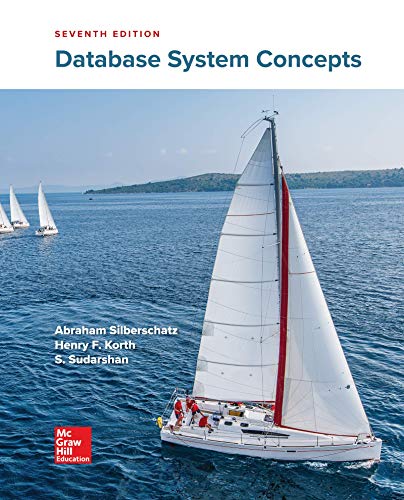
Concept explainers
Write a C program mexp that multiplies a square matrix by itself a specified number of times. mexp takes a single argument, which is the path to a file containing a square (k × k) matrix M and a non-negative exponent n. It computes Mn and prints the result.
Note that the size of the matrix is not known statically. You must use malloc to allocate space for the matrix once you obtain its size from the input file.
To compute Mn , it is sufficient to multiply M by itself n −1 times. That is, M3 = M ×M ×M . Naturally, a different strategy is needed for M0 .
Input format The first line of the input file contains an integer k. This indicates the size of the matrix M , which has k rows and k columns.
The next k lines in the input file contain k integers. These indicate the content of M. Each line corresponds to a row, beginning with the first (top) row.
The final line contains an integer n. This indicates the number of times M will be multiplied by itself. n is guaranteed to be non-negative, but it may be 0.
For example, an input file file.txt containing
3
1 2 3
4 5 6
7 8 9
2
indicates that mexp must compute
|1 2 3|2
|4 5 6|
|7 8 9|
.
Output format The output of mexp is the computed matrix M n. Each row of M nis printed on a separate line, beginning with the first (top) row. The items within a row are separated by spaces.
Using file.txt from above,
$ ./mexp file1.txt
30 36 42
66 81 96
102 126 150
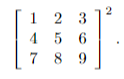

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 1 images

- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
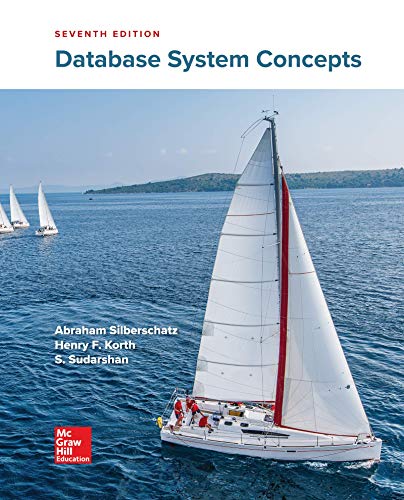
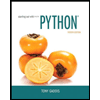
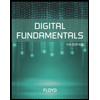
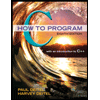
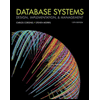
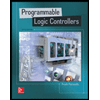