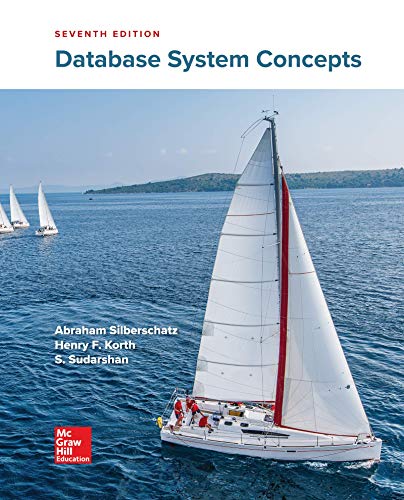
Concept explainers
![What does the following code output?
#include <iostream>
void pointerUpd (double* upt);
int main() {
double* pp;
double b[6] = { 1.01, 2.02, 3.03, 4.04, 5.05 };
pp = & (b[4]);
double *dp = pp;
dp++;
std::cout « *dp << std::endl;
std::cout <« *pp « std::endl;
pointerUpd (pp);
std::cout <« *dp << std::endl;
std::cout << *pp << std::endl;
void pointerUpd (double* upt) {
* (++upt) = 6.06;
upt = new double;
*upt = 1.01;](https://content.bartleby.com/qna-images/question/0dc061a5-6ce8-4788-a9b1-d5cfea3c8a74/c8b4480e-eea9-4159-8b2b-c1658c0b1359/1zjqza_thumbnail.png)

The C++ code given in the problem:
#include <iostream>
void pointerUpd(double* upt);
int main()
{
double* pp;
double b[6]={1.01,2.02,3.03,4.04,5.05};
pp=&(b[4]);
double *dp=pp;
dp++;
std::cout<< *dp <<std::endl;
std::cout<< *pp <<std::endl;
pointerUpd(pp);
std::cout<< *dp <<std::endl;
std::cout<< *pp <<std::endl;
}
void pointerUpd(double* upt){
*(++upt)=6.06;
upt=new double;
*upt=1.01;
}
From the code given in the problem:
pp is a pointer of type double.
b is an array having capacity 6 but 5 elements are present
pp is storing the address of the last element of the array i.e., (b[4]=5.05).
dp is another pointer of type double which pointing to pp.
dp is incremented and as dp is pointing to pp and pp was storing the last element of the array.
So, dp is now pointing to the sixth element or b[5] which by default stores the value of 0 as it was empty.
So, *dp=b[5]=0
and *pp=b[4]=5.05.
pointerUpd function is called and pointer pp is passed as parameter.
By using pointerUpd function, the last value of the array i.e., b[5] is changed to 6.06.
As dp is pointing to b[5] and pp is pointing to b[4] so after the execution of the pointerUpd function,
*dp=b[5]=6.06
and *pp=b[4]=5.05.
Step by stepSolved in 3 steps with 2 images

- #include <iostream> using namespace std; void insertElement(int* LA, int ITEM, int N, int K){ int J=N; //initialize j to n while(J>=K) //enters loop only if j less than or equal to k { LA[J+1]=LA[J]; //stores current value in next index J=J-1; //decrement j } LA[K]=ITEM; //insert element at index k N=N+1; //increment n by 1 //this is just used for printing and you can ignore if not required for(int i=0;i<N;i++) //i from 0 to n { cout << LA[i] << " "; //print element at index i }} int main(){ int LA[6]={1,2,3,4,5}; //declare an array and initialize values to it insertElement(LA,6,5,3); //call function to insert 6 at index 3 return 0;}note: Remove Funcationarrow_forward#include <iostream>using namespace std;double median(int *, int);int get_mode(int *, int);int *create_array(int);void getinfo(int *, int);void sort(int [], int);double average(int *, int);int getrange(int *,int);int main(){ int *dyn_array; int students; int mode,i,range;float avrg;do{cout << "How many students will you enter? ";cin >> students;}while ( students <= 0 );dyn_array = create_array( students );getinfo(dyn_array, students);cout<<"\nThe array is:\n";for(i=0;i<students;i++)cout<<"student "<<i+1<<" saw "<<*(dyn_array+i)<<" movies.\n";sort(dyn_array, students);cout << "\nthe median is "<<median(dyn_array, students) << endl;cout << "the average is "<<average(dyn_array, students) << endl;mode = get_mode(dyn_array, students);if (mode == -1)cout << "no mode.\n";elsecout << "The mode is " << mode << endl;cout<<"The range of movies seen is…arrow_forwardC++ Given code is #pragma once#include <iostream>#include "ourvector.h"using namespace std; ourvector<int> intersect(ourvector<int> &v1, ourvector<int> &v2) { // TO DO: write this function return {};}arrow_forward
- Write a user defined function named as “length_list” function that finds the length of a list of list_node_t nodes. This code should fit with the following code.Partial Code: #include <stdio.h> #include <stdlib.h> /* gives access to malloc */ #define SENT -1 typedef struct list_node_s { int digit; struct list_node_s *restp; } list_node_t; list_node_t *get_list(void); int main(void) { list_node_t *x;int y; x=get_list(); printf("%d\n",x->digit); printf("%d\n",x->restp->digit); printf("%d\n",x->restp->restp->digit); // your length_list function should be called from here printf("Length is: %d",y); } /* * Forms a linked list of an input list of integers * terminated by SENT */ list_node_t * get_list(void) { int data; list_node_t *ansp; [20]scanf("%d", &data); if (data == SENT) { ansp = NULL; } else { ansp = (list_node_t *)malloc(sizeof (list_node_t)); ansp->digit = data; ansp->restp = get_list(); } return (ansp);arrow_forward#include #include using namespace std; //function prototypesint countLetters(char*); int countDigits(char*); int countWhiteSpace(char*); int main() { int numLetters, numDigits, numWhiteSpace; char inputString[51]; cout <<"Enter a string of no more than 50 characters: " << endl << endl; cin.getline(inputString,51); numLetters = countLetters(inputString); numDigits = countDigits(inputString); numWhiteSpace = countWhiteSpace(inputString); cout << "The number of letters in the entered string is " << numLetters << endl; cout << "The number of digits in the entered string is " << numDigits << endl; cout << "The number of white spaces in the entered string is " << numWhiteSpace << endl; return 0; } int countLetters(char *strPtr) { int occurs = 0; while(*strPtr != '\0') // loop is executed as long as// the pointer strPtr does not point // to the null character which// marks the end of the string // isalpha determines…arrow_forward#include <bits/stdc++.h>using namespace std;int main() { double matrix[4][3]={{2.5,3.2,6.0},{5.5, 7.5, 12.6},{11.25, 16.85, 13.45},{8.75, 35.65, 19.45}}; cout<<"Input no in first row of matrix"<<endl; for(int i=0;i<3;i++){ double t; cin>>t; matrix[0][i]=t; } cout<<"Contents of the last column in matrix"<<endl; for(int i=0;i<4;i++){ cout<<matrix[i][2]<<" "; } cout<<"Content of first row and last column element in matrix is: "<<matrix[0][3]<<endl; matrix[3][2]=13.6; cout<<"Updated matrix is :"<<endl; for(int i=0;i<4;i++){ for(int j=0;j<3;j++){ cout<<matrix[i][j]<<" "; }cout<<endl; } return 0;} Please explain this codearrow_forward
- compute z= y-√2, #include <iostream>#include <cmath>#include <ios>#include <iomanip>using namespace std; int main() {double x;double y;double z; cin >> x;cin >> y; /* Your code goes here */ cout << fixed << setprecision(2); // This will output only 2 decimal places.cout << z << endl; return 0;}arrow_forward1. What is the output of the following code segment: char* s [] = { "NBY", "SDT"}; const char** z = s; const cout << z [1] [1]; cout << cout << a. DSDT b. DTY c. DTBY d. DTS e. *(z [1]+ 2); (*z + 1); None of the abovearrow_forwardcomplete the //TODOs #include<iostream> #include "swap.h" using namespace std; int main(int argc, char const *argv[]) { intfoo[5] = {1, 2, 3, 4, 5}; cout<<"Addresses of elements:"<<endl; //TODO Print the addresses of array elements cout<<endl; cout<<"Elements:"<<endl;; //TODO Print all the elements using pointers cout<<endl; inta,b; intf; intflag = 1; while(flag == 1){ cout<<"Enter indices of elements you want to swap?"<<endl; cout<<"First index"<<endl; cin>>a; cout<<"Second index"<<endl; cin>>b; cout<<"Enter 0 for pass-by-value, 1 for pass-by-pointer"<<endl; cin>>f; switch (f) { case0: // Pass by Value // Compare the resultant array with the array you get after passing by pointer cout<<"Before swapping"<<endl; for(inti = 0;i<5;i++){ cout<<foo[i]<<" "; } cout<<endl; swap(foo[a],foo[b]); cout<<"\nAfter swapping"<<endl;…arrow_forward
- #include <iostream> #include <string> #include <cstdlib> using namespace std; template<class T> class A { public: T func(T a, T b){ return a/b; } }; int main(int argc, char const *argv[]) { A <float>a1; cout<<a1.func(3,2)<<endl; cout<<a1.func(3.0,2.0)<<endl; return 0; } Give output for this code.arrow_forwardWhat does the following code output? tinclude void pointerUpd (double* upt); int main() { double* pp; double b[6] = { 1.01, 2.02, 3.03, 4.04, 5.05 }; PP = 6 (b[4]); double *dp = pp; dp++; std::cout <« *dp < std::endl; std::cout « *pPp « std::endl; pointerUpd (pp) ; std::cout « *dp « std::endl; std::cout <« *pp << std::endl; void pointerUpd (double* upt) { * (++upt) = 6.06; upt = new double; *upt = 1.01;arrow_forward#include <iostream> #include <string> #include <cstdlib> using namespace std; template<class T> T func(T a) { cout<<a; return a; } template<class U> void func(U a) { cout<<a; } int main(int argc, char const *argv[]) { int a = 5; int b = func(a); return 0; } Give output for this code.arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
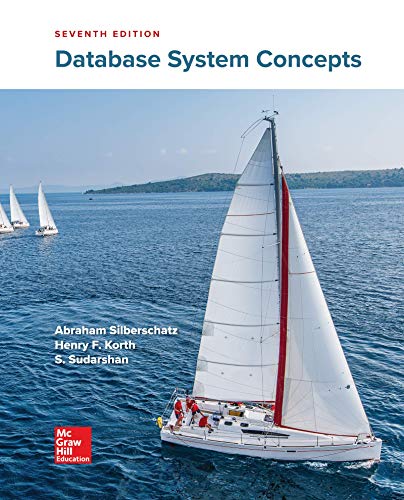
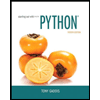
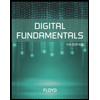
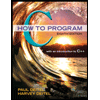
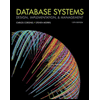
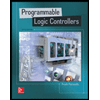