Using a for Loop Summary In this lab the completed program should print the numbers O through 10, along with their values multiplied by 2 and by 10. You should accomplish this using a for loop instead of a counter- controlled while loop. Instructions 1. Write a for loop that uses the loop control variable to take on the values O through 10. 2. In the body of the loop, multiply the value of the loop control variable by 2 and by 10. 3. Execute the program by clicking the Run button at the bottom of the screen. Is the output the same?
Types of Loop
Loops are the elements of programming in which a part of code is repeated a particular number of times. Loop executes the series of statements many times till the conditional statement becomes false.
Loops
Any task which is repeated more than one time is called a loop. Basically, loops can be divided into three types as while, do-while and for loop. There are so many programming languages like C, C++, JAVA, PYTHON, and many more where looping statements can be used for repetitive execution.
While Loop
Loop is a feature in the programming language. It helps us to execute a set of instructions regularly. The block of code executes until some conditions provided within that Loop are true.



Trending now
This is a popular solution!
Step by step
Solved in 2 steps

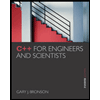
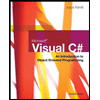
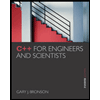
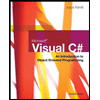