Using the above circular linked list which is implemented above in question, write a function that will remove all odd numbers stored at even nodes of the circular linked list. For example Linked List contains Data: 9,8,7,13,6 Output: 9,8,7,6
Data Structures and
Topic: Doubly and circular Linked Lists in java:
Please solve this on urgent basis:
Attach output's picture and explain every statement in commments:
Implemented Circular Linked List CODE:
#include <bits/stdc++.h> // include the standard c++ header file
using namespace std;
class Node // definition of the class for the node of the linked list
{
public:
int data;
Node* next;
};
void push(Node** head_ref, int data) // definition of the function to insert the element in the list
{
Node* ptr1 = new Node();
ptr1->data = data;
ptr1->next = *head_ref;
if (*head_ref != NULL) // when the head of the list does not points to null
{
Node* temp = *head_ref;
while (temp->next != *head_ref)
temp = temp->next;
temp->next = ptr1;
}
else // when the head points to null
ptr1->next = ptr1;
*head_ref = ptr1;
}
void printList(Node* head) // function to display the list
{
Node* temp = head;
if (head != NULL)
{
do // loop to access every element of the list
{
cout << temp->data << " ";
temp = temp->next;
} while (temp != head);
}
cout << endl;
}
void deleteNode(Node** head, int key) // function definition to delete node from the list
{
if (*head == NULL)
return;
if((*head)->data==key && (*head)->next==*head)
{
free(*head);
*head=NULL;
return;
}
Node *last=*head,*d;
if((*head)->data==key) // when head points to the required key
{
while(last->next!=*head)
last=last->next;
last->next=(*head)->next;
free(*head);
*head=last->next;
}
while(last->next!=*head&&last->next->data!=key)
{
last=last->next;
}
if(last->next->data==key)
{
d=last->next;
last->next=d->next;
free(d);
}
else
cout<<"no such keyfound";
}
int main() // starts the definition of the main function
{
Node* head = NULL;
push(&head, 9); // method to insert the elements in the list
push(&head, 5);
push(&head, 5);
push(&head, 7);
push(&head, 4);
cout << "List after insertion operation: ";
printList(head); // call the method to display the list
deleteNode(&head, 7); // function to delete the node
cout << "List After Deletion operation: ";
printList(head); // calls the function to display the list
return 0;
}
Using the above circular linked list which is implemented above in question, write a function that will remove all odd numbers stored at even nodes of the circular linked list.
For example
Linked List contains Data: 9,8,7,13,6
Output: 9,8,7,6
Explanation: It will check if a number is odd and stored at even node it will simply remove it and check other elements. You can use the functions that you have developed in the Circular List class.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

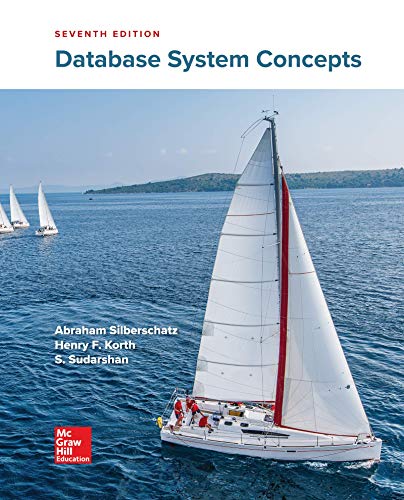
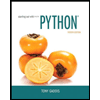
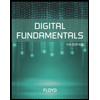
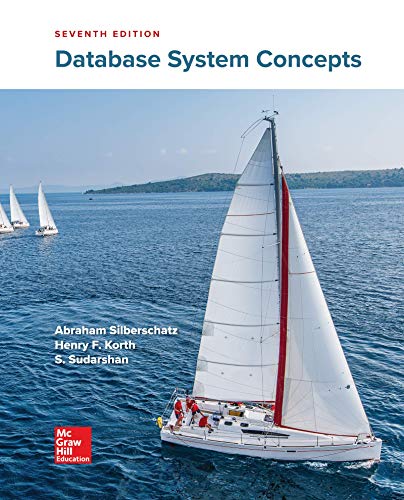
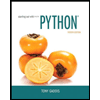
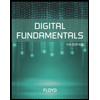
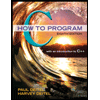
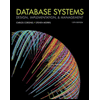
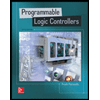