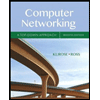
java
Using classes, design an online address book to keep track of the names,
addresses, phone numbers, and birthdays of family members, close friends,
and certain business associates. Your program should be able to handle a
maximum of 500 entries.
a. Define the class Address that can store a street address, city, state, and
zip code. Use the appropriate methods to print and store the address.
Also, use constructors to automatically initialize the data members.
b. Define the class ExtPerson using the class Person (as defined in
Example 8-8, Chapter 8), the class Date (as designed in this chapter’s
Programming Exercise 2), and the class Address. Add a data member
to this class to classify the person as a family member, friend, or business
associate. Also, add a data member to store the phone number. Add
(or override) methods to print and store the appropriate information.
Use constructors to automatically initialize the data members.
c. Define the class AddressBook using previously defined classes. An object
of type AddressBook should be able to process a maximum of 500 entries.
The program should perform the following operations:
i. Load the data into the address book from a disk.
ii. Sort the address book by last name.
iii. Search for a person by last name.
iv. Print the address, phone number, and date of birth (if available) of a
given person.
v. Print the names of the people whose birthdays are in a given month or
between two given dates.
vi. Print the names of all the people between two last names.

Trending nowThis is a popular solution!
Step by stepSolved in 7 steps with 1 images

- Hi, this is a java programming question, I'll attach the image and type the question Implement the class diagram below (diagram attached) Create a new class called LibraryManagementService and implement the main method. Inside the main method:• Create the following book objects.o Title: Sherlock Homeso Author: Arthur Conan Doyleo Genre: Detective fictiono Title: Pride and Prejudiceo Author: Jane Austeno Genre: Fictiono Title: Anne of Green Gableso Author: Jane Eyreo Genre: Dramao Title: Leave it to PSmitho Author: P G Wodehouseo Genre: Comedyo Title: Angels and Demonso Author: Dan Browno Genre: Suspense• All titles are case insensitive (Sherlock Homes and SHERLOCK HOMES should refer to the same book)• By default, for all the books, isAvailable should be true• Implement the following methods in the Library class:o addBook() should take in a book object and should add it to the books array. For instance, if we create a 6th book object• Title: Jurassic Park• Author: Michael Crichton•…arrow_forwardJava Program This assignment requires one project with two classes. Class Employee Class Employee- I will attach the code for this: //Import the required packages. import java.text.DecimalFormat; import java.text.NumberFormat; //Define the employee class. class Employee { //Define the data members. private String id, lastName, firstName; private int salary; //Create the constructor. public Employee(String id, String lastName, String firstName, int salary) { this.id = id; this.lastName = lastName; this.firstName = firstName; this.salary = salary; } //Define the getter methods. public String getId() { return id; } public String getLastName() { return lastName; } public String getFirstName() { return firstName; } public int getSalary() { return salary; } //Define the method to return the employee details. @Override public String toString() { //Use number format and decimal format //to…arrow_forward
- Computer Networking: A Top-Down Approach (7th Edi...Computer EngineeringISBN:9780133594140Author:James Kurose, Keith RossPublisher:PEARSONComputer Organization and Design MIPS Edition, Fi...Computer EngineeringISBN:9780124077263Author:David A. Patterson, John L. HennessyPublisher:Elsevier ScienceNetwork+ Guide to Networks (MindTap Course List)Computer EngineeringISBN:9781337569330Author:Jill West, Tamara Dean, Jean AndrewsPublisher:Cengage Learning
- Concepts of Database ManagementComputer EngineeringISBN:9781337093422Author:Joy L. Starks, Philip J. Pratt, Mary Z. LastPublisher:Cengage LearningPrelude to ProgrammingComputer EngineeringISBN:9780133750423Author:VENIT, StewartPublisher:Pearson EducationSc Business Data Communications and Networking, T...Computer EngineeringISBN:9781119368830Author:FITZGERALDPublisher:WILEY
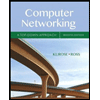
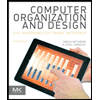
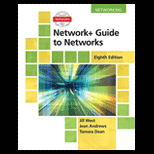
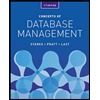
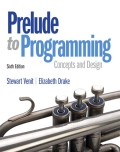
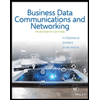