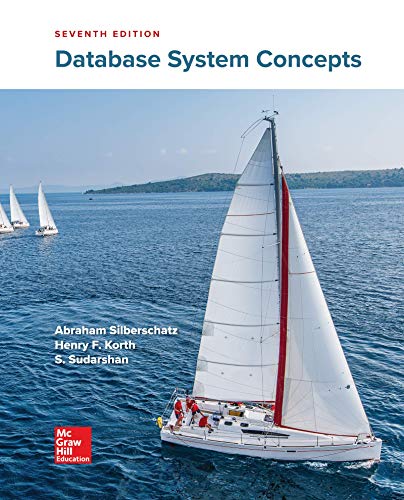
Concept explainers
Write a C# program StudentGradeDemo that attempts to create several valid and invalid ReportCard objects. Immediately after each instantiation attempt, handle any thrown exceptions by displaying an error message. Create a ReportCard class with four fields for a student name, a numeric midterm grade, a numeric final exam grade, and letter grade. The ReportCard constructor requires values for the name and two numeric grades and determines the letter grade. Upon construction, throw an ArgumentException if the midterm or final exam grade is less than 0 or more than 100. The letter grade is based on the arithmetic average of the midterm and final exams using a grading scale of A for an average of 90 to 100, B for 80 to 90, C for 70 to 80, D for 60 to 70, and F for an average below 60. Display all the data if the instantiation is successful.
Use the Main() method to test your code.

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 1 images

- Write a Temperature class to hold the data, partially listed below, in a named tuple. The class should contain a constructor and a Python internal function to convert a class to a string. Temperature.csv file excerpt: Year, Median,Upper,Lower 1850,-0.373,-0.339,-0.425 1851,-0.218,-0.184,-0.274 1852,-0.228,-0.196,-0.28 1853,-0.269,-0.239.-0.321u 1854,-0.248,-0.218,-0.301 1855,-0.272,-0.241,-0.324 1856,-0.358,-0.327,-0.413 1857,-0.461,-0.431,-0.512 1858,-0.467,-0.435,-0.521 1859,-0.284,-0.249,-0.34 1860,-0.343,-0.31,-0.405arrow_forwardWritten in Python It should have an init method that takes two values and uses them to initialize the data members. It should have a get_age method. Docstrings for modules, functions, classes, and methodsarrow_forwardPlease!!arrow_forward
- in visual c# (windows form app) no console or Console.WriteLine Design a non-static method that can be contained within an... Design a non-static method that can be contained within an instance/object of the MyCallback delegate type. This method should display values of two data fields contained within the object that is passed to the method. Make-up your own method definition that meets these requirements.arrow_forwardIn C++ programarrow_forwardCreate the VisualCounter class, which supports both increment and decrement actions. Take the function Object() { [native code] }'s two arguments N and max, where N specifies the maximum action number and max specifies the counter's maximum absolute value. Make a plot that displays the worth of the counter each time its tally changes as a byproduct.arrow_forward
- 5. Declare a class called coordinate. This is to represent 3 dimensional Cartesian coordinates (x, y and z).Define the following methods cumulatively in Java cumulatively:a. Constructorb. Display, to print values of membersc. Add_coordinates, to add three such coordinate objects to produce a resultant coordinate object.Generate and handle exceptions if x, y and z coordinate of the result are zero.d. Main, to show use of the above methods.arrow_forwardWhich of the following, can be done, with the help of the this reference?arrow_forward// Declare data fields: a String named customerName, // an int named numItems, and // a double named totalCost.// Your code here... // Implement the default contructor.// Set the value of customerName to "no name"// and use zero for the other data fields.// Your code here... // Implement the overloaded constructor that// passes new values to all data fields.// Your code here... // Implement method getTotalCost to return the totalCost.// Your code here... // Implement method buyItem.//// Adds itemCost to the total cost and increments// (adds 1 to) the number of items in the cart.//// Parameter: a double itemCost indicating the cost of the item.public void buyItem(double itemCost){// Your code here... }// Implement method applyCoupon.//// Apply a coupon to the total cost of the cart.// - Normal coupon: the unit discount is subtracted ONCE// from the total cost.// - Bonus coupon: the unit discount is subtracted TWICE// from the total cost.// - HOWEVER, a bonus coupon only applies if the…arrow_forward
- In this lab, you create a derived class from a base class, and then use the derived class in a C++ program. The program should create two Motorcycle objects, and then set the Motorcycle’s speed, accelerate the Motorcycle object, and check its sidecar status. Instructions Ensure the file named Motorcycle.cpp is open in your editor. Create the Motorcycle class by deriving it from the Vehicle class. Use a public derivation. In the Motorcycle class, create a private attribute named sidecar. The sidecar attribute should be data type bool. Write a public set method to set the value for sidecar. Write a public get method to retrieve the value of sidecar. Write a public accelerate() method. This method overrides the accelerate() method inherited from the Vehicle class. Change the message in the accelerate() method so the following is displayed when the Motorcycle tries to accelerate beyond its maximum speed: "This motorcycle cannot go that fast". Open the file named…arrow_forwardIn C++ Please: The class and functions need to be on an .h file while the main needs to be small and on a seperate .cpp file. Create a class AccessPoint with the following attributes: x - a double representing the x coordinate y - a double representing the y coordinate range - an integer representing the coverage radius status - On or Off Add constructors. The default constructor should create an access point object at position (0.0, 0.0), coverage radius 0, and Off. Add accessor and mutator functions: getX, getY, getRange, getStatus, setX, setY, setRange and setStatus. Also, add a set function that sets the location coordinates and the range. Add the following member functions: move and coverageArea. Add a function overlap that checks if two access points overlap their coverage and returns true if they overlap. Add a function signalStrength that returns the wireless signal strength as a percentage. The signal strength decreases as one moves away from the access point location. Test…arrow_forwardCreate a C# program named ConferencesDemo for a hotel that hosts business conferences. Allows a user to enter data about five Conference objects and then displays them in order of attendance from smallest to largest. The Conference class contains fields for the following: group - The group name (as a string) date - The starting date (as a string) attendees - The number of attendees (as an int) Include properties for each field. Also, include an IComparable.CompareTo() method so that Conference objects can be sorted in order from least to greatest attendees. Your output for each conference should match the following: NAME Conference starts on DATE and has ATTENDEES attendeesarrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
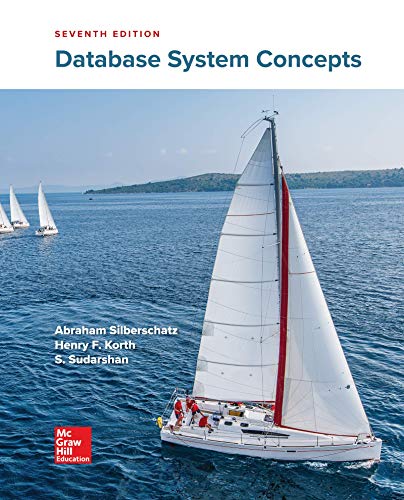
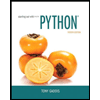
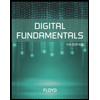
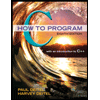
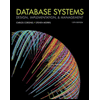
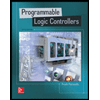