Use C++. This lab will help you practice with dynamic memory (NOT mixed with classes). Program Information One statistic of interest to many researchers is the median of a set of data. The median is the value in the middle. (This is NOT the average, mind you.) Being in the middle, of course implies that the data are in order (sorted). If there are an odd number of data items, the middle is simple to find. If there are an even number of data items, you are supposed to take the average of the two middle values. You'll need to find the median of a set of data from a file. You don't know how many data are in the file, of course. You'll have to count the data, then read them in to sort, then find the median, and finally print it (the median) out. Do NOT use any more memory than is necessary!!
Topical Information
Use C++. This lab will help you practice with dynamic memory (NOT mixed with classes).
Program Information
One statistic of interest to many researchers is the median of a set of data. The median is the value in the middle. (This is NOT the average, mind you.) Being in the middle, of course implies that the data are in order (sorted). If there are an odd number of data items, the middle is simple to find. If there are an even number of data items, you are supposed to take the average of the two middle values.
You'll need to find the median of a set of data from a file. You don't know how many data are in the file, of course. You'll have to count the data, then read them in to sort, then find the median, and finally print it (the median) out.
Do NOT use any more memory than is necessary!!

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

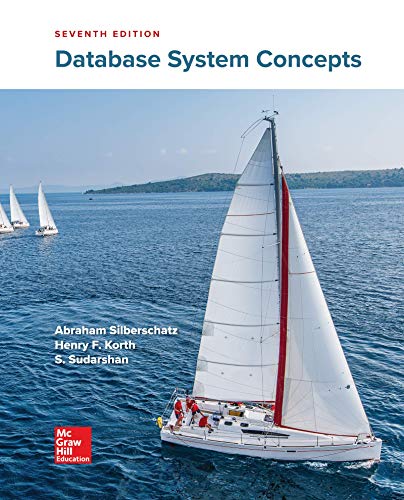
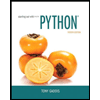
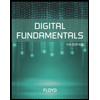
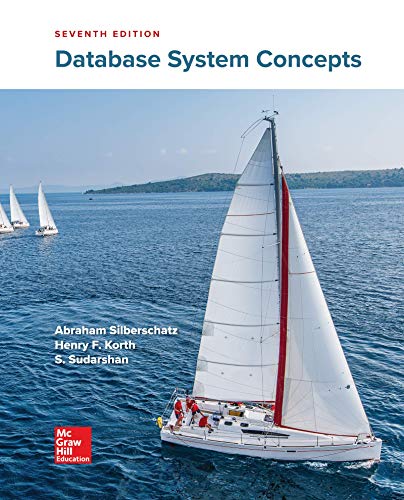
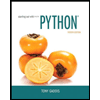
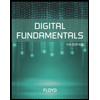
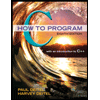
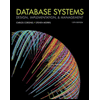
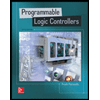