What will be the output if the input is 0? Revise line 27 in Listing 5.11 so that the program displays hex number 0 if the input decimal is 0. import java.util.Scanner; public class Dec2Hex { /** Main method */ public static void main(String[] args){ //Create a Scanner Scanner input = new Scanner(System.in); //Prompt the user to enter a decimal integer System.out.print("Enter a decimal number: "); int decimal = input.nextInt(); //Convert decimal to hex String hex = ""; while (decimal != 0){ int hexValue = decimal % 16; // Convert a decimal value to a hex digit char hexDigit = (0 <= hexValue && hexValue <= 9) ? (char) (hexValue + '0') : (char) (hexValue - 10 + 'A'); hex = hexDigit + hex; decimal = decimal / 16; } System.out.println("The hex number is " + hex); } }
What will be the output if the input is 0? Revise line 27 in Listing 5.11 so that the
import java.util.Scanner;
public class Dec2Hex {
/** Main method */
public static void main(String[] args){
//Create a Scanner
Scanner input = new Scanner(System.in);
//Prompt the user to enter a decimal integer
System.out.print("Enter a decimal number: ");
int decimal = input.nextInt();
//Convert decimal to hex
String hex = "";
while (decimal != 0){
int hexValue = decimal % 16;
// Convert a decimal value to a hex digit
char hexDigit = (0 <= hexValue && hexValue <= 9) ?
(char) (hexValue + '0') : (char) (hexValue - 10 + 'A');
hex = hexDigit + hex;
decimal = decimal / 16;
}
System.out.println("The hex number is " + hex);
}
}
![Listing 5.11 Dec2Hex.java
1 import java.util.Scanner;
3 public class Dec2Hex {
4 /** Main method */
5 public static void main (String[] args) {
6 7/ Create a Scanner
7 Scanner input
= new Scanner (System.in);
%3D
8
9 // Prompt the user to enter a decimal integer
10 System.out.print ("Enter a decimal number: ");
11 int decimal = input.nextInt();
12
13 // Convert decimal to hex
14 String hex = "";
%3|
15
16 while (decimal != 0) {
%3D
17 int hexValue
decimal % 16;
18
19 // Convert a decimal value to a hex digit
(0 <= hexValue && hexValue <= 9) ?
20 char hexDigit
21 (char) (hexValue + '0') : (char) (hexValue
%3D
10 + 'A');
22
hexDigit + hex;
decimal / 16;
23 hex
24 decimal
25 }
26
27 System.out.println ("The hex number is
28 }
29 }
+ hex);](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3d0b0572-8d36-4468-a5b0-740b909e03c0%2F25912942-29e7-499f-a4e2-2ade7006dc02%2Fc161qvq_processed.jpeg&w=3840&q=75)

Step by step
Solved in 4 steps with 2 images

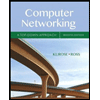
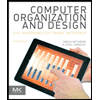
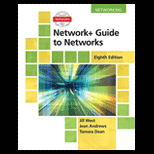
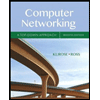
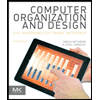
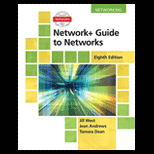
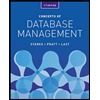
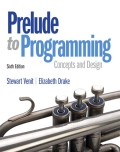
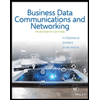