Update the following PHP script to check the users answer to this quiz. The answer key is provided as part of the HTML and the weight of each question is also given. When the user submits the quiz provide them a report of how they did. Include points they got right, percentage score, and letter grade. Add feedback in mon-day-year hh24:mi Great Explorers Quiz = 90: $letter_grade = 'A'; break; case $score_percent >= 80: $letter_grade = 'B'; break; case $score_percent >= 70: $letter_grade = 'C'; break; case $score_percent >= 60: $letter_grade = 'D'; break; default: $letter_grade = 'F'; } } // provide feedback on submission ?>
Update the following PHP script to check the users answer to this quiz. The answer key is provided as part of the HTML and the weight of each question is also given. When the user submits the quiz provide them a report of how they did. Include points they got right, percentage score, and letter grade. Add feedback in mon-day-year hh24:mi
<!DOCTYPE html>
<!--
To change this license header, choose License Headers in Project Properties.
To change this template file, choose Tools | Templates
and open the template in the editor.
-->
<html>
<head>
<meta charset="UTF-8">
<title>Great Explorers Quiz</title>
</head>
<body>
<?php
// put your code here
$points_possible = 36;
$points_correct = 0;
$letter_grade = '';
$score_percent = 0;
$feedback= date('M-d-Y-H:i');
if (isset($_POST['grade'])) {
$question1 = $_POST['question1'];
$question2 = $_POST['question2'];
$question3 = $_POST['question3'];
$question4 = $_POST['question4'];
$question5 = $_POST['question5'];
// check each answer and add points for correct ones
if ($question1 === 'd') {
$points_correct += 5;
}
if ($question2 === 'b') {
$points_correct += 5;
}
if ($question3 === 'a') {
$points_correct += 8;
}
if ($question4 === 'b') {
$points_correct += 8;
}
if ($question5 === 'c') {
$points_correct += 10;
}
// calculate the score percent and letter grade
$score_percent = round($points_correct / $points_possible * 100);
switch (true) {
case $score_percent >= 90:
$letter_grade = 'A';
break;
case $score_percent >= 80:
$letter_grade = 'B';
break;
case $score_percent >= 70:
$letter_grade = 'C';
break;
case $score_percent >= 60:
$letter_grade = 'D';
break;
default:
$letter_grade = 'F';
}
} // provide feedback on submission
?>
<h1>Great Explorers Quiz</h1>
<p>Answer all of the questions on the quiz, then select the Score button to grade the quiz.</p>
<?php
//Display this quiz FORM only if they have not submitted answers
echo '
<form action="explorequiz.php" method="post">
<p><b>1. In what year did Sir Edmun Hillary and Tenzing Norgay become the first climbers to reach the summit of Mt. Everest?</b></p>
<p><input type="radio" name="question1" value="a" /> 1927<br />
<input type="radio" name="question1" value="b" /> 1936<br />
<input type="radio" name="question1" value="c" /> 1949<br />
<input type="radio" name="question1" value="d" /> 1953<br /> </p><!-- correct answer 5 points-->
<p><b>2. Which desert did David Livingston cross to reach Lake Ngami?</b></p>
<p><input type="radio" name="question2" value="a" /> Gobi<br />
<input type="radio" name="question2" value="b" /> Kalahari<br /> <!-- correct answer 5 points -->
<input type="radio" name="question2" value="c" /> Sahara<br />
<input type="radio" name="question2" value="d" /> Negev<br /></p>
<p><b>3. What African-American explorer reached the North Pole with Robert Peary in 1909?</b></p>
<p><input type="radio" name="question3" value="a" /> Matthew Henson<br />
<input type="radio" name="question3" value="b" /> Jim Beckwourth<br /> <!-- correct answer 8 points -->
<input type="radio" name="question3" value="c" /> Jesse Owens<br />
<input type="radio" name="question3" value="d" /> Booker T. Washington<br /> </p>
<p><b>4. What was the name of Jacques Cousteau\'s research vessel?</b></p>
<p><input type="radio" name="question4" value="a" /> Sea Witch<br />
<input type="radio" name="question4" value="b" /> Calypso<br /> <!-- correct answer 8 points -->
<input type="radio" name="question4" value="c" /> New Frontier<br />
<input type="radio" name="question4" value="d" /> Avignon<br /> </p>
<p><b>5. Who was the first European to explore Florida?</b></p>
<p><input type="radio" name="question5" value="a" /> Hernando De Soto<br />
<input type="radio" name="question5" value="b" /> Sir Francis Drake<br />
<input type="radio" name="question5" value="c" /> Ponce De Leon<br /><!-- correct answer 10 points -->
<input type="radio" name="question5" value="d" /> James Cook<br /> </p>
<p><input type = "submit" value = "Grade Quiz" name="grade"/></p>
</form>';
?>
</body>
</html>

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

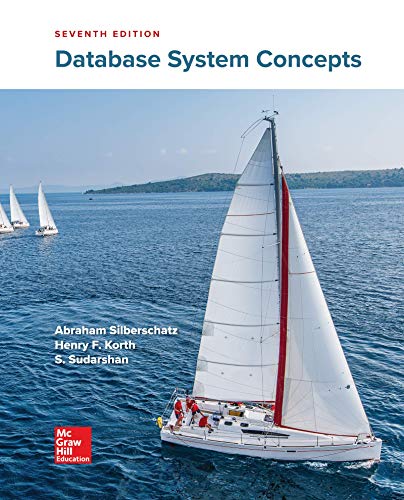
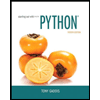
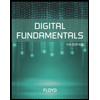
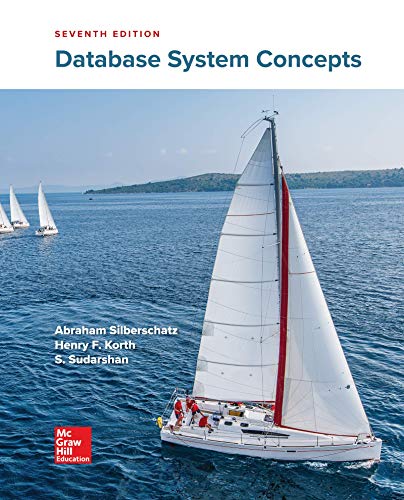
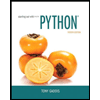
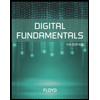
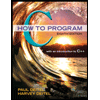
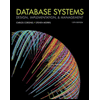
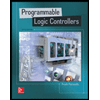