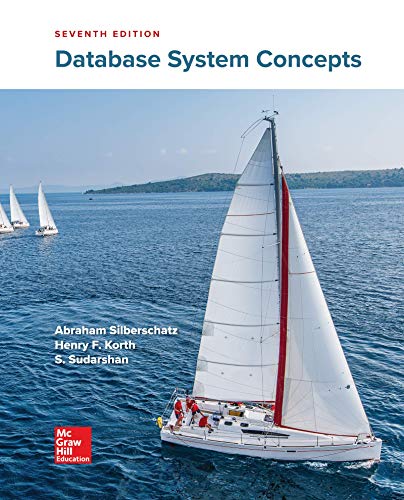
Java code
Question: -->The array Parent[3] has three index positions: 0, 1, and 2. We assigned a value to Parent[0] using the Child1.java class (know Child1.java is not a person just a set of instructions. It is a child of the Parent.java class.).
Please add values to index position Parent[1], and index position Parent[2]. Try deposit, withdraw, addFee. Then print a summary having the balance total of all three index position.
Here are the four codes:
(1)
public class Child1 extends Parent
{
private double amount;
private double balance;
public Child1(double amount)
{
super(amount);
this.amount = amount;
balance = this.amount;
}
public void deposit(double amount)
{
this.amount = amount;
balance = balance + amount;
}
public void withdraw(double amount)
{
this.amount = amount;
balance = balance - amount;
}
public double getBalance()
{
balance = balance + addFee(balance);
return balance;
}
public String toString()
{
String result = super.toString();
result = "Child1.java balance: " + balance;
return result;
}
}
(2)
public class mainClassPolyInherit
{
public static void main(String[] args)
{
SetupAccounts accounts = new SetupAccounts();
accounts.message();
}
}
(3)
abstract public class Parent
{
protected double amount;
protected double balance;
public Parent(double amount)
{
this.amount = amount;
balance = 0.0;
}
public void deposit(double amount)
{
this.amount = amount;
balance = balance + amount;
}
public void withdraw(double amount)
{
this.amount = amount;
balance = balance - amount;
}
public double getBalance()
{
return balance;
}
public double addFee(double balance)
{
balance = balance + 3.33;
return balance;
}
public String toString()
{
String result = "Parent.java balance: " + balance;
return result;
}
}
(4)
public class SetupAccounts
{
private Parent[] parentAcct;
double amount = 0.0;
public SetupAccounts()
{
parentAcct = new Parent[3];
parentAcct[0] = new Child1(100.01);
((Child1)parentAcct[0]).deposit(50.01);
}
public void message()
{
amount = parentAcct[0].getBalance();
System.out.println("Setup Accounts: " + amount);
}
}

Step by stepSolved in 2 steps

- In C Programming: Write a function printCourseRow() which receives a course pointer and prints all its fields as a single row. Use proper formatting so that when we print 2 or more courses as rows, the same members align below each other. Test the function, but don’t include the testing code in your homework.Upload a screenshot of a sample output.arrow_forwardHelp with writing a program a C program implements a bubble sort algorith on an array of integers, and use command line paramameters to populate an array with data. The program should follow below guidelines if possible: If there are no command-line arguments at all when the program is run, the program should print out instructions on its use (a "usage message"). There should be one common usage message (consider a method/function for printing the usage message) for any type of usage error. The program will accept an A or D as the second command line argument (after the program name). This letter will tell you whether the bubble sort should sort in ascending or descending fashion. Anything other than A or D in that position should display the usage message and terminate the program. The program will be able to accept up to 32 numbers (integers) on the command line. If there are more than 32 numbers on the command line, or no numbers at all, the program should print out the usage…arrow_forwardWhat are the drawbacks of using assembly language for non-specialist programming tasks? Is there ever a time when you'd recommend using assembly language?arrow_forward
- QUESTION 1 When defining a Java array, the index range of the array: Must start at 1 Must start at 0 Can be a customized range (from 2 to 5, e.g.) at compilation time Can be a customized range (from 2 to 5, e.g.) at run timearrow_forwardParagraph Styles Editing 5. Given the following series of n integers:1+2+3++n-2+n-1+nWrite a Java method that returns the sum of the series. 6. Given the following function:f(x)-2xFor, determine 7. Consider an array that stores the following sequence of integers:10, 15, 20, 25, 30Write a Java method that returns the sum of the integers stored in the array. The array must be passed to the method as an argument. I OFocus Earrow_forwardWrite a java program that uses an ArrayList object to store the following set of names in memory: [Steve, Tim, Lucy, Pat, Angela, Tom] Now write some more code so that the same ArrayList object is augmented with the name 'Steve' after the name 'Lucy' After the ArrayList object has been augmented with the new name, display the original and new lists on the console (as shown below), to verify that the new name is positioned correctly in the list.arrow_forward
- Classes, Objects, Pointers and Dynamic Memory Program Description: This assignment you will need to create your own string class. For the name of the class, use your initials from your name. The MYString objects will hold a cstring and allow it to be used and changed. We will be changing this class over the next couple programs, to be adding more features to it (and correcting some problems that the program has in this simple version). Your MYString class needs to be written using the .h and .cpp format. Inside the class we will have the following data members: Member Data Description char * str pointer to dynamic memory for storing the string int cap size of the memory that is available to be used(start with 20 char's and then double it whenever this is not enough) int end index of the end of the string (the '\0' char) The class will store the string in dynamic memory that is pointed to with the pointer. When you first create an MYString object you should…arrow_forwardPlease create the task program below in JAVA language. ASAP!! thanks!! Provide a screenshot for output and the source code.arrow_forwardNeed help making a java file that combines both linearSearch and binarySearch •Both search methods must use the Comparable<T> interface and the compareTo() method.•Your program must be able to handle different data types, i.e., use generics.•For binarySearch, if you decide to use a midpoint computation formula that is different fromthe textbook, explain that formula briefly as a comment within your code. //code from textbook //linearSearch public static <T> boolean linearSearch(T[] data, int min, int max, T target) { int index = min; boolean found = false; while (!found && index <= max) { found = data [index].equals(target); index++; } return found; } //binarySearch public static <T extends Comparable<T>> boolean binarySearch(T[] data, int min, int max, T target) { boolean found = false; int midpoint = (min + max)/2; if (data[midpoint].compareTo(target)==0)…arrow_forward
- Database System ConceptsComputer ScienceISBN:9780078022159Author:Abraham Silberschatz Professor, Henry F. Korth, S. SudarshanPublisher:McGraw-Hill EducationStarting Out with Python (4th Edition)Computer ScienceISBN:9780134444321Author:Tony GaddisPublisher:PEARSONDigital Fundamentals (11th Edition)Computer ScienceISBN:9780132737968Author:Thomas L. FloydPublisher:PEARSON
- C How to Program (8th Edition)Computer ScienceISBN:9780133976892Author:Paul J. Deitel, Harvey DeitelPublisher:PEARSONDatabase Systems: Design, Implementation, & Manag...Computer ScienceISBN:9781337627900Author:Carlos Coronel, Steven MorrisPublisher:Cengage LearningProgrammable Logic ControllersComputer ScienceISBN:9780073373843Author:Frank D. PetruzellaPublisher:McGraw-Hill Education
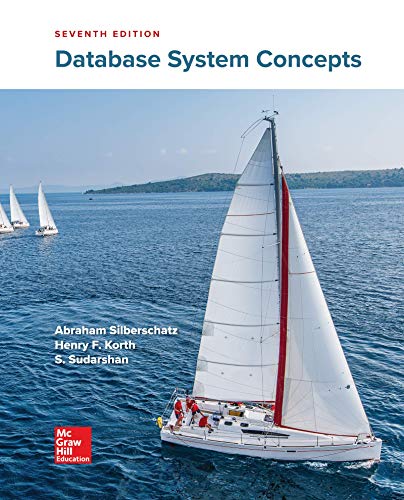
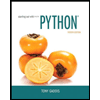
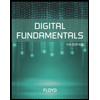
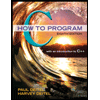
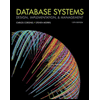
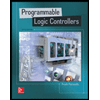