python assignment Matrix class Implement a matrix class (in matrix.py). a) The initializer should take a list of lists as an argument, where each outer list is a row, and each value in an inner list is a value in the corresponding row. b) Implement the __str__ method to nicely format the string representation of the matrix: one line per row, two characters per number (%2d) and a space between numbers. For example: m = Matrix([[1,0,0],[0,1,0],[0,0,1]]) print(m) > 1 0 0 > 0 1 0 > 0 0 1 c) Implement a method scale(factor) that returns a new matrix where each value is multiplied by scale. For example: m = Matrix([[1,2,3],[4,5,6],[7,8,9]]) n = m.scale(2) print(n)> 2 4 6 > 8 10 12 >14 16 18 print(m) > 1 2 3 > 4 5 6 > 7 8 9 d) Implement a method transpose() that returns a new matrix that has been transposed. Transposing flips a matrix over its diagonal: it switches rows and columns. m = Matrix([[1,2,3],[4,5,6],[7,8,9]]) print(m) > 1 2 3 > 4 5 6 > 7 8 9 print(m.transpose()) > 1 4 7 > 2 5 8 > 3 6 9 e) Implement a method a.multiply(b) that gives a new matrix, c, that is the result of multiplying a by b. Matrix multiplication only works if matrix a has the same number of columns as matrix b has rows. If this is not the case, please return None. The value of matrix c at row i, column j is defined as: cij=ai1b1j+ai2b2j+...+ainbnj Where aij is the value of matrix a at row i, column j. e = Matrix([[1, 2, 3], [2, 1, 3], [3, 2, 1]]) print(e.multiply(e)) >14 10 12 >13 11 12 >10 10 16
python assignment
Matrix class
Implement a matrix class (in matrix.py).
a) The initializer should take a list of lists as an argument, where each outer list is a row, and each value in an inner list is a value in the corresponding row.
b) Implement the __str__ method to nicely format the string representation of the matrix: one line per row, two characters per number (%2d) and a space between numbers. For example:
m = Matrix([[1,0,0],[0,1,0],[0,0,1]])
print(m)
> 1 0 0
> 0 1 0
> 0 0 1
c) Implement a method scale(factor) that returns a new matrix where each value is multiplied by scale. For example:
m = Matrix([[1,2,3],[4,5,6],[7,8,9]])
n = m.scale(2)
print(n)> 2 4 6
> 8 10 12
>14 16 18
print(m)
> 1 2 3
> 4 5 6
> 7 8 9
d) Implement a method transpose() that returns a new matrix that has been transposed. Transposing flips a matrix over its diagonal: it switches rows and columns.
m = Matrix([[1,2,3],[4,5,6],[7,8,9]])
print(m)
> 1 2 3
> 4 5 6
> 7 8 9
print(m.transpose())
> 1 4 7
> 2 5 8
> 3 6 9
e) Implement a method a.multiply(b) that gives a new matrix, c, that is the result of multiplying a by b. Matrix multiplication only works if matrix a has the same number of columns as matrix b has rows. If this is not the case, please return None. The value of matrix c at row i, column j is defined as:
cij=ai1b1j+ai2b2j+...+ainbnj
Where aij is the value of matrix a at row i, column j.
e = Matrix([[1, 2, 3], [2, 1, 3], [3, 2, 1]])
print(e.multiply(e))
>14 10 12
>13 11 12
>10 10 16

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

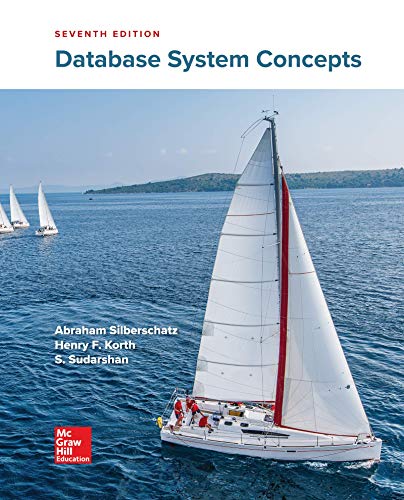
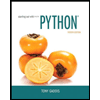
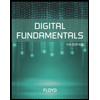
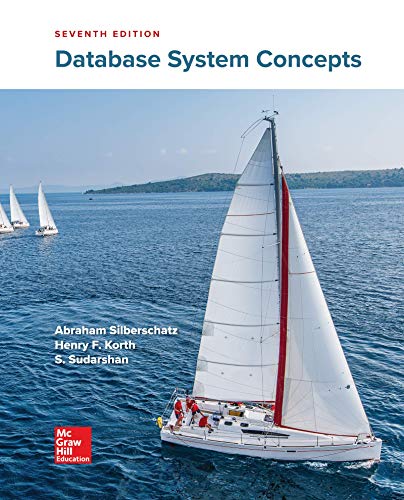
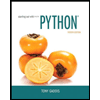
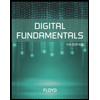
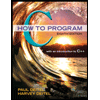
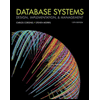
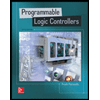