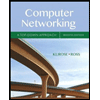
Using SIMPLE java, I need some help with file encryption. Looking for a way to include the ability to decrypt encrypted messages. The decryption program should read the contents of "Encrypted.txt", restore the data to its original state, and write it to a file named "Decrypted.txt". Also demonstrate appropriate exception handling for file I/O. Thanks!
Here is code I have and I need help with the decryptFile method.
public class Crypto
{
/**
The decryptFile method decrypts an encrypted file.
@param existing The name of the existing file to decrypt.
@param decrypted The name of the decrypted file to create.
@exception IOException When an IO error occurs.
*/
private static final byte KEY = 10;
public static void decryptFile(String existing, String encrypted)
{
}
public class FileDecryptionFilter
{
public static void main(String[] args)
{
System.out.println("Decrypting the contents of the file");
System.out.println("Encrypted.txt. The encrypted file will");
System.out.println("be stored as MyLetters.txt");
try
{
Crypto.decryptFile("src//Encrypted.txt", "src//Decrypted.txt");
System.out.println("Done. Use Notepad to inspect the decrypted file.");
}
catch (IOException e)
{
System.out.println("Error - " + e.getMessage());
}
}
}

Trending nowThis is a popular solution!
Step by stepSolved in 2 steps with 3 images

- First, launch NetBeans and close any previous projects that may be open (at the top menu go to File ==> Close All Projects).Then create a new Java application called "CheckString" (without the quotation marks) according to the following guidelines.** Each method below, including main, should handle (catch) any Exceptions that are thrown. ** ** If an Exception is thrown and caught, print the Exception's message to the command line. ** Write a complete Java method called checkWord that takes a String parameter called word, returns nothing, and is declared to throw an Exception of type Exception. In the method, check if the first character of the parameter is a letter. If it is not a letter, the method throws an Exception of type Exception with the message of: "This is not a word." void checkWord(String word) throws Exception Write a complete Java method called getWord that takes no parameters and returns a String. The method prompts the user for a word, and then calls the checkWord…arrow_forwardMCQ 1. A Java programme may deal with an exception in a variety of ways. None of the above is not a possible method for a Java programme to deal with an exception?a. disregard the exception b. manage the exception using try to capture phrases as it occursc. pass the exception to another process for handling d. pass the exception to a predefined Exception class for handlinge. Any of these is a legitimate method of handling an exception.arrow_forwardYour task is to build a simple login system to ask users for a username and a password and then ask them a simple mathematical question to check if they are human. Building proper exceptions and when to use them is learned through practice. This assessment will be focused on building out try-except blocks to manage user inputs, which are the most common sources of errors. You will have to build the following pieces of code. Variables needed User Input Input variable to hold password Input variable to hold username Input variable for math answer Backend Variables Math Answer Math Value 1 Math Value 2 Methods Random number generator for values of math values 1 and 2 Division method to divide math values 1 and 2 and return math answer Comparator between user math answer to math answer to see if they are equal or not If the answers are not the same, ask the user to reenter the value again A method to ensure that password and username are string values Method for console input…arrow_forward
- Select the one option that best completes this sentence: Exceptions are ... Select one: O a. a mechanism for dealing with regular outcomes of calculations in your programme. Ob. a mechanism for handling exceptional events while your programme is running to handle them gracefully. Ос. a mechanism for detecting extreme values in your data. d. a mechanism that can be used instead of an if-statement to provide more sophisticated handling of conditional code execution.arrow_forwardAnswer the following questions based on your knowledge of java exception and java text and binary I/Os: Java exception can be handled locally using try-catch block or can be thrown to the calling When should you prefer to throw an exception to the calling method instead of using try-catch? Is it possible to write a program to read from a text file without handling the exception? Why should a programmer consider binary I/O instead of text I/O? Suppose that you are implementing a program to write a string “সিএিই-110” in a binary file. Java provides you three different methods – writeBytes(String), writeChars(String), and writeUTF(String). How many bytes will be written by each of those method for the give string? Why should not you consider the writeBytes(String) method in this case?arrow_forwardPost a total of 3 substantive responses over 2 separate days for full participation. This includes your initial post and 2 replies to classmates or your faculty member. Due Day 3 It’s important to program your code efficiently. Efficient code manages errors and exceptions and cleans up memory after it ends. The try-except statements are helpful in handling errors that are detected during execution. Respond to the following in a minimum of 175 words: What are the 2 categories of errors when debugging code? How can the try-except statements handle errors in Python? Provide a code example that supports your comments.arrow_forward
- Create and implement a programme that generates the InvalidDocumentCodeException exception class, which is intended to be fired if an incorrect designation for a document is discovered during processing. Consider a scenario where all papers in a certain company are assigned a two-character identification that begins with U, C, or P, which stands for unclassified, confidential, or proprietary. The exception is raised if a document designation is found that doesn't suit that description. To test the exception and allow it to end the programme, create a driver programme.arrow_forwardnew java code can only be added between lines 22 - 24. as seen in image.arrow_forwardsolve:arrow_forward
- could you please code this on notepade.arrow_forwardHello, I am struggling to write a program in Python for a following problem: Write a file ( .py). In that file create a function that strips the first letters from all file names in a directory. If the new name is a duplicate of an existing name, Python will throw an exception. Handle that exception by adding “dup” a number as part of the files new name. Call that function from a Jupyter Notebook, passing in the number of characters to delete and the file path. Thank you!arrow_forwardWrite a Python Program to handle different types of exceptions that occurs at runtime in the Program and also the method of raising an exception.arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
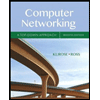
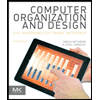
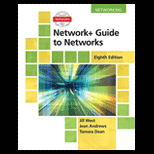
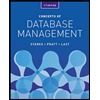
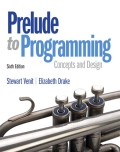
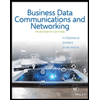