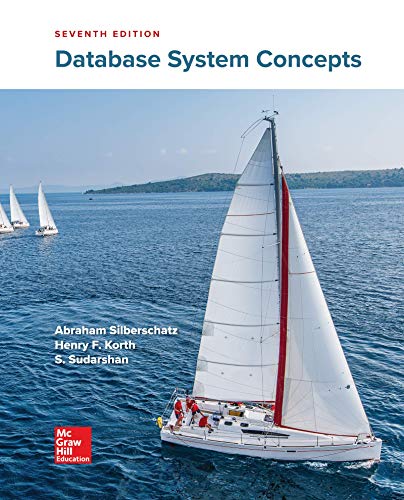
Using C++ Language
In this program you will write a function of void return type named compare that
accepts an integer parameter named guess. This function will compare the value of
guess with a seeded randomly generated integer between 1 to 100, inclusive, and let
the user know what the random number was as well as whether the guess was larger
than, smaller than or equal to the random number.
NOTE THAT: You will NOT generate a random number inside the compare function.
Rather, you will write another function named getRandom of int return type to do it.
You will need to call getRandom from compare, no parameters are necessary.
Inside your main function, get the guess from user and pass it to the compare
function.
Code:
#include <iostream>
using namespace std;
int getRandom()
{
//generate seeded random num.
//in between 1-100 inclusive
//return random number
}
void compare(int guess)
{
//call getRandom
//compare guess with random number
//inform user what random num was
//was it greater than, equal to, or less than guess?
}
int main()
{
int guess;
//get value of guess from user
//call compare and pass guess as parameter
return 0;
}

Trending nowThis is a popular solution!
Step by stepSolved in 3 steps with 2 images

- An automated donation machine accepts donations via 10 or 20 bills to a maximum of $990. The machine needs to print a receipt with the donation amount in English, rather than numerically. Complete the following function which converts any number between 10 to 990 dollars to English.NOTE: You may define and use another function if necessary.NOTE: You should use arrays for this task. Use of switch statements or very long if/else if statements for printing is not allowed. However, using if/else if/else statements for the logic of your algorithm is permitted.NOTE: This function returns the result, and does not print it. Make sure the result is not lost when you return. Example function input and output (donation is 110):Input: 110Output: “one hundred ten dollars” string convertToEnglish (int donation); in C++ #include <iostream>#include <string>using namespace std; string convertToEnglish (int donation); int main() {int donation;cin>>donation;…arrow_forwardCorrect and detailed answer will be Upvoted else downvoted. Also Explain why other options are FALSE. Thank you!arrow_forwardThank you soo much!arrow_forward
- An automated donation machine accepts donations via 10 or 20 bills to a maximum of $990. The machine needs to print a receipt with the donation amount in English, rather than numerically. Complete the following function which converts any number between 10 to 990 dollars to English.NOTE: You may define and use another function if necessary.NOTE: You should use arrays for this task. Use of switch statements or very long if/else if statements for printing is not allowed. However, using if/else if/else statements for the logic of your algorithm is permitted.NOTE: This function returns the result, and does not print it. Make sure the result is not lost when you return. Example function input and output (donation is 110): in C++arrow_forwardIn C++, Write a function named that accepts argc and args with the same data type as command line arguments (int argc, char * args[]). It will return two boolean values. It checks whether each of command line argument ending with a comma (‘,’) or not. If they all are, it will return true. Otherwise, it returns false. It will also return true if there is no argument is given. In addition, it also returns another boolean flag indicating whether one of the arguments is just a comma only.For this question, you are not allowed to use string class and its method or string function such as strlen.arrow_forwardPlease help with the assignment! Language is C. Answer as simply as you can as it is an Intro to C class. Write two functions, total_product and total_sum. Both functions return an integer. Total_product function takes in a 2D array of integers along with an integer with the number of rows and columns. This function will return an integer with the product of the sum of the rows. See example for details. The total_sum function takes in an array of integers with the size of the array. The total_sum function will find the sum of the 1D array. You must call the total_sum function in the total_product to receive full credit. Example: row/column 0 1 2 3 total_sum(row) Result 0 1 2 3 4 10 1 5 6 7 8 26 Given this 2D array above, the answer for total_product would be 260. Because row one adds up to be 10, and row two adds up to be 26. We multiple this together to get total product.arrow_forward
- In C++, please and thank you!arrow_forwardIn C++, a reference variable is a variable type that refers to another variable. Example: “int& maxValRef” declares a reference to a variable of type int.arrow_forwardIn C++ only Create a function called MultyDieRoll. It should take in an integer and run the function DieRoll that number of times, printing out each result to the console. So for instance, if you pass in a 7, it should output 7 random numbers; if you pass in a 21, it should output 21 random numbers.arrow_forward
- You are given a function called is_prime which consumes a positive number and returns True if the number is prime and False if the number is not prime. You do not need to write the is_prime function - it is already pre-defined. Your task is to use the Design Recipe to write a main function, that consumes no parameters, which prompts the user for a positive integer, n, and displays the first n primes. Your main function should call the is_prime function. Include a docstring!arrow_forwardAn automated donation machine accepts donations via 10 or 20 bills to a maximum of $990. The machine needs to print a receipt with the donation amount in English, rather than numerically. Complete the following function which converts any number between 10 to 990 dollars to English.NOTE: You may define and use another function if necessary.NOTE: You should use arrays for this task. Use of switch statements or very long if/else if statements for printing is not allowed. However, using if/else if/else statements for the logic of your algorithm is permitted.NOTE: This function returns the result, and does not print it. Make sure the result is not lost when you return. Example function input and output (donation is 110):Input: 110Output: “one hundred ten dollars” string convertToEnglish (int donation); in C++ #include <iostream>#include <string>using namespace std; string convertToEnglish (int donation); int main() {int donation;cin>>donation;…arrow_forwardIn C++, When an array is passed to a function as a pointer, the function doesn't know the size of the array. List 3 ways to handle this problem.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
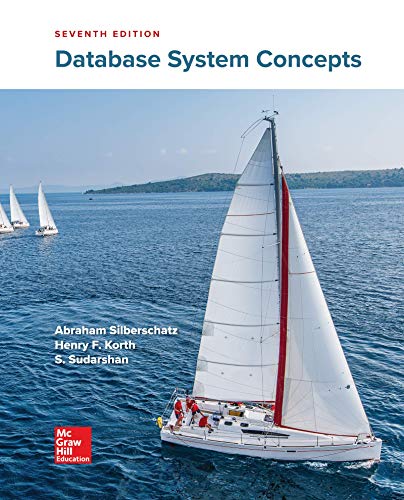
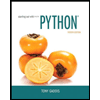
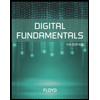
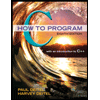
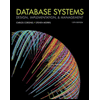
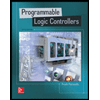