Using SIMPLE java, I need some help with file encryption. Looking for a way to read the first file one character at a time, and add some number between 1 and 31 to the ASCII character code of each character before it is written to the second file. The input file will contain printable characters from character 32 to character 125 in the ASCII character set. Thanks! Here is code I have and I need help with the encrpytFile method. public class Crypto { /** The encryptFile method makes an encrypted copy of an existing file. @param existing The name of the existing file to encrypt. @param encrypted The name of the encrypted file to create. @exception IOException When an IO error occurs. */ private static final byte KEY = 10; public static void encryptFile(String existing, String encrypted) { } public class FileEncryptionFilter { public static void main(String[] args) { System.out.println("Encrypting the contents of the file"); System.out.println("MyLetters.txt. The encrypted file will"); System.out.println("be stored as Encrypted.txt"); try { Crypto.encryptFile("src//MyLetters.txt", "src//Encrypted.txt"); System.out.println("Done. Use Notepad to inspect the encrypted file."); } catch (IOException e) { System.out.println("Error - " + e.getMessage()); } } }
Using SIMPLE java, I need some help with file encryption. Looking for a way to read the first file one character at a time, and add some number between 1 and 31 to the ASCII character code of each character before it is written to the second file. The input file will contain printable characters from character 32 to character 125 in the ASCII character set. Thanks!
Here is code I have and I need help with the encrpytFile method.
public class Crypto
{
/**
The encryptFile method makes an encrypted copy
of an existing file.
@param existing The name of the existing file to encrypt.
@param encrypted The name of the encrypted file to create.
@exception IOException When an IO error occurs.
*/
private static final byte KEY = 10;
public static void encryptFile(String existing, String encrypted)
{
}
public class FileEncryptionFilter
{
public static void main(String[] args)
{
System.out.println("Encrypting the contents of the file");
System.out.println("MyLetters.txt. The encrypted file will");
System.out.println("be stored as Encrypted.txt");
try
{
Crypto.encryptFile("src//MyLetters.txt", "src//Encrypted.txt");
System.out.println("Done. Use Notepad to inspect the encrypted file.");
}
catch (IOException e)
{
System.out.println("Error - " + e.getMessage());
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

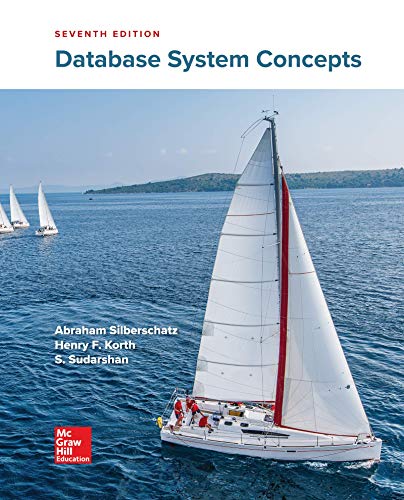
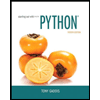
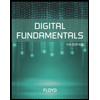
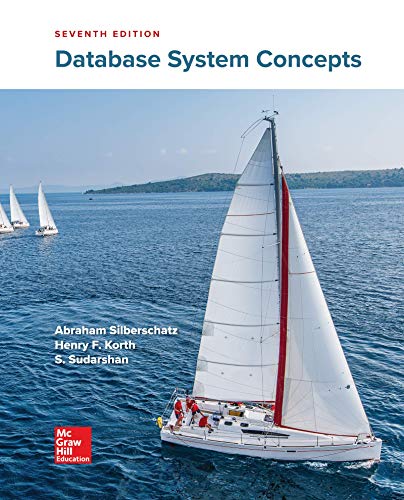
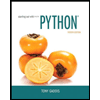
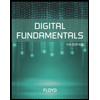
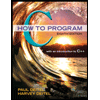
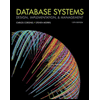
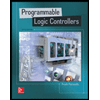