what is the problem here /* * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package testcylinder; /** * * @author imtia */ public class TestCylinder { /** * @param args the command line arguments */ public static void main(String[] args) { // TODO code application logic here int r = 43; int h = 16; Cylinder c1 = new Cylinder(r,h); Cylinder c2 = new Cylinder(r,h); System.out.println("Cylinder-1: "+c1.getVolume()); System.out.println("Cylinder-2: "+c2.getVolume()); maze(c1,c2); System.out.println("After Update :"); System.out.println("Cylinder-1: "+c1.getVolume()); System.out.println("Cylinder-2: "+c2.getVolume()); } public static void maze(Cylinder c1, Cylinder c2) { c1.height = c2.height/2; c2.height = c2.height/3; c1 = c2; c1.radius = 10; c2 = new Cylinder (10,10); } } public class Cylinder { int radius, height; public Cylinder(int radius, int height){ this.radius = radius; this.height = height; } public int getVolume(){ return (int)(3.14*radius*radius*height); } }
what is the problem here
/*
* To change this license header, choose License Headers in Project Properties.
* To change this template file, choose Tools | Templates
* and open the template in the editor.
*/
package testcylinder;
/**
*
* @author imtia
*/
public class TestCylinder {
/**
* @param args the command line arguments
*/
public static void main(String[] args) {
// TODO code application logic here
int r = 43;
int h = 16;
Cylinder c1 = new Cylinder(r,h);
Cylinder c2 = new Cylinder(r,h);
System.out.println("Cylinder-1: "+c1.getVolume());
System.out.println("Cylinder-2: "+c2.getVolume());
maze(c1,c2);
System.out.println("After Update :");
System.out.println("Cylinder-1: "+c1.getVolume());
System.out.println("Cylinder-2: "+c2.getVolume());
}
public static void maze(Cylinder c1, Cylinder c2)
{
c1.height = c2.height/2;
c2.height = c2.height/3;
c1 = c2;
c1.radius = 10;
c2 = new Cylinder (10,10);
}
}
public class Cylinder {
int radius, height;
public Cylinder(int radius, int height){
this.radius = radius;
this.height = height;
}
public int getVolume(){
return (int)(3.14*radius*radius*height);
}
}

Step by step
Solved in 3 steps with 1 images

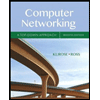
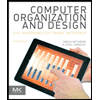
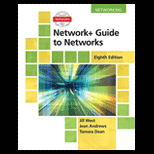
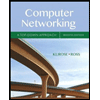
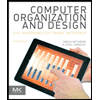
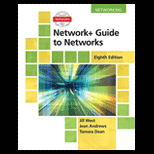
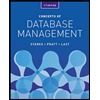
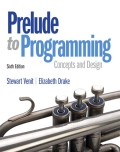
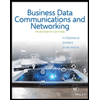