USE SIMPLE PYTHON MULTI THREADING code to perform parallel array summing. replace the mthod of using processes to complete and use threading techniques to do the same the thing. 1) Basic version with two levels of threads (master and slaves) One master thread aggregates and sums the result of n slave-threads where each slavethread sums a different range of values in an array of 1000 random integers (please program to generate 1000 random integers to populate the array). The number of slave-threads is a parameter which the user can change. For example, if the user chooses 4 slave threads, each slave thread will sum 1000/4 = 250 numbers. If the user chooses 3 slave threads, the first two may each sum 333 numbers and the third slave thread sums the rest 334 numbers. 2) Advanced version with more than two levels of threads The master thread creates two slave-threads where each slave-thread is responsible to sum half segment of the array. Each slave thread will fork/spawn two new slave-threads where each new slave-thread sums half of the array segment received by its parent. Each slave thread will return the subtotal to its parent thread and the parent thread aggregates and returns the total to its parent thread. Start with 7 nodes thread tree, when you are comfortable, you can extend it to a full thread tree.
USE SIMPLE PYTHON MULTI THREADING code to perform parallel array summing. replace the mthod of using processes to complete and use threading techniques to do the same the thing.
1) Basic version with two levels of threads (master and slaves)
One master thread aggregates and sums the result of n slave-threads where each slavethread sums a different range of values in an array of 1000 random integers (please
program to generate 1000 random integers to populate the array).
The number of slave-threads is a parameter which the user can change. For example, if the
user chooses 4 slave threads, each slave thread will sum 1000/4 = 250 numbers. If the user
chooses 3 slave threads, the first two may each sum 333 numbers and the third slave thread
sums the rest 334 numbers.
2) Advanced version with more than two levels of threads
The master thread creates two slave-threads where each slave-thread is responsible to sum
half segment of the array.
Each slave thread will fork/spawn two new slave-threads where each new slave-thread
sums half of the array segment received by its parent. Each slave thread will return the
subtotal to its parent thread and the parent thread aggregates and returns the total to its
parent thread. Start with 7 nodes thread tree, when you are comfortable, you can extend it
to a full thread tree.

Here's a sample code for the basic version of parallel array summing using threading in Python:
CODE in PYTHON:
import random
import threading
def sum_array(arr, start, end, result):
total = 0
for i in range(start, end):
total += arr[i]
result.append(total)
def parallel_sum(arr, num_threads):
n = len(arr)
chunk_size = n // num_threads
threads = []
result = []
for i in range(num_threads):
start = i * chunk_size
end = start + chunk_size
if i == num_threads - 1:
end = n
thread = threading.Thread(target=sum_array, args=(arr, start, end, result))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
return sum(result)
# Generate 1000 random integers
arr = [random.randint(0, 1000) for _ in range(1000)]
# Test parallel_sum function with 4 threads
print(parallel_sum(arr, 4))
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

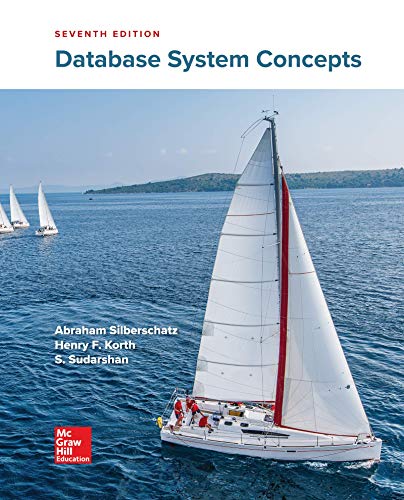
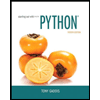
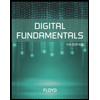
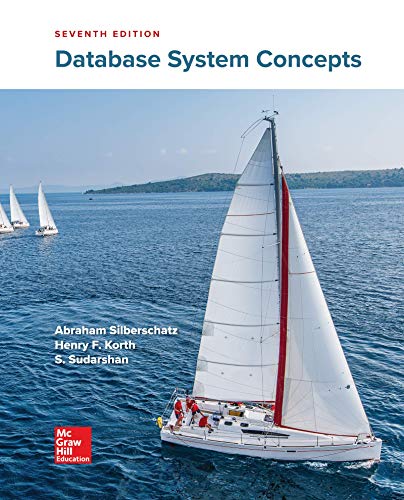
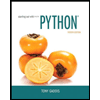
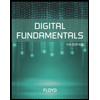
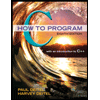
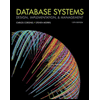
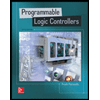