Data Structure Using C++ (Queue) C++ code (NOT JAVA C++ JUST) C++ PROGRAMMING LANGUAGE PLEASE :: We can use a queue to simulate the flow of customers through a check-out line in a store. In this simulation we will have the following details: one check-out line the expected service time for each customer is one minute (However, they may have to wait in line before being serviced) between zero and two customers join the line every minute We can simulate the flow of customers through the line during a time period n minutes long using the following algorithm: Initialize the queue to empty. for ( minute = 0 ; minute < n ; ++minute ) { if the queue is not empty, then remove the customer at the front of the queue. Compute a random number k between 0 and 3. If k is 1, then add one customer to the line. If k is 2, then add two customers to the line. Otherwise (if k is 0 or 3), do not add any customers to the line. } In addition, the algorithm will keep track of the following: the total number of customers served the combined total wait time of all customers the maximum length of time any of these customers spent waiting in line Operations on the Queue for the Check-out Line Simulation Given a time of 5 minutes, the following demonstrates the operations on the queue: use 1.png this is for test the program :: use 2.png nd you can use these thing :::: struct node { int data; node *next; node(int d,node *n=0) { data=d; next=n; } }; class stack { node *topp; public: stack(); void push(int el); bool pop(); int top(); bool top(int &el); //~stack(); //void operator=(stack &o); //stack(stack &o); }; stack::stack() { topp=0; } void stack::push(int el) { topp=new node(el,topp); } bool stack::pop() { if(topp==0) return false; node *t=topp; topp=topp->next; delete t; return true; } int stack::top() { if(topp!=0) return topp->data; } bool stack::top(int &el) { if(topp==0) return false; el=topp->data; return true; } please need a full code without any errors
Data Structure Using C++ (Queue) C++ code (NOT JAVA C++ JUST)
C++ PROGRAMMING LANGUAGE PLEASE ::
We can use a queue to simulate the flow of customers through a check-out line in a store. In this simulation we will have the following details:
- one check-out line
- the expected service time for each customer is one minute (However, they may have to wait in line before being serviced)
- between zero and two customers join the line every minute
We can simulate the flow of customers through the line during a time period n minutes long using the following
Initialize the queue to empty.
for ( minute = 0 ; minute < n ; ++minute )
{
if the queue is not empty, then remove the customer at the front of the queue.
Compute a random number k between 0 and 3.
If k is 1, then add one customer to the line.
If k is 2, then add two customers to the line.
Otherwise (if k is 0 or 3), do not add any customers to the line.
}
In addition, the algorithm will keep track of the following:
- the total number of customers served
- the combined total wait time of all customers
- the maximum length of time any of these customers spent waiting in line
Operations on the Queue for the Check-out Line Simulation
Given a time of 5 minutes, the following demonstrates the operations on the queue:
use 1.png
this is for test the program ::
use 2.png
nd you can use these thing ::::
struct node { int data; node *next; node(int d,node *n=0) { data=d; next=n; } }; class stack { node *topp; public: stack(); void push(int el); bool pop(); int top(); bool top(int &el); //~stack(); //void operator=(stack &o); //stack(stack &o); }; stack::stack() { topp=0; } void stack::push(int el) { topp=new node(el,topp); } bool stack::pop() { if(topp==0) return false; node *t=topp; topp=topp->next; delete t; return true; } int stack::top() { if(topp!=0) return topp->data; } bool stack::top(int &el) { if(topp==0) return false; el=topp->data; return true; }
please need a full code without any errors



Trending now
This is a popular solution!
Step by step
Solved in 3 steps

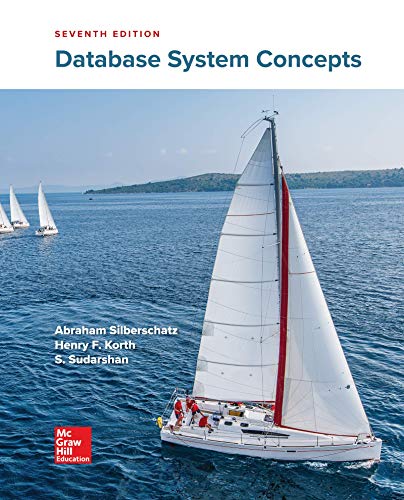
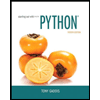
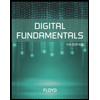
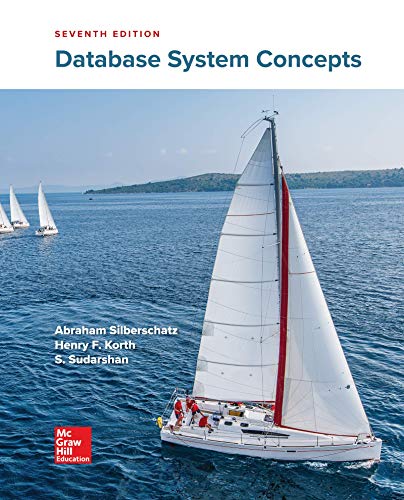
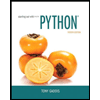
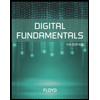
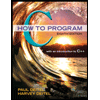
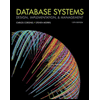
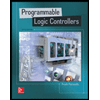