Using C++ Language Start with the provided code and understand what it is doing. We are interested in computing the maximum grade out of the 6 quiz scores for each student in class. Assume the maximum class size is 10, but we will ask the teacher how many students there are in the class. It cannot be more than 10. In the below program, we create a two-dimensional array with the number of rows equal to the number of students (one for each student) and 6 columns (one column for each quiz grade). code: #include using namespace std; const int COLS = 6; const int ROWS = 10; void computeMaximum (float grades[][COLS], int num, float maximum[]); void showArray(float array[], int size); int main() { float grades[ROWS][COLS]; float maximum[ROWS]; int num; cout<<"How many students in the class?"; cin>>num; for (int row = 0; row < num; row++) { //enters 6 quiz grades for each student for (int col = 0; row< COLS; col++) { cout<<"Enter quiz #"<>grades[row][col]; } } //call function computeMaximum for (int row=0; row
Using C++ Language
Start with the provided code and understand what it is doing. We are interested in
computing the maximum grade out of the 6 quiz scores for each student in class. Assume
the maximum class size is 10, but we will ask the teacher how many students there are in
the class. It cannot be more than 10.
In the below program, we create a two-dimensional array with the number of rows equal
to the number of students (one for each student) and 6 columns (one column for each
quiz grade).
code:
#include <iostream>
using namespace std;
const int COLS = 6;
const int ROWS = 10;
void computeMaximum (float grades[][COLS], int num, float maximum[]);
void showArray(float array[], int size);
int main()
{
float grades[ROWS][COLS];
float maximum[ROWS];
int num;
cout<<"How many students in the class?";
cin>>num;
for (int row = 0; row < num; row++)
{
//enters 6 quiz grades for each student
for (int col = 0; row< COLS; col++)
{
cout<<"Enter quiz #"<<col+1<<" for student #"<<row+1<<": ";
cin>>grades[row][col];
}
}
//call function computeMaximum
for (int row=0; row<num; row++)
{
for (int col=0; col<COLS; col++)
{
cout<<grades[row][col]<<" ";
}
}
cout<<"The maximum grades:\n";
//call function showArray
cout<<endl;
return 0;
}
Now, make the following changes to your code.
• The function computeMaximum computes the maximum quiz score out of the 6
scores of each student and stores them in the maximum array. Write this function
in a C++ code file named computeMaximum.cpp.
• The function showArray displays the content of the array passed to the
function. Write this function in a C++ code file named showArray.cpp.
• Create a header file called Lab8_Header.h. Move the include and the using
namespace statements, as well as function and constant declarations to this file.
• Include Lab8_Header.h file in your three .cpp files.
• Make sure all files are in the same folder before you compile and test.

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 7 images

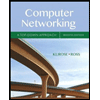
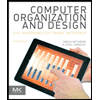
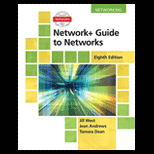
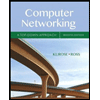
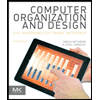
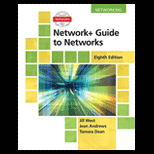
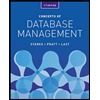
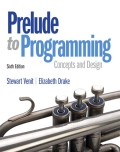
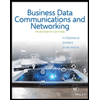