $75.00 Your program should prompt the user to input the gross amount and the employee name. The output will be stored in a file. Format your output to have two decimal places. A sample output follows: Bill Robinson Gross Amount: .......................... $3575.00 Federal Tax: ................................ $ 536.25 State Tax: .....................................$ 125.13 Social Security Tax: ................... $ 205.56 Medicare/Medicaid Tax: ......... $ 98.31 Pension Plan: .............................. $ 178.75 Health Insurance: .......................$ 75.00 Net Pay: ....................................... $2356.00 NOTE: The first column is left-justified, and the right column is…arrow_forward
- IN C# PLEASE Create class Cube. The class has attributes length and width and depth, each of which defaults to 1. It has read-only properties that calculate the Perimeter and the Area for a side of the cube, and another that calculates the cubed feet for the cube. (You will have to research how to do that calculation online) It has properties for length and width and depth. The set accessors should verify that length and width and depth are all floating-point numbers greater than 0.0 and less than 20.0. Write an app to test class Cube by creating 3 cubes of different sizes.arrow_forwardX609: Magic Date A magic date is one when written in the following format, the month times the date equals the year e.g. 6/10/60. Write code that figures out if a user entered date is a magic date. The dates must be between 1 - 31, inclusive and the months between 1 - 12, inclusive. Let the user know whether they entered a magic date. If the input parameters are not valid, return false. Examples: magicDate(6, 10, 60) -> true magicDate(50, 12, 600) –> falsearrow_forwardC++ program Write a method that displays information about a movie. The method accepts the movie title, running time in minutes and the release year as arguments. Provide a default value for the running time so that if you call the method without minutes, it defaults to 90. Write a main() method that demonstrates you can call the method with two or three arguments. Save the file as Movie.cpparrow_forward
- MONTY HALL LAB write this code in python and use # to answers questions - You are only allowed to use the following functions/methods: print(), range(), len(), sum(), max(), min(), and .append(). It is not mandatory to useall of these functions/methods. In this lab, you will write a code that simulates the Monty Hall Game Show. Thegame host gives the participant the choice of selecting one of three doors. Twodoors has a goat behind them and one door has a prize. The set of choices arerandomized each round. The participant needs to select the door with the prizebehind it. When the participant selects a door, the game host reveals a door with agoat behind it. The game host opens a door (different from the one selected by theparticipant) that has a goat behind it. The participant is then given the option tochange their choice. When you run your code, the code would display a message prompting the user toinput their door choice, labelled as 1, 2, and 3. Then the code will display a…arrow_forwardFor c++. Need help designing class, no namespsace. FoodWastageRecord NOTE: You need to design this class. It represents each food wastage entry recorded by the user through the form on the webpage (frontend). If you notice the form on the webpage, you’ll see that each FoodWastageRecord will have the following as the data members aka member variables. Date (as string) Meal (as string) Food name (as string) Quantity in ounces (as double) Wastage reason (as string) Disposal mechanism (as string) Cost (as double) Each member variable comes with its accessor/mutator functions. --------------------------------------------------------------------------------- FoodWastageReport NOTE: You need to design this class. It represents the report generated on the basis of the records entered by the user. This class will be constructed with all the records entered by the user as a parameter. It will then apply the logic to go over all the records and compute the following: Names of most commonly…arrow_forwardIt is not sufficient to declare a variable by using "type" alone. A variable may be identified by the data type it uses as well as other properties. The next obvious step is to figure out how to utilize this concept to describe any particular variable?arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
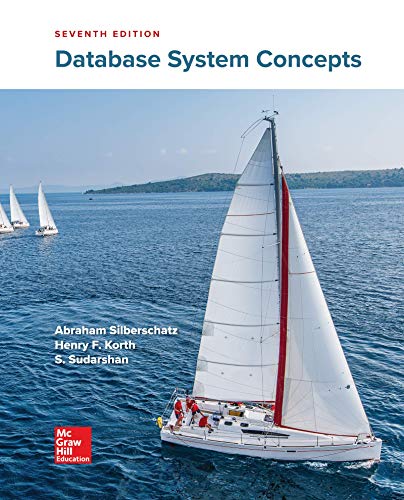
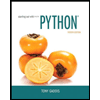
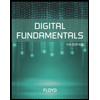
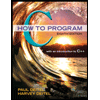
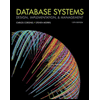
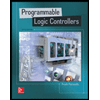