Use Java language ONLY Mastermind is a code-breaking game between two players, the codemaker (in this case the computer) and the codebreaker (the player). How to Play See the following video https://www.youtube.com/watch?v=XwuwWTH39ac. Project Requirements The goal of the project is to implement a text-based/menu-driven Mastermind game. The game will include a menu from which the user will choose: See Rules Play Game See Highest Score Quit When the user chooses to play a game, the computer will generate a three-position code using five colors (Red/Blue/White/Yellow/Green).
Use Java language ONLY
Mastermind is a code-breaking game between two players, the codemaker (in this case the computer) and the codebreaker (the player).
How to Play
See the following video https://www.youtube.com/watch?v=XwuwWTH39ac.
Project Requirements
The goal of the project is to implement a text-based/menu-driven Mastermind game.
The game will include a menu from which the user will choose:
- See Rules
- Play Game
- See Highest Score
- Quit
- When the user chooses to play a game, the computer will generate a three-position code using five colors (Red/Blue/White/Yellow/Green).
The player will try to break the code by choosing a color for each of the three positions available - color repeats are allowed. Example: R G B (Red-Green-Blue)
The computer will provide feedback using three pins (one for each color) placed in non-related order:
-
- Red Pin (R)– A color is part of the code and has been placed on the right position.
- White Pin(W) – A color is part of the code but has been placed on the wrong position.
- No Pin (O)– The color is incorrect.
The game ends when either:
-
- The player wins. Breaks the code – three colors on the exact positions as initially generated by the computer. The player’s score is the round when the code was broken (1-10).
- The player loses. No code is broken after ten attempts. No score is awarded.
- Example
Assume the computer has generated the following code:
R G B
A possible output at the end of the second round could be:
Colors Pins
R R R R O O
R B Y R W O
- At the end of each game, the system will read in the score stored in “highscore.txt”.
- If the file does not exist, it will create one with the current player as the high score (name & score).
- If the user has scored higher than the value in the file, it will replace the text file with the current player’s name and score.
- Otherwise, the text file will remain intact.
- The user should be able to play as many times as he/she wishes.
Notes about Functionality, Implementation & Program Requirements:
- The program should validate the information entered by the user and display the appropriate error messages.
- The code should be self-documented, including:
-
-
- Descriptive Comment indicating
- Author
- Program Summary
- IPO Chart
- Appropriate comments throughout the code that highlight, among other things, the implementation of the
algorithm . - Adequate use of spaces and indentation so the source code is easy to read when printed out.
- Descriptive Comment indicating
- Functional decomposition implementation of the algorithm. Must define at least four different functions. Make sure to include functions that exemplify void vs. return-type, and value vs. reference parameters.
- Two-Dimensional array implementation. The mastermind color & pin boards must be implemented using parallel two-dimensional arrays.
-
Grading Rubric
Documentation & Algorithm (5) ______
Menu-Driven interface (10) ______
- Option driving
- Looping
See Rules (5) ______
Game Implementation (25) ______
- Generate code
Random function seeding & color generation - Prompt player for guess
- Provide feedback
- Determine win/lose
- Loop up to ten rounds
Array Implementation (20) ______
- Two-Dimensional Arrays
- Parallel Arrays
File Manipulation (20) ______
- See highest score
- Create/Update highscore.txt
Functional Decomposition (20) ______
- Methods
Game Interface (5) ______
- Add you own creativity
Total (110/100)

Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

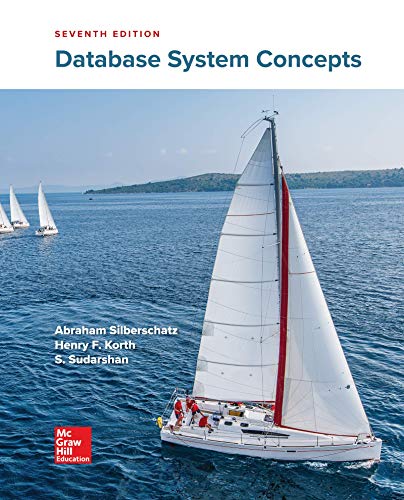
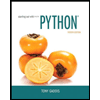
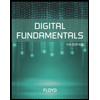
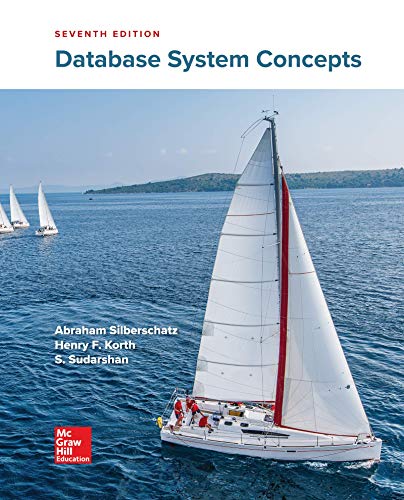
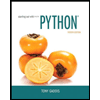
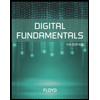
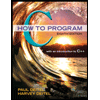
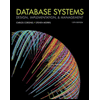
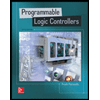