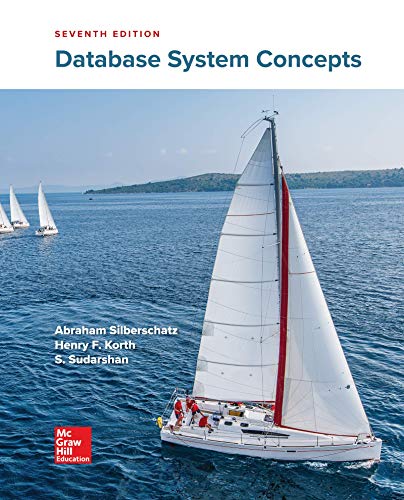
Concept explainers
With a single line of code create binned variables with 4 equal width bins for the variables 'UnsecLines', 'Age', and 'MonthlyIncome'Set. Use lambda function within the apply() function.

Step by stepSolved in 3 steps

the loop part is erroring now, it says ' Categoricals can only be compared if 'categories' are the same.' How do I fix?
I keep getting error saying 'Input array must be 1 dimensional'. What do I do to fix?
Using a for loop print frequency distribution for each of the binned variables from above. Make sure the bins in the frequency distribution are properly sorted
the loop part is erroring now, it says ' Categoricals can only be compared if 'categories' are the same.' How do I fix?
I keep getting error saying 'Input array must be 1 dimensional'. What do I do to fix?
Using a for loop print frequency distribution for each of the binned variables from above. Make sure the bins in the frequency distribution are properly sorted
- Inside this module, import random and blackout_utils, and define the following global variable, which you should use whenever appropriate: OPERATIONS = ['^', 'x', '/', '+', '-']For full marks, all the following functions must be part of this module: calculate(tokens): Takes one list of tokens as input corresponding to a mathematical equation. The function will evaluate both sides of the equality and return a list of three tokens: the result of the left-hand side (integer), the equals sign (string), and the result of the right-hand side (integer). The equation may contain any of the operations in the OPERATIONS list, as well as opening and closing parentheses. Your function should evaluate both sides of the equation while respecting order of operations (that is, parentheses, followed by exponentiation, then multiplication and division, and then addition and subtraction). Equations may have multiple sets of parentheses and nested parentheses. The contents of the inner-most nested…arrow_forwardFor Assignment 5, you will design and write a program that covers the following topics: Functions, Vectors, Structures, and Classes. Functions & Passing Variables: Write a different function for each type of variable used. The functions should provide some sort of output through a cout or return value. Normal variable: Passing a variable by value to a paramater of a function. Show that this only makes a copy of the variable passed to it. Normal variable with a default value: Set a default value for a function parameter. Show how the default value gets used. Reference variable: Passing a variable by reference to a parameter of a function. Show how a reference variable can change the value of variable in main(). Show how the use of const can make a reference variable safer (think of it in terms of read/write). Pointer variable: Passing an address of a variable to a parameter of a function. Show how a pointer can change the value of a variable in main(). Show…arrow_forward0110 earlier_name Complete the following function according to its docstring description. 1 def earlier_name(name1: str, name2: str) -> str: 2. *"Return the name, name1 or name2, that comes first alphabetically. >>> earlier_name ('Jen', 'Paul') 4 'Jen' >> earlier_name('Colin', 'Colin') 7 "Colin' Submit History LOarrow_forward
- C++ Create a function that will read the following .txt file contents and put them into an array: "apple", "avocado", "apricot","banana", "blackberry", "blueberry", "boysenberry","cherry", "cantaloupe", "cranberry", "coconut", "cucumber", "currant","date", "durian", "dragonfruit","elderberry",arrow_forwardTemplate functions may also have reference parameters. Write the function template consume which reads a value from standard input, and returns the value. Complete the following file: consumer.h 1 #ifndef CONSUMER_H 2 #define CONSUMER_H 3 4 #include 5 6 // Add your function template here 7 8 Submit } #endif Use the following file: Demo.cpp #include #include #include #include using namespace std; #include "consumer.h" int main() { int a; cout << consume (a) * 2 << endl; double b; cout << sqrt(consume (b)) << endl; string s; cout << consume(s).size() << endl;arrow_forwardUsing C++ Language In this program you will write a function of void return type named compare that accepts an integer parameter named guess. This function will compare the value of guess with a seeded randomly generated integer between 1 to 100, inclusive, and let the user know what the random number was as well as whether the guess was larger than, smaller than or equal to the random number. NOTE THAT: You will NOT generate a random number inside the compare function. Rather, you will write another function named getRandom of int return type to do it. You will need to call getRandom from compare, no parameters are necessary. Inside your main function, get the guess from user and pass it to the compare function. Code: #include <iostream>using namespace std; int getRandom(){ //generate seeded random num. //in between 1-100 inclusive //return random number } void compare(int guess){ //call getRandom //compare guess with random number //inform user what…arrow_forward
- If the number of items bought is less than 5, then the shipping charges are $7.00 for each item bought; if the number of items bought is at least 5, but less than 10, then the shipping charges are $3.00 for each item bought; if the number of items bought is at least 10, there are no shipping charges. Correct the following code so that it computes the correct shipping charges. if (numOfItemsBought > 10)shippingCharges = 0.0;else if (5 <= numOfItemsBought || numOfItemsBought <= 10);shippingCharges = 3.00 * numOfItemsBought;else if (0 < numOfItemsBought || numOfItemsBought < 5)shippingCharges = 7.00 * numOfItemsBought;arrow_forward3. Which among the following shows a valid use of the Direction enumeration as a parameter to the moveCharacter function? Select al that apply. enum Direction { case north, south, west, east}func moveCharacter(x: Int, y: Int, facing: Direction) {// code here} moveCharacter(x: 0, y: 0, facing: .southwest) moveCharacter(x: 0, y: 0, facing: Direction.north) moveCharacter(x: 0, y: 0, facing: .south) moveCharacter(x: 0, y: 0, facing: Direction.northeast)arrow_forwardPlease use the R language to answer the following questionsarrow_forward
- JQuery loading Consider following code $(function(){ // jQuery methods go here... }); What is the purpose of this code with respect to JQuery ? O This is to prevent any jQuery code from running before the document is finished loading (is ready). O This is to load the jQuery and to run this as soon as page start before loading. This is to load the function so we can run jQuery codearrow_forwardProgram: final.py Specification First, download the template file, final.py. This file contains the function names and documentation. You are required to add a header comment block with your name, the file name, the current semester, and year. Do not change the function headers, just add your implementation for each function where indicated. Add descriptive comments to your code. Remember to indent all the code for the function. Here are the functions that you will write: • Calculating tips is a common operation and the first function you write for the final project will do exactly that. The function calculate_tip accepts two parameters, the bill amount and the percent to tip, and returns the amount of the tip to write into the bill. The function should return the string "Invalid charge amount" if the charge is not more that 0 and "Invalid tip percent" if the tip is less than 0. Function heading: def calculate tip(charge, tip_percent): Parameter Specification: charge is the total ch…arrow_forwardGame of Hunt in C++ language Create the 'Game of Hunt'. The computer ‘hides’ the treasure at a random location in a 10x10 matrix. The user guesses the location by entering a row and column values. The game ends when the user locates the treasure or the treasure value is less than or equal to zero. Guesses in the wrong location will provide clues such as a compass direction or number of squares horizontally or vertically to the treasure. Using the random number generator, display one of the following in the board where the player made their guess: U# Treasure is up ‘#’ on the vertical axis (where # represents an integer number). D# Treasure is down ‘#’ on the vertical axis (where # represents an integer number) || Treasure is in this row, not up or down from the guess location. -> Treasure is to the right. <- Treasure is to the left. -- Treasure is in the same column, not left or right. +$ Adds $50 to treasure and no $50 turn loss. -$ Subtracts…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
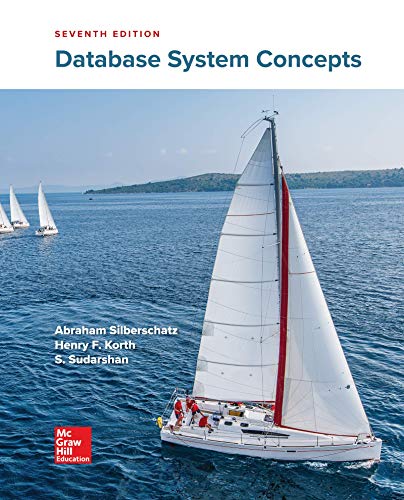
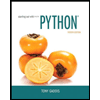
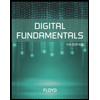
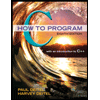
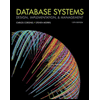
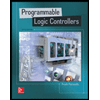